- Java String intern() method returns the canonical representation of the string.
- When we invoke intern() method on a string, if the string pool already has a string having the same value, then the reference of that string is returned. Otherwise, a new string with the same value is created in the pool and its reference is returned.
- Java String intern() is a native method.
- For two strings s1 and s2,
s1.intern() == s2.intern()
is true if and only ifs1.equals(s2)
is true. - If we call intern() method on a string literal, the same reference is returned because the string is already in the pool.
- The intern() method guarantees that the string returned is from the pool.
Table of Contents
Java String intern() Method Example
jshell> String s1 = "Hello";
s1 ==> "Hello"
jshell> String s2 = new String("Hello");
s2 ==> "Hello"
jshell> s1 == s2
$24 ==> false
jshell> String s3 = s2.intern();
s3 ==> "Hello"
jshell> s1 == s3
$26 ==> true
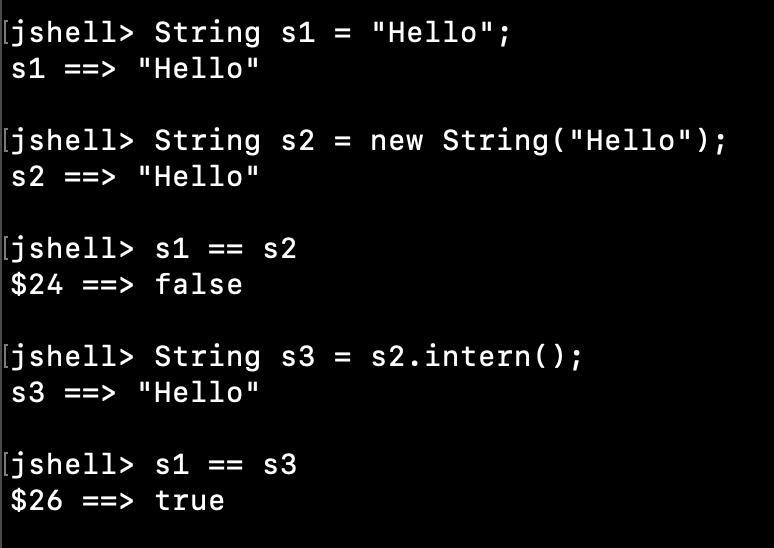
String intern() Example Explanation
Let’s understand what is happening in each of the above statements.
- String s1 is created in the string pool. It’s a string literal.
- String s2 is created in the heap space because we are using the new operator.
s1 == s2
returns false because they are referring to different objects.- When we are calling
s2.intern()
, a reference from the string pool is returned where the string value is “Hello”. Since string pool contains only unique values and we already have s1 with the same value, the same reference is returned. - We are assigning the interned string to a new string variable s3.
s1 == s3
returns true because both of them are referring to the same string object in the pool.
Why do we need the String intern() Method?
- If you have a lot of strings created using the new operator, it makes sense to call intern() method to save memory space.
- Generally, we use the equals() method for string equality. But, it can be slow if the string size is huge. We can call intern() method to make sure that the string is part of the pool and then use
==
operator for equality check. Since == operator only checks the reference, it’s much faster than the equals() method. - String intern() method is useful when we are getting a string object from other APIs. For example, StringBuilder and StringBuffer
toString()
method uses the new operator to create the string. If it’s huge and we want to test for equality, calling intern() and then using == operator would be better.