Java 7 started support for using String in switch case. In earlier versions, if you have to write conditional logic based on a string, you had to use if-else blocks.
Table of Contents
Java String Switch Case Example
Let’s look at some examples of using string in switch case statements.
package net.javastring.strings;
import java.util.Scanner;
public class JavaStringSwitchCase {
public static void main(String[] args) {
System.out.print("Please enter any programming language:\n");
Scanner scanner = new Scanner(System.in);
String input = scanner.next();
scanner.close();
switch (input) {
case "java":
System.out.println("Java Current Version is 12.");
break;
case "python":
System.out.println("Python Current Version is 3.7");
break;
case "rust":
System.out.println("Rust Current Version is 1.34.1");
break;
default:
System.out.println("We don't have information about this programming language");
}
}
}
Output:
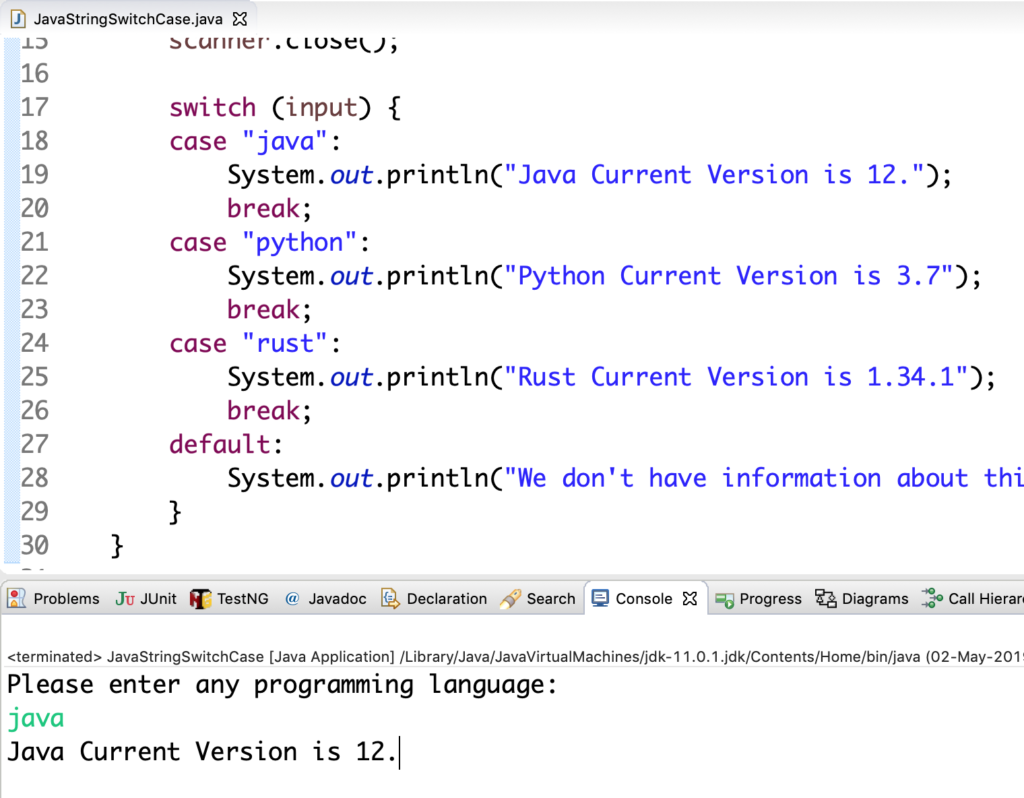
Java String Switch Case vs if-else
Let’s have a look at the alternate implementation of above code using if-else block.
if ("java".equals(input))
System.out.println("Java Current Version is 12.");
else if ("python".equals(input))
System.out.println("Python Current Version is 3.7");
else if ("rust".equals(input))
System.out.println("Rust Current Version is 1.34.1");
else
System.out.println("We don't have information about this programming language");
Important Points for String in Switch Case
- Java switch case with string is more readable than the multiple if-else if-else blocks.
- The switch case matching is case sensitive, so “java” will not match for input string “Java”.
- If the input string is null, switch-case will throw NullPointerException. So have a null check in place before writing the switch-case code.
- The bytecode generated by switch-case is more efficient than the if-else blocks. You can check this in the reference article below.
- You can convert the input string and all the case values to either uppercase or lowercase for case insensitive match.