Java String lastIndexOf() method is used to get the last index of a character or a substring in this string.
Table of Contents
String lastIndexOf() Methods
There are four variants of lastIndexOf() methods in the String class.
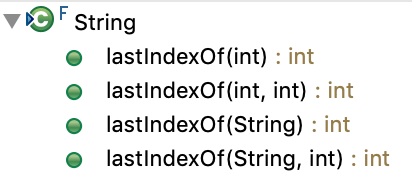
- lastIndexOf(int ch): returns the index of the last occurrence of the given character in this string. If the character is not present in the string, this method returns -1.
- lastIndexOf(int ch, int fromIndex): returns the index of the last occurrence of the given character, searching backward from the given index. If the fromIndex is negative, -1 is returned. If the fromIndex is greater than or equal to the length of the string, the entire string is searched.
- lastIndexOf(String str): returns the index of the last occurrence of the substring. The method returns -1 if the substring is not found.
- lastIndexOf(String str, int fromIndex): returns the index of the last occurrence of the substring, searching backward from the given index. The fromIndex is treated in the same way as in the above method.
The lastIndexOf() methods are very similar to the indexOf() methods, except that the element is searched backward to return the last index.
Java String lastIndexOf() Method Examples
Let’s look at some examples of lastIndexOf() method usage.
1. lastIndexOf(int ch)
jshell> String str = "012301230123";
str ==> "012301230123"
jshell> str.lastIndexOf('0')
$47 ==> 8
jshell> str.lastIndexOf(48)
$48 ==> 8
jshell> str.lastIndexOf('\u0030')
$49 ==> 8
jshell> str.lastIndexOf('a')
$50 ==> -1
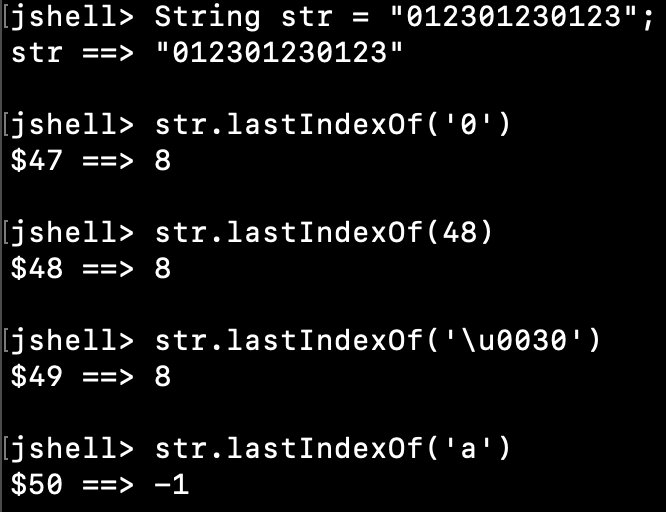
The Unicode code point of character ‘0’ is 48 and we can also write it in the unicode format as ‘\u0030’.
2. lastIndexOf(int ch, int fromIndex)
jshell> String str = "Hello World";
str ==> "Hello World"
jshell> str.lastIndexOf('l', 7)
$52 ==> 3
jshell> str.lastIndexOf('l', 50)
$53 ==> 9
jshell> str.lastIndexOf('l', -5)
$54 ==> -1
jshell> str.lastIndexOf('l', 1)
$55 ==> -1
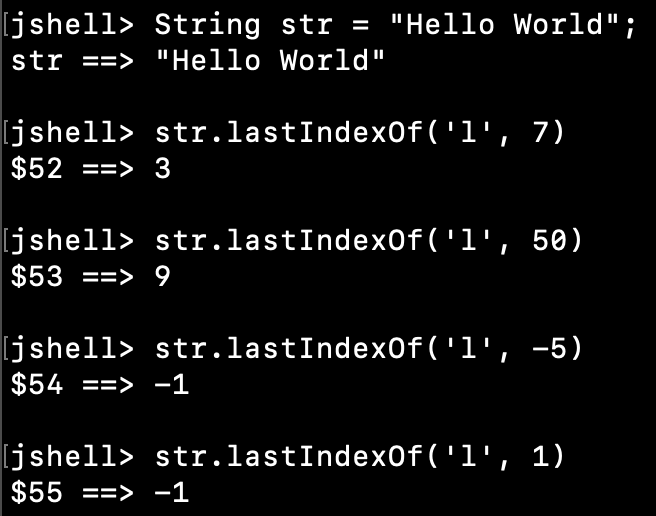
3. lastIndexOf(String str)
jshell> String str = "Hello Jello Trello";
str ==> "Hello Jello Trello"
jshell> str.lastIndexOf("llo")
$58 ==> 15
jshell> str.lastIndexOf("Jello")
$59 ==> 6
jshell> str.lastIndexOf("123")
$60 ==> -1
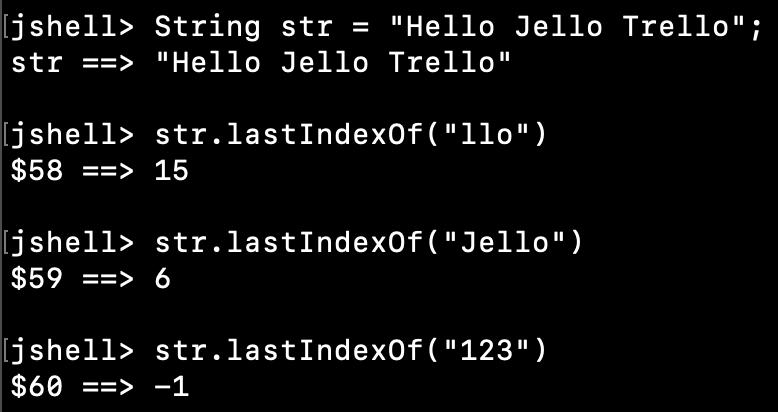
4. lastIndexOf(String str, int fromIndex)
jshell> String str = "Hello Jello Trello";
str ==> "Hello Jello Trello"
jshell> str.lastIndexOf("llo", 10)
$62 ==> 8
jshell> str.lastIndexOf("llo", -10)
$63 ==> -1
jshell> str.lastIndexOf("llo", 100)
$64 ==> 15
jshell> str.lastIndexOf("llo", 0)
$65 ==> -1