Java String Constructors allows us to create a string object from different type of arguments.
Table of Contents
Why do we need String Constructors?
We can create string object using double quotes.
String s1 = "Java String";
So why do we need so many constructors in String Class?
A string is a sequence of characters. Sometimes we want to create a string object from different sources. For example, byte array, character array, StringBuffer, and StringBuilder. The String class constructors are provided to create a string object from these arguments.
String Constructor Categories
We can broadly classify string constructors into following categories.
- Creating Empty String
- Creating String from another string
- String from Byte Array
- String from Character Array
- String from Code Points
- String from StringBuffer and StringBuilder
List of Java String Constructors
String()
: creates an empty string. It’s mostly useless because String is immutable.String(String original)
: creates a string object from another string. Since String is immutable, it’s of no use.String(byte[] bytes)
: constructs a new string from the byte array using system default encoding.String(byte bytes[], String charsetName)
: uses the specified character encoding. If the encoding is not supported,UnsupportedEncodingException
is thrown.String(byte bytes[], Charset charset)
: a better way to specify an encoding for constructing the string object.String(byte bytes[], int offset, int length)
: Theoffset
specifies the index of the first byte to decode. Thelength
specifies the number of bytes to decode. This constructor throwsIndexOutOfBoundsException
ifoffset
is negative,length
is negative, oroffset
is greater thanbytes.length - length
.String(byte bytes[], int offset, int length, Charset charset)
: It’s similar to the above constructor except that we have to specify the encoding to use.String(byte bytes[], int offset, int length, String charsetName)
: Similar to above except that the character set encoding name is passed as a string. This will throwUnsupportedEncodingException
if the encoding is not supported.String(char value[])
: creates the string object from the character array.String(char value[], int offset, int count)
: Theoffset
specifies the index of the first character. Thelength
specifies the number of characters to use. This constructor throwsIndexOutOfBoundsException
ifoffset
is negative,length
is negative, oroffset
is greater thanvalue.length - length
.String(int[] codePoints, int offset, int count)
: creates a string from the input Unicode code points array. It throwsIllegalArgumentException
if any of the code points are invalid. This constructor throwsIndexOutOfBoundsException
if theoffset
is negative, thelength
is negative, oroffset
is greater thancodePoints.length - length
.String(StringBuffer buffer)
: creates a new string from the contents of the string buffer. This constructor internally calls StringBuffer toString() method.String(StringBuilder buffer)
: creates a new string from the contents of the string builder.
String Constructor Examples
Let’s look at some code snippets to use the string constructors.
1. Creating Empty String
String s2 = new String(); // useless though
s2 = ""; // better approach
2. Creating String from Another String
String s5 = new String("2019"); // useless since string is immutable
3. Java String Constructor with Byte Array Argument
byte[] bytes = "Java".getBytes();
System.out.println(Arrays.toString(bytes));
String s3 = new String(bytes);
System.out.println(s3);
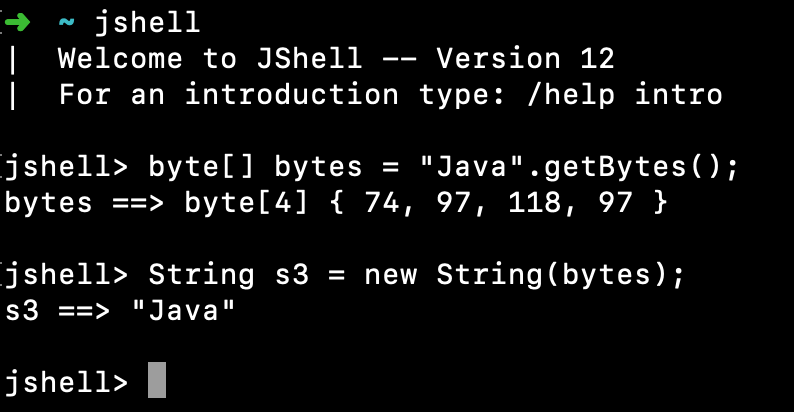
4. Creating String from Byte Array with Encoding
byte[] bytes = "Java".getBytes();
String s3 = null;
try {
s3 = new String(bytes, "UTF-8");
} catch (UnsupportedEncodingException e1) {
e1.printStackTrace();
}
System.out.println(s3);
s3 = new String(bytes, StandardCharsets.UTF_8);
System.out.println(s3);
Output:
Java
Java
5. Creating String from Byte Array with Index
byte[] bytes = "Java".getBytes();
String s3 = new String(bytes, 1, 2);
System.out.println(s3);
s3 = new String(bytes, 1, 3, StandardCharsets.US_ASCII);
System.out.println(s3);
try {
s3 = new String(bytes, 1, 3, "UTF-8");
} catch (UnsupportedEncodingException e) {
e.printStackTrace();
}
System.out.println(s3);
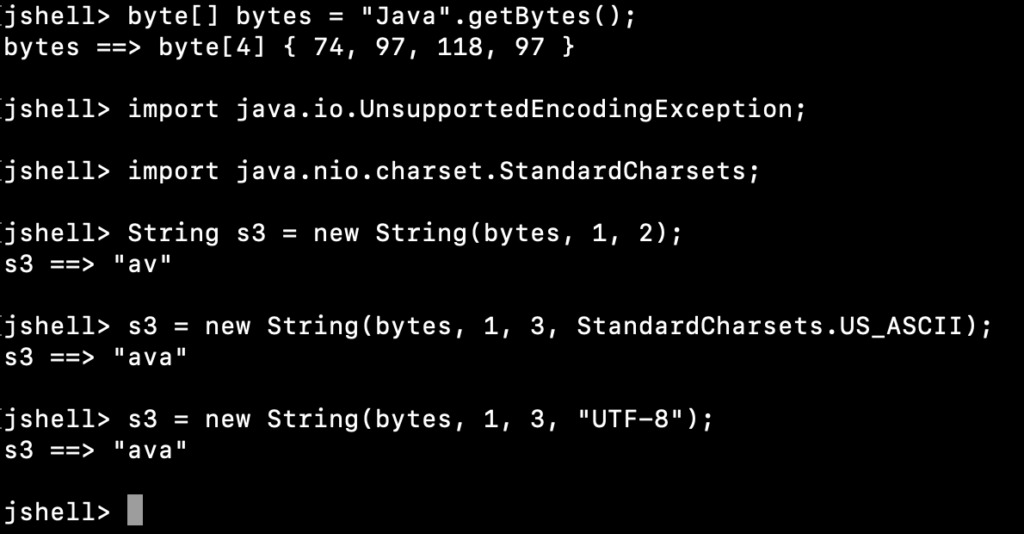
6. String Constructor with Character Array Argument
char[] chars = "String Constructors".toCharArray();
System.out.println(Arrays.toString(chars));
String s4 = new String(chars);
System.out.println(s4);
s4 = new String(chars, 2, 8);
System.out.println(s4);
Output:
[S, t, r, i, n, g, , C, o, n, s, t, r, u, c, t, o, r, s]
String Constructors
ring Con
7. String Constructor with Code Points Array
int[] codePoints = { 74, 97, 118, 97 };
String s6 = new String(codePoints, 0, 2);
System.out.println(s6);
s6 = new String(codePoints, 0, codePoints.length);
System.out.println(s6);
Output:
Ja
Java
8. String Constructor with StringBuffer Argument
StringBuffer sb = new StringBuffer("Hello");
sb.append("World");
String s7 = new String(sb);
System.out.println(s7);
s7 = sb.toString(); // better approach
System.out.println(s7);
9. String Constructor with StringBuilder Argument
StringBuilder sb1 = new StringBuilder("Java ");
sb1.append("String").append(" Class");
String s8 = new String(sb1);
System.out.println(s8);
s8 = sb1.toString(); // better approach
System.out.println(s8);
Conclusion
Java String provides a lot of constructors for general purpose requirements. If you are creating a new string, try to use a string literal. You can use the constructors to create the string from a byte array, char array, and code points. Always use StringBuffer and StringBuilder toString() method to create their string representation.