Java String length() method returns the length of this string. The output is the number of Unicode code units in the string.
Table of Contents
Java String length() Method Example
Let’s look at a simple program for finding the length of a string in Java.
package net.javastring.strings;
public class JavaStringLength {
public static void main(String args[]) {
String s1 = "Hello";
System.out.println(s1.length());
}
}
Output:
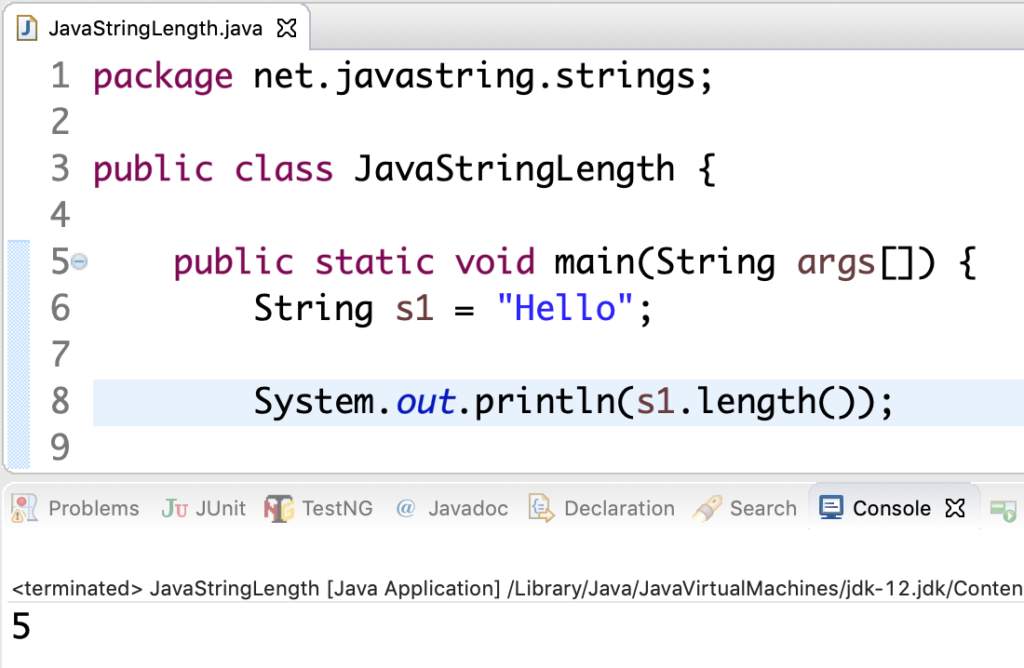
What happens when String has Unicode characters?
We can create a string object with Unicode value too. Let’s look at an example to find the length of a string having Unicode value. We will use JShell for this example.
jshell> String s2 = "\u00A9";
s2 ==> "©"
jshell> s2.length();
$11 ==> 1
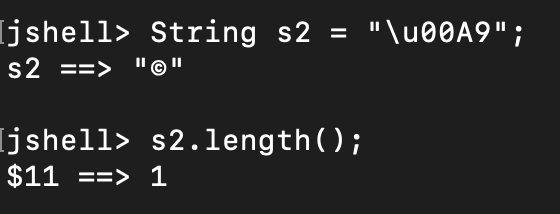
Alternative Ways to Find the Length of String
This is an interesting interview question. You will have to write a program to find the length of the String without using the length() method. Let’s look at two alternative ways to find the length of the string.
1. Using Character Array
We can convert the string to the character array. Then use its length attribute to find the length of the string.
String s3 = "Welcome To \u00A9 JavaString.net";
char [] ca = s3.toCharArray();
System.out.println(ca.length); // 27
2. Using String lastIndexOf() method
We can use lastIndexOf() method cleverly to get the length of the string.
String s4 = "Hi\nHowdy \u00A9 Java ";
int length = s4.lastIndexOf("");
System.out.println(length); // 16
Let’s look at the output in JShell. The newline character length is also considered as 1.
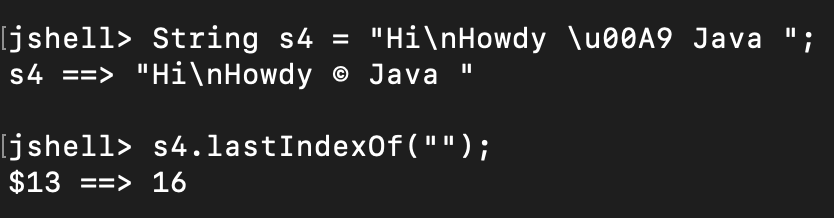
Conclusion
Java String length() method returns the length of the string. The newline character and tab character length is 1. If the string has Unicode value, they are considered as a single character.
We looked into some alternate ways to find the length of the string. But, always use the length() method to get the length of the string.