Java String startsWith() method is used to test if the string starts with the given prefix. This method is case sensitive.
Table of Contents
String startsWith() Methods
There are two variants of startsWith() method.
- startsWith(String prefix): used to determine if the string starts with the specified prefix or not. If the prefix is empty string then this method returns true. If the prefix is null, the method throws NullPointerException.
- startsWith(String prefix, int toffset): used to test if the substring of this string beginning at the given index starts with the given prefix.
String startsWith() Method Examples
Let’s look at some examples of string startsWith() method.
1. Checking if the string starts with the given prefix
jshell> String s1 = "Hello World";
s1 ==> "Hello World"
jshell> s1.startsWith("Hello")
$38 ==> true
jshell> s1.startsWith("Hi");
$39 ==> false
jshell> s1.startsWith("hello");
$40 ==> false
Output:
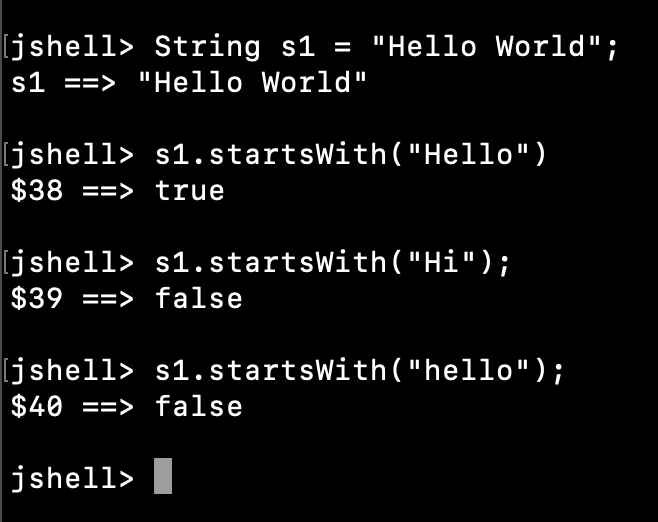
2. Checking if this string contains a substring at the given index
jshell> String s1 = "Hello World";
s1 ==> "Hello World"
jshell> s1.startsWith("Wor", 6)
$41 ==> true
jshell> s1.startsWith("Wor", 5)
$42 ==> false
3. Getting NullPointerException when string argument is null
Let’s see what happens when we pass string argument as null in the startsWith() method.
jshell> s1.startsWith(null)
| Exception java.lang.NullPointerException
| at String.startsWith (String.java:1437)
| at String.startsWith (String.java:1479)
| at (#46:1)
jshell> s1.startsWith(null, 1)
| Exception java.lang.NullPointerException
| at String.startsWith (String.java:1437)
| at (#47:1)
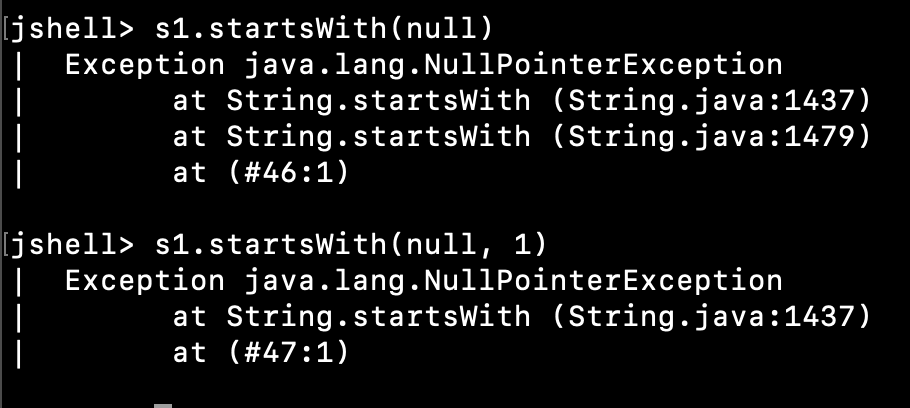
4. Java String startsWith() Method with invalid index
If the index argument in the startsWith() method is negative or greater than the length, the method returns false
. It doesn’t throw any exceptions.
jshell> s1.startsWith("Wor", 500)
$48 ==> false
jshell> s1.startsWith("Wor", -500)
$49 ==> false
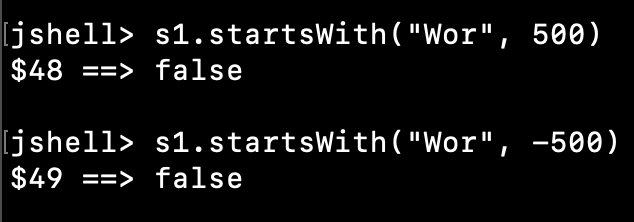
5. Using startsWith() Method to check if the string contains a substring
The String contains() method can be used to check if the string contains a substring. Just for the sake of an example, we can implement a method to check if the string contains a substring using startsWith() method.
package net.javastring.strings;
public class JavaStringStartsWith {
public static void main(String[] args) {
String s1 = "Hello World";
System.out.println(containsSubstring(s1, "ll"));
System.out.println(containsSubstring(s1, "ld"));
System.out.println(containsSubstring(s1, "Hi"));
}
private static boolean containsSubstring(String s1, String prefix) {
boolean result = false;
for (int i = 0; i < s1.length() - prefix.length() + 1; i++) {
result = s1.startsWith(prefix, i);
if (result) {
return result;
}
}
return result;
}
}
Output:
true
true
false
Conclusion
Java String startsWith() is a simple utility method to test if this string starts with the given prefix or not. This can be used to check if the string has the given character sequence at a specific index or not.