Sometimes we have to generate random string in Java. Usually, a random string is used to create a unique identifier for session, database table primary key, etc.
Table of Contents
Algorithm to Generate Random String in Java
- Create an alphanumeric string that contains all the ASCII uppercase and lowercase characters and digits.
- Use the Random class to generate a random number between 0 and the length of the alphanumeric string.
- Add the character at the random index of the alphanumeric string to a StringBuilder object.
- Repeat steps 2 and 3 until the StringBuilder size is equal to the required length of the random string.
- Return the random string using the StringBuilder toString() method.
Java Program to Generate Random String of Given Length
Here is the utility method implementation to generate a random string based on the above algorithm. We are getting the random string length from the user input.
package net.javastring.strings;
import java.util.Random;
import java.util.Scanner;
public class GenerateRandomString {
public static void main(String[] args) {
String asciiUpperCase = "ABCDEFGHIJKLMNOPQRSTUVWXYZ";
String asciiLowerCase = asciiUpperCase.toLowerCase();
String digits = "1234567890";
String asciiChars = asciiUpperCase + asciiLowerCase + digits;
Scanner sc = new Scanner(System.in);
System.out.println("Please enter random string length");
int length = sc.nextInt();
sc.close();
String randomString = generateRandomString(length, asciiChars);
System.out.println(String.format("The random string is %s", randomString));
}
private static String generateRandomString(int length, String seedChars) {
StringBuilder sb = new StringBuilder();
int i = 0;
Random rand = new Random();
while (i < length) {
sb.append(seedChars.charAt(rand.nextInt(seedChars.length())));
i++;
}
return sb.toString();
}
}
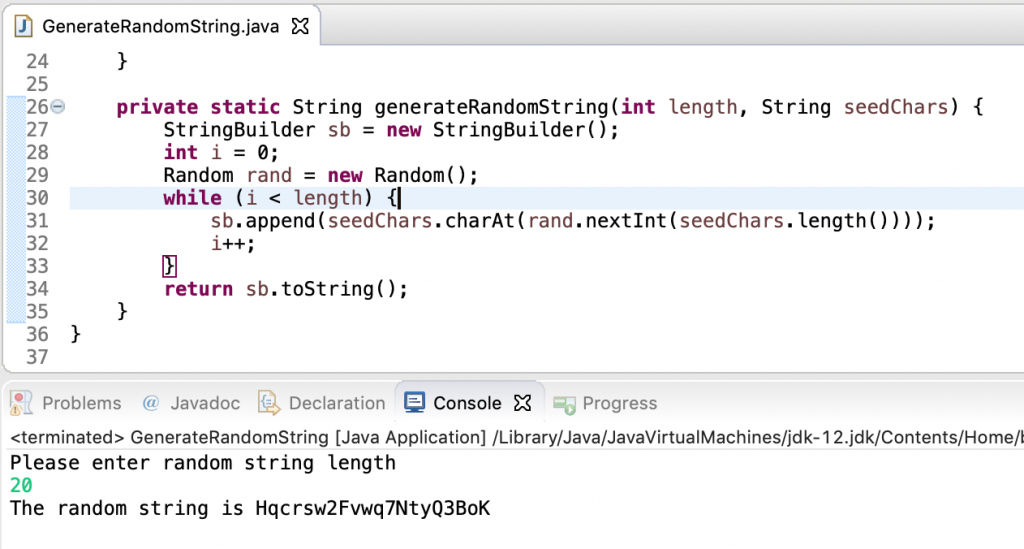
Important Points
- If you want more security in generating a random index, use SecureRandom class.
- We can also add some special characters in the seed string to generate a strong random string. This is required if you are generating a random password string in your program.
- We can easily modify the generateRandomString() method to add more complexity in generating the random string.