- Java String matches(regex) method is used to test if the string matches the given regular expression or not.
- String matches() method internally calls Pattern.matches() method.
- This method returns a boolean value. If the regex matches the string, it returns “true”, otherwise “false”.
- If the regex pattern is invalid,
PatternSyntaxException
is thrown.
Table of Contents
Java String matches() Method Implementation
If you look at the source code of the String class, matches() method is implemented like this.
public boolean matches(String regex) {
return Pattern.matches(regex, this);
}
It’s very similar to the String replaceAll() method.
Java String matches(regex) Examples
Let’s look at some examples of matches() method.
1. Matching String for Two Words of 5 characters
jshell> String str = "Hello World";
str ==> "Hello World"
jshell> str.matches("\\w{5} \\w{5}");
$13 ==> true
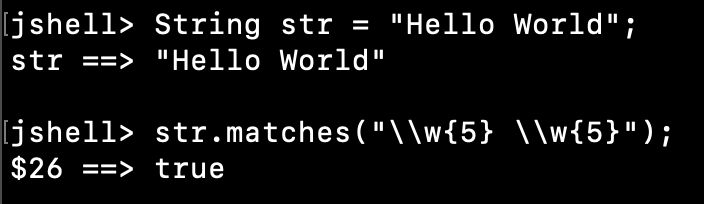
The pattern “\w{5}” will match a word having the length as 5.
2. Matching a Positive Integer of any length
We can use “\d+” to match a string having the positive integer data of any length.
jshell> "1245".matches("\\d+");
$14 ==> true
jshell> "0".matches("\\d+");
$15 ==> true
jshell> "-10".matches("\\d+");
$16 ==> false
jshell> "10.5".matches("\\d+");
$17 ==> false
3. Matching Special Characters
We can use “\W+” to test if a string is made up of only special characters.
jshell> "$%#".matches("\\W+");
$18 ==> true
jshell> "$%#A".matches("\\W+");
$19 ==> false
jshell> "".matches("\\W+");
$20 ==> false
jshell> "_@_".matches("\\W+");
$21 ==> false
jshell> "@".matches("\\W+");
$22 ==> true
Note that the underscore is not considered as a special character. It’s treated as a word character in Java.
4. Case Insensitive Matching
String matches() perform case sensitive matching. If you want case insensitive matching, there are two options.
- Use Pattern class directly and compile it with Pattern.CASE_INSENSITIVE flag.
- Use (?i) for the whole regex string for the case insensitive comparison.
jshell> "a".matches("A");
$23 ==> false
jshell> "a".matches("(?i)A");
$24 ==> true
Conclusion
Java String matches(regex) is a utility method to quickly test if the string matches a regex or not. If you want to perform more complex operations, it’s better to use Pattern class.