- Java String subSequence() method returns a character subsequence from this string.
- This method is added to String class so that it can implement CharSequence interface.
- The subSequence() method internally calls substring() method.
- We can explicitly cast the returned CharSequence to a String object.
- It throws StringIndexOutOfBoundsException if the index values are invalid.
Table of Contents
String subSequence() Method Implementation
If you look at the source code of the String class, subSequence() method calls substring() method.
public CharSequence subSequence(int beginIndex, int endIndex) {
return this.substring(beginIndex, endIndex);
}

Java String subSequence() Example
Let’s look at a simple example of subSequence() method.
jshell> String str = "JavaString.net";
str ==> "JavaString.net"
jshell> CharSequence cs = str.subSequence(4, 10);
cs ==> "String"
Here is an example to cast the returned CharSequence to a string object.
jshell> String s = (String) str.subSequence(4, 10);
s ==> "String"
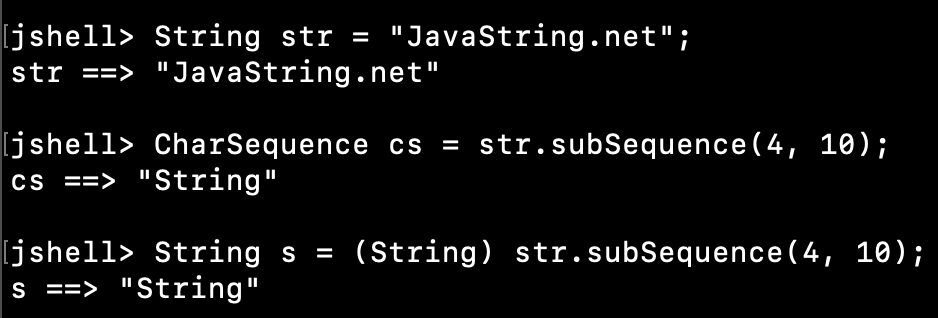
StringIndexOutOfBoundsException with subSequence()
The possible scenarios where we get StringIndexOutOfBoundsException exception are:
- The beginIndex or endIndex is negative.
- The endIndex is larger than the length of this string.
- The beginIndex is greater than the endIndex.
Let’s look at some examples of StringIndexOutOfBoundsException when calling subSequence() method.
jshell> "Hello".subSequence(-1, 2)
| Exception java.lang.StringIndexOutOfBoundsException: begin -1, end 2, length 5
| at String.checkBoundsBeginEnd (String.java:3410)
| at String.substring (String.java:1883)
| at String.subSequence (String.java:1922)
| at (#4:1)
jshell> "Hello".subSequence(1, -2)
| Exception java.lang.StringIndexOutOfBoundsException: begin 1, end -2, length 5
| at String.checkBoundsBeginEnd (String.java:3410)
| at String.substring (String.java:1883)
| at String.subSequence (String.java:1922)
| at (#5:1)
jshell> "Hello".subSequence(1, 200)
| Exception java.lang.StringIndexOutOfBoundsException: begin 1, end 200, length 5
| at String.checkBoundsBeginEnd (String.java:3410)
| at String.substring (String.java:1883)
| at String.subSequence (String.java:1922)
| at (#6:1)
jshell> "Hello".subSequence(3, 1)
| Exception java.lang.StringIndexOutOfBoundsException: begin 3, end 1, length 5
| at String.checkBoundsBeginEnd (String.java:3410)
| at String.substring (String.java:1883)
| at String.subSequence (String.java:1922)
| at (#7:1)
jshell>
Java String subSequence() vs substring()
- If you need a substring, use substring() method.
- You should use subSequence() if you want the substring in the form of CharSequence.
- The subSequence() method internally calls substring() method.
- String subSequence() method is added so that the String class can implement CharSequence method.
Conclusion
You should use substring() to create a substring from the string. The only possible use of subSequence() is when you need the substring in the form of the CharSequence object.