Java String endsWith() method is used to test if this string ends with the given suffix string or not. This method is case sensitive.
Table of Contents
Java String endsWith() Method Implementation
The String endsWith() method internally calls the startsWith() method. The method implementation from the Java Doc is:
public boolean endsWith(String suffix) {
return startsWith(suffix, length() - suffix.length());
}
String endsWith() Method Example
Here is a simple example of String endsWith() method.
package net.javastring.strings;
public class JavaStringEndsWith {
public static void main(String[] args) {
String message = "Hello World 2019";
System.out.println(message.endsWith("2019"));
System.out.println(message.endsWith("2018"));
}
}
Output:
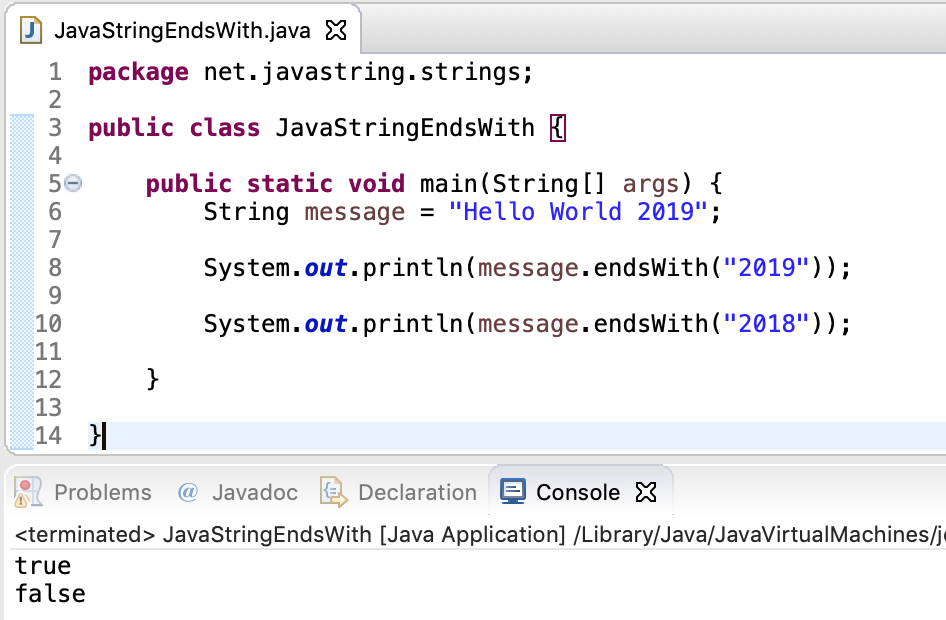
What if the suffix string is empty or null?
- If the suffix string is empty, the endsWith() method will return true.
- If the suffix string is null, the endsWith() method will throw NullPointerException.
jshell> String msg = "Hi";
msg ==> "Hi"
jshell> msg.endsWith("");
$51 ==> true
jshell> msg.endsWith(null);
| Exception java.lang.NullPointerException
| at String.endsWith (String.java:1494)
| at (#52:1)
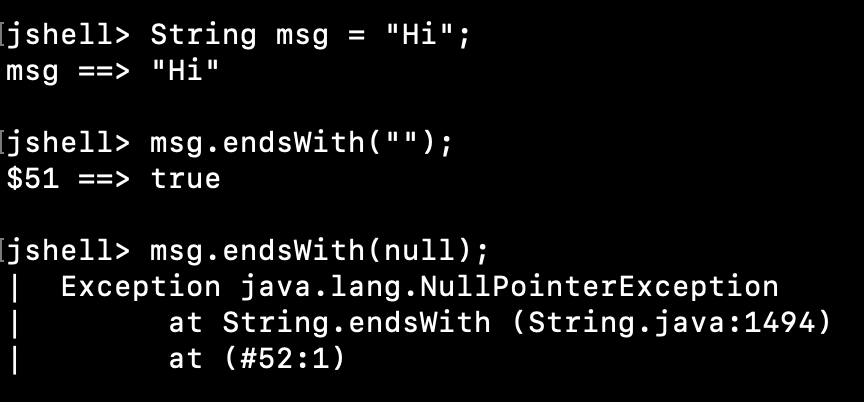