- Java StringJoiner is a utility class to create a sequence of characters separated by a delimiter.
- When creating StringJoiner instance, we can also specify the prefix and suffix character sequence.
- If StringJoiner doesn’t have any sequence added, its toString() method will return “prefix+suffix” string.
- We can call setEmptyValue() method to set the output of the toString() method when we haven’t added any elements to the StringJoiner.
- We can use StringJoiner merge() method to add the elements from the other StringJoiner instance to this instance. The prefix and suffix of the given StringJoiner are not merged. The delimiter of the given StringJoiner is used to create the character sequence before merging to this StringJoiner.
- StringJoiner length() method returns the length of the
String
representation of thisStringJoiner
. - StringJoiner class was added in Java 1.8 release.
Table of Contents
What is the benefit of Java StringJoiner class?
StringJoiner class provides a standard way to create a character sequence by merging other character sequences with a delimiter. The popular use case is to create a CSV string from a string array or list of strings.
StringJoiner Class Constructors
- StringJoiner(CharSequence delimiter): creates the instance with the given delimiter and no prefix suffix character sequence. If the delimiter is null, NullPointerException is thrown.
- StringJoiner(CharSequence delimiter, CharSequence prefix, CharSequence suffix): creates the instance with the given delimiter, prefix, and suffix character sequence. This will throw NullPointerException if any of the argument is null.
StringJoiner Class Methods
- add(CharSequence newElement): adds the given CharSequence element to the StringJoiner. If the argument is null, then “null” string is added.
- setEmptyValue(CharSequence emptyValue): sets the output of the toString() method when the StringJoiner is empty i.e. no character sequences is added to the StringJoiner instance.
- length(): returns the length of the string representation of the StringJoiner.
- merge(StringJoiner other): merges the elements of the given StringJoiner to this instance without prefix and suffix value. If the given StringJoiner is using a different delimiter, then elements of the other StringJoiner are concatenated with that delimiter and the result is appended to this StringJoiner instance.
- toString(): returns the string representation of the StringJoiner. If no element has been added, then “prefix+suffix” or the “emptyValue” is returned. If elements are added, then “prefix+{elements_with_delimiter}+suffix” string is returned.
Java StringJoiner Examples
Let’s look at some examples of StringJoiner class and its methods.
1. Creating a CSV string from elements of String Array
This is a very popular use case for StringJoiner class. Earlier, we had to write our own code to create a CSV string from a string array.
package net.javastring.strings;
import java.util.StringJoiner;
public class JavaStringJoiner {
public static void main(String[] args) {
String[] strArray = { "A", "B", "C" };
StringJoiner sj = new StringJoiner(",");
for (String s : strArray) {
sj.add(s);
}
System.out.println(sj);
}
}
Output: A,B,C
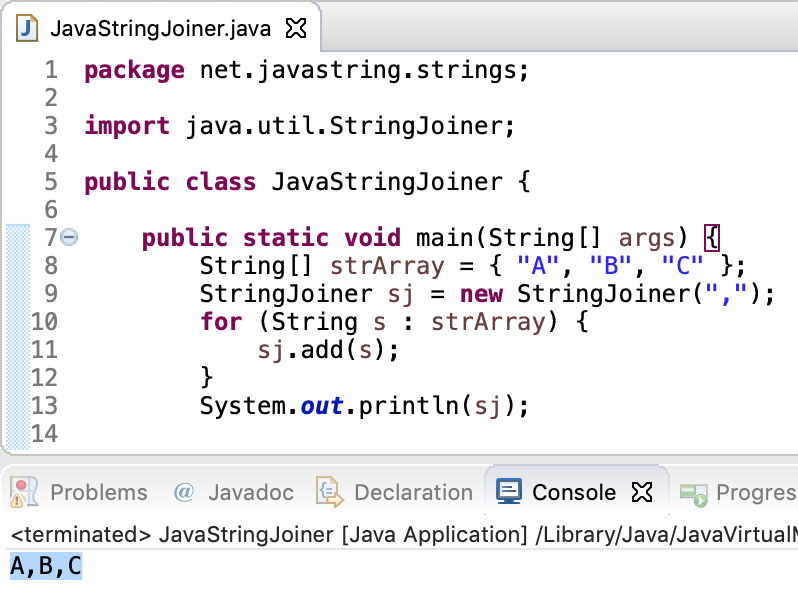
2. StringJoiner Prefix Suffix Example
jshell> StringJoiner sj = new StringJoiner(",", "{", "}");
sj ==> {}
jshell> sj.add("USA");
$102 ==> {USA}
jshell> sj.add("India");
$103 ==> {USA,India}
jshell> sj.add("UK");
$104 ==> {USA,India,UK}
jshell> System.out.println(sj.toString());
{USA,India,UK}
3. StringJoiner length() Example
jshell> StringJoiner sj = new StringJoiner(",", "{", "}");
sj ==> {}
jshell> sj.length();
$107 ==> 2
jshell> sj.add("India");
$108 ==> {India}
jshell> sj.length();
$109 ==> 7
4. Merging Two StringJoiner Elements
package net.javastring.strings;
import java.util.StringJoiner;
public class JavaStringJoiner {
public static void main(String[] args) {
StringJoiner sj = new StringJoiner(",", "{", "}");
sj.add("USA");
sj.add("India");
sj.add("UK");
System.out.println(sj);
StringJoiner sj1 = new StringJoiner("|", "#", "#");
sj1.add("France");
sj1.add("Germany");
sj.merge(sj1);
System.out.println(sj);
}
}
Output:
{USA,India,UK}
{USA,India,UK,France|Germany}
5. StringJoiner setEmptyValue() Example
package net.javastring.strings;
import java.util.StringJoiner;
public class JavaStringJoiner {
public static void main(String[] args) {
StringJoiner sj2 = new StringJoiner(",", "{", "}");
System.out.println(sj2);
sj2.setEmptyValue("[]");
System.out.println(sj2);
sj2.add("Apple");
System.out.println(sj2);
}
}
Output:
{}
[]
{Apple}
Notice that the toString() output changes when the StringJoiner is empty and we set its empty value. Once we have added an element to it, the empty value has no meaning.
6. StringJoiner with StringBuffer and StringBuilder
StringJoiner works with CharSequence objects. So we can also use it to create a character sequence from StringBuffer and StringBuilder objects.
StringJoiner sj2 = new StringJoiner(",", "{", "}");
sj2.add("Apple");
sj2.add(new StringBuffer("Banana"));
sj2.add(new StringBuilder("Mango"));
System.out.println(sj2);
Output: {Apple,Banana,Mango}
Conclusion
StringJoiner is a utility class to concatenate multiple character sequences with a delimiter to create a new character sequence. This is a very trivial operation and it’s good to have a standard way to do this rather than writing our own logic for it.