Java String to double conversion can be done in many ways. The double is a primitive data type. Double is the corresponding wrapper class. Java supports autoboxing. So we can use double primitive value and Double object interchangeably. The Double class provides different methods to convert string to double in Java.
Table of Contents
Different Ways to Convert String to Double
- Double.parseDouble()
- Double.valueOf()
- new Double(String s)
- DecimalFormat.parse()
1. Double.parseDouble()
We can use Double.parseDouble() to convert String object to double. The string can start with “+” or “-” to declare positive and negative double values. The string can end with “d” to denote that it contains a double value.
If the string is null, then this method throws NullPointerException
. If the string is not parsable, then NumberFormatException
is thrown.
If there are trailing 0s, then they are removed from the output double value.
Let’s look at some examples to convert String to double using Double.parseDouble() method.
// positive double value
double d1 = Double.parseDouble("+10.234");
System.out.println(d1);
// negative double value
double d2 = Double.parseDouble("-23.23");
System.out.println(d2);
// double value ending with 0s and 'd'
double d3 = Double.parseDouble("12.3400d");
System.out.println(d3);
Output:
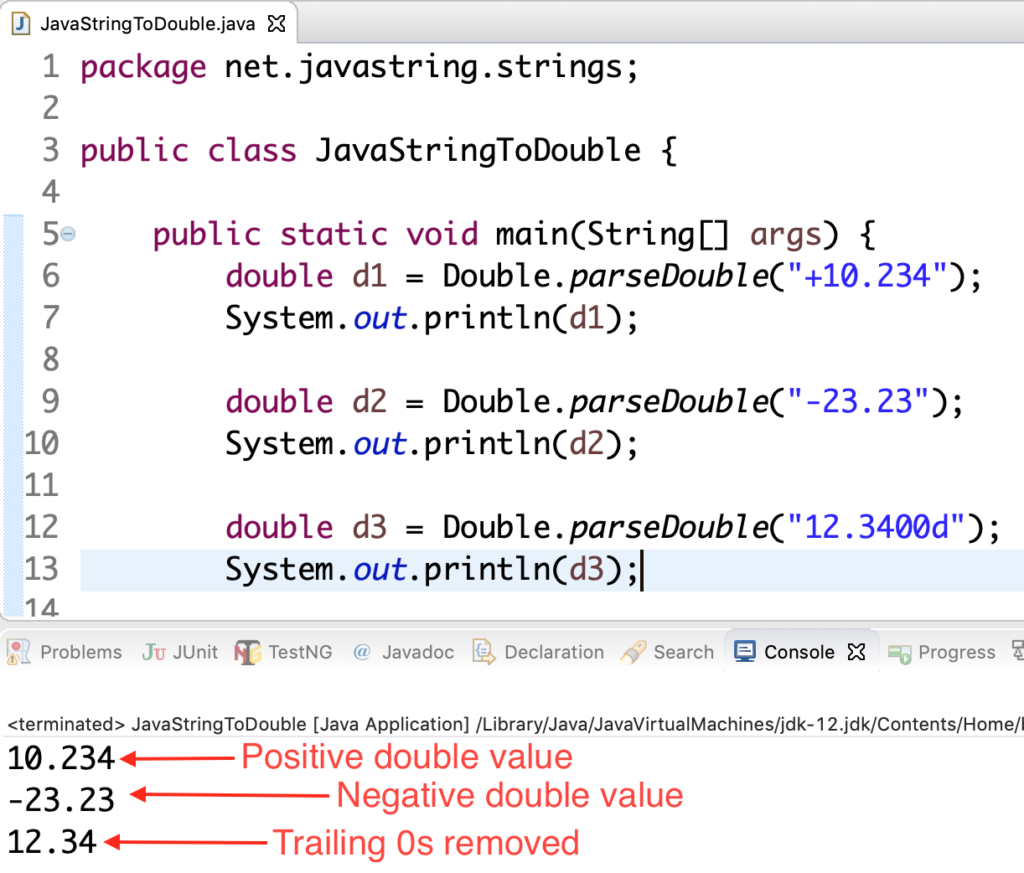
2. Double.valueOf()
This method returns the Double object. It’s very similar to the parseDouble() method. Let’s look at some examples to convert string to Double object using valueOf() method. This time we will use JShell to run our code snippets.
jshell> Double d4 = Double.valueOf("+10.234");
d4 ==> 10.234
jshell> Double d5 = Double.valueOf("-23.23");
d5 ==> -23.23
jshell> Double d6 = Double.valueOf("12.3400d");
d6 ==> 12.34
Output:
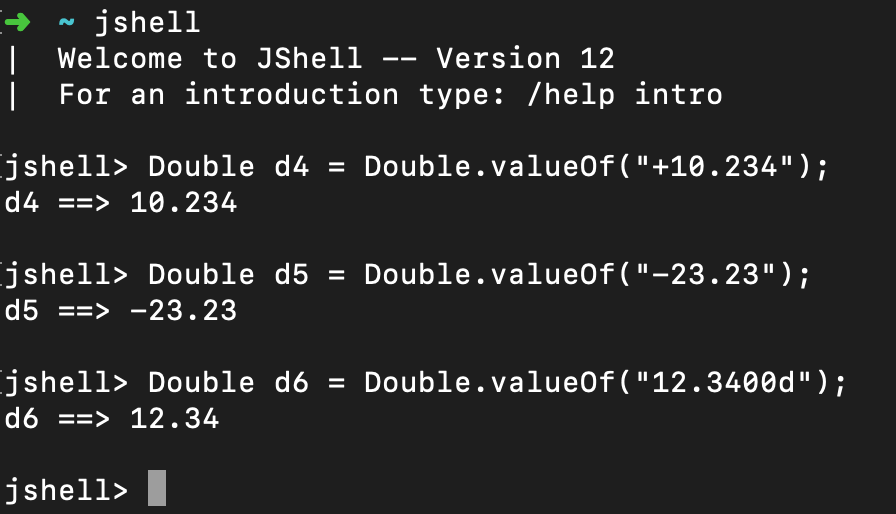
3. new Double(String s)
This Double class constructor accepts string argument and converts to the Double object. This constructor has been deprecated in Java 9. Java suggests to use parseDouble() or valueOf() methods to convert String to double. If the string is not parsable, then NumberFormatException is thrown. This constructor works in the same way as the valueOf() method.
jshell> Double d7 = new Double("+10.234");
d7 ==> 10.234
jshell> Double d8 = new Double("-23.23");
d8 ==> -23.23
jshell> Double d9 = new Double("12.3400d");
d9 ==> 12.34
4. DecimalFormat.parse()
DecimalFormat is a very useful class. We can use its parse() method to convert a formatted string to double value. We can parse percentage values, currency values, scientific values. DecimalFormat provides different methods to get a specific type of formatter. Some of these formatters support Locale too.
DecimalFormat parse()
method throws ParseException
when the string is not parsable.
Let’s look at some examples to convert formatted string to double value.
jshell> import java.text.DecimalFormat;
jshell> double d10 = DecimalFormat.getNumberInstance().parse("1,23.45d").doubleValue();
d10 ==> 123.45
jshell> double d11 = DecimalFormat.getCurrencyInstance(Locale.US).parse("$12,345.68").doubleValue();
d11 ==> 12345.68
jshell>
Output:

If you are using Eclipse, you will have to catch the ParseException or throw it to the caller.
try {
double d10 = DecimalFormat.getNumberInstance().parse("1,23.45d").doubleValue();
System.out.println(d10);
double d11 = DecimalFormat.getCurrencyInstance(Locale.US).parse("$12,345.68").doubleValue();
System.out.println(d11);
} catch (ParseException e) {
e.printStackTrace();
}
Conclusion
We looked at different ways to convert String to double in Java. When you want double primitive type, use Double.parseDouble() method. When you want Double object, use Double.valueOf() method. If the string is formatted, DecimalFormat provides a lot of useful formatters to help you out.