Java String indexOf() method is used to get the first index of a character or a substring in this string.
Table of Contents
String indexOf() Methods
There are four overloaded indexOf() methods in String class.
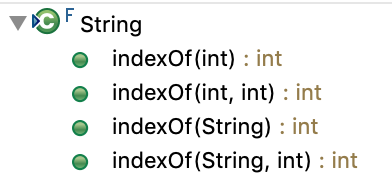
- indexOf(int ch): returns the index of the first occurrence of the given character. If the character is not found, then this method returns -1.
- indexOf(int ch, int fromIndex): returns the index of the first occurrence of the given character, starting the search from the given index. The method returns -1 if the given character is not found. If the fromIndex is negative, it’s considered as 0. If the fromIndex value is greater than the length of the string, -1 is returned.
- indexOf(String str): returns the index of the first occurrence of the given substring. If the substring is not found, then -1 is returned.
- indexOf(String str, int fromIndex): returns the index of the first occurrence of the given substring, starting from the given index. The method returns -1 if the substring is not found or the fromIndex is greater than the length of the string. If the fromIndex is negative, it’s considered as 0.
Java String indexOf() Method Examples
Let’s look at some simple examples of all the indexOf() methods.
1. indexOf(int ch)
jshell> String str = "Hello World";
str ==> "Hello World"
jshell> str.indexOf('o')
$18 ==> 4
jshell> str.indexOf('O')
$20 ==> -1
jshell> str.indexOf(111)
$24 ==> 4
jshell> str.indexOf('\u006F')
$25 ==> 4
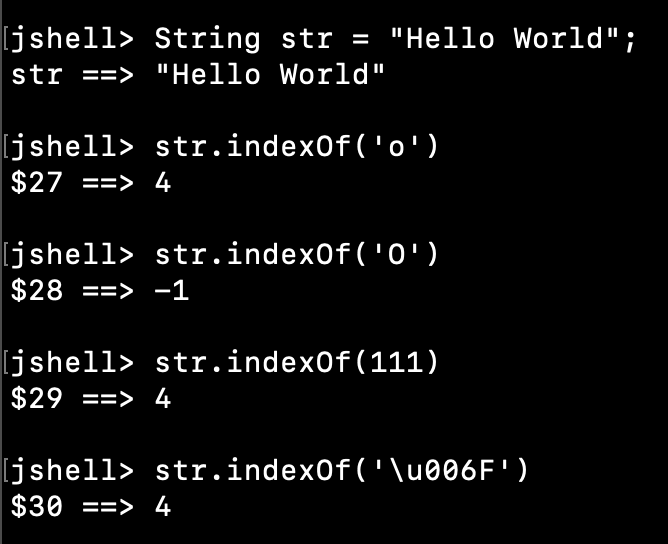
The code point value of character ‘o’ is 111 and it can also be written in Unicode as ‘\u006F’.
2. indexOf(int ch, int fromIndex)
jshell> String str = "Hello World";
str ==> "Hello World"
jshell> str.indexOf('o', 5)
$32 ==> 7
jshell> str.indexOf('o', 8)
$33 ==> -1
jshell> str.indexOf('o', -5)
$34 ==> 4
jshell> str.indexOf('o', 50)
$35 ==> -1
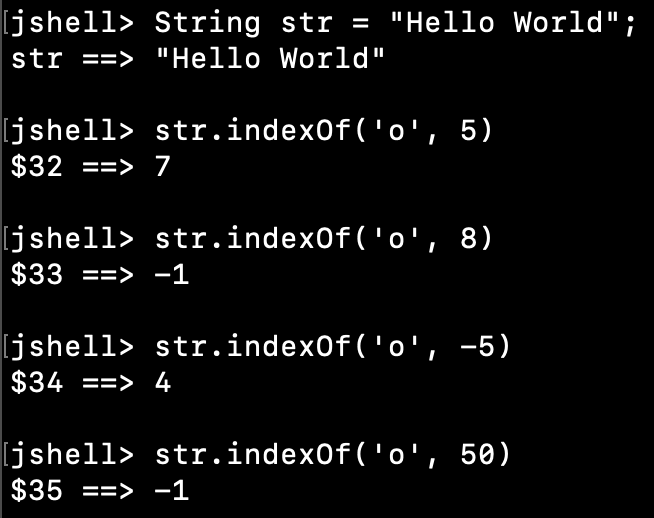
3. indexOf(String str)
jshell> String str = "Hello JavaString";
str ==> "Hello JavaString"
jshell> str.indexOf("Hell")
$37 ==> 0
jshell> str.indexOf("Hello")
$38 ==> 0
jshell> str.indexOf("Java")
$39 ==> 6
jshell> str.indexOf("123")
$40 ==> -1
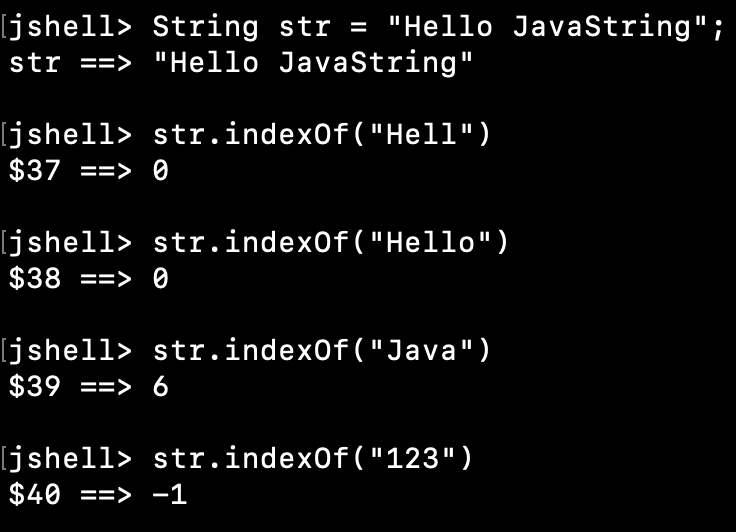
4. indexOf(String str, int fromIndex)
jshell> String str = "Hello Jello";
str ==> "Hello Jello"
jshell> str.indexOf("ll", 5)
$42 ==> 8
jshell> str.indexOf("ll", -5)
$43 ==> 2
jshell> str.indexOf("ll", 50)
$44 ==> -1
jshell> str.indexOf("xyz", 0)
$45 ==> -1
Getting All the indexes of a Substring
There is no method defined to get all the indexes of the occurrence of a substring. However, we can easily write the code to get all the indexes of the occurrences of a substring.
package net.javastring.strings;
import java.util.ArrayList;
import java.util.List;
import java.util.Scanner;
public class JavaStringIndexOf {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("Please enter a line:");
String input = scanner.nextLine();
System.out.println("Please enter a substring:");
String substring = scanner.nextLine();
scanner.close();
int index = 0;
List<Integer> indexes = new ArrayList<>();
while (index < input.length()) {
int i = input.indexOf(substring, index);
if (i == -1)
break;
indexes.add(i);
index = i + 1;
}
System.out.println("The substring found at indexes: " + indexes);
}
}
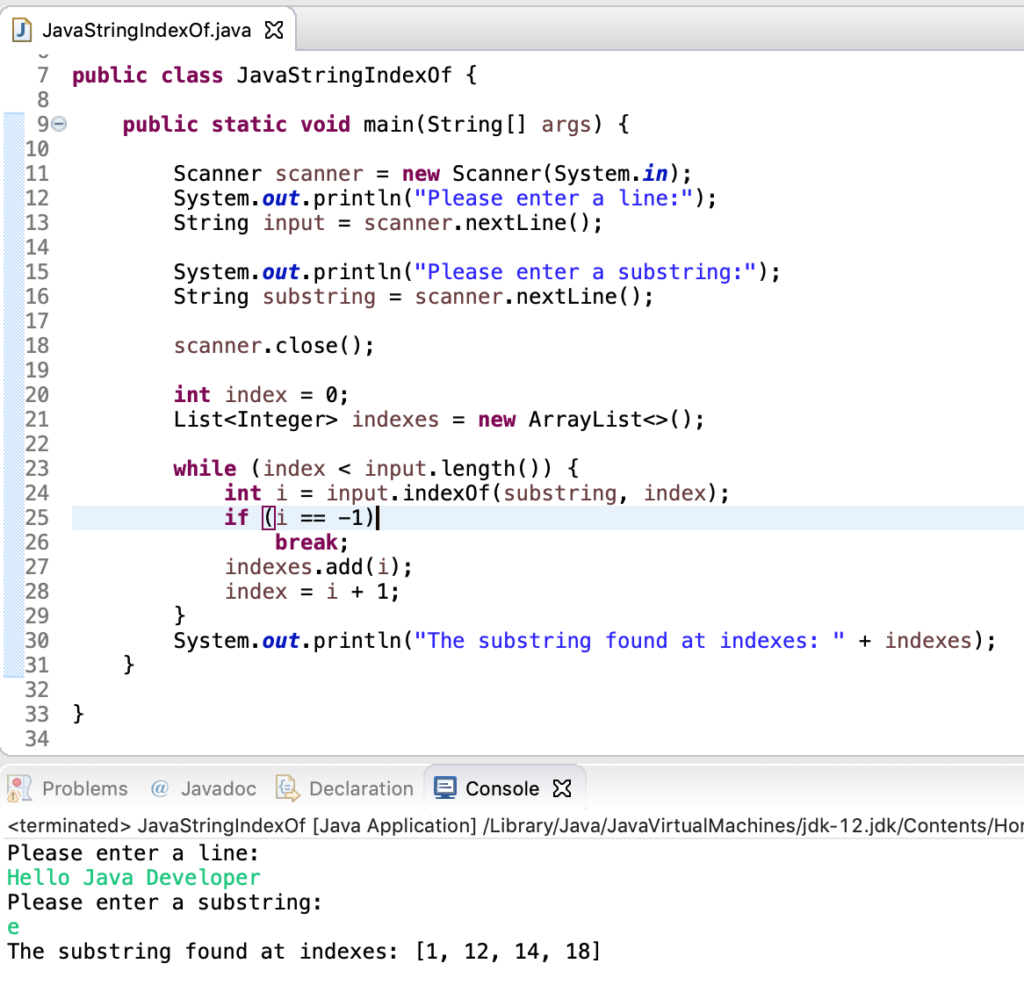
String indexOf() vs contains()
- The String contains() method is used to test if the specified substring is present in this string or not. The String indexOf() method is used to get the index of the first occurrence of a character or a substring in this string.
- The contains() method internally uses indexOf() method. It’s implemented as
return indexOf(s.toString()) >= 0
. - We can use the indexOf() method as a replacement of the contains() method. But from a readability point of view, use contains() method to test the presence of a substring.