- Java StringBuilder class is used to manipulate string objects.
- StringBuilder is a mutable sequence of characters.
- StringBuilder was introduced in Java 1.5
- StringBuilder methods are not thread-safe, so it’s recommended for the single-threaded environment.
- You should use StringBuffer in a multithreaded environment for thread safety.
- Some of the common operations StringBuilder provides are – append, insert, reverse, and delete.
Table of Contents
StringBuilder Constructors
StringBuilder class has 4 constructors.
- StringBuilder(): creates an empty string builder with a capacity of 16 characters.
- StringBuilder(int capacity): creates an empty string builder with the specified character capacity. This is useful when you know the capacity required by the string builder to save time in increasing the capacity.
- StringBuilder(String str): creates a new string builder having the same character as the string argument. The initial capacity of the string builder is “length of str + 16”.
- StringBuilder(CharSequence seq): creates a new string builder with the same characters as in the CharSequence. The initial capacity of the string builder is “length of seq + 16”.
Let’s look at some examples of creating a StringBuilder object using the above constructors.
package net.javastring.strings;
public class JavaStringBuilder {
public static void main(String[] args) {
StringBuilder sb = new StringBuilder();
System.out.println(sb.capacity());
System.out.println(sb.toString());
StringBuilder sb1 = new StringBuilder(1000);
System.out.println(sb1.capacity());
System.out.println(sb1.toString());
StringBuilder sb2 = new StringBuilder("Java");
System.out.println(sb2.capacity());
System.out.println(sb2.toString());
CharSequence seq = new StringBuilder("String");
StringBuilder sb3 = new StringBuilder(seq);
System.out.println(sb3.capacity());
System.out.println(sb3.toString());
}
}
Java StringBuilder Capacity
- StringBuilder uses a byte array to store the characters.
- When we initialize the StringBuilder, the byte array is also initialized.
- The size of the byte array can be specified through the constructor.
- The default capacity of the byte array is 16.
- When we append more characters to the string builder than the existing capacity, the byte array is reinitialized with higher capacity and the existing content.
- If you have some idea of the capacity required by the StringBuilder, use the constructor to specify the required initial capacity. It will make StringBuilder operations faster because the byte array reshuffle won’t be required.
Java StringBuilder to String
We can call the toString() method on the StringBuilder to get its string representation.
jshell> StringBuilder sb = new StringBuilder("Hello");
sb ==> Hello
jshell> String str = sb.toString();
str ==> "Hello"
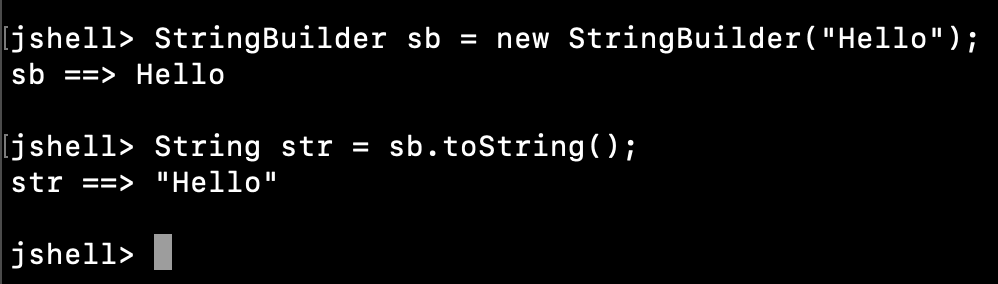
Java StringBuilder Methods
StringBuilder methods can be divided into the following categories.
- append(): many overloaded methods to append primitive as well as objects to the StringBuilder. The data is always added at the end of the string builder.
- insert(): used to insert characters at the given index. There are multiple overloaded methods for a specific type of argument.
- delete(): used to delete characters from the string builder.
- replace(): replaces the character in the string buffer.
- reverse(): reverses the character sequence. It’s useful in reversing a string because String class doesn’t have a reverse() method.
- Miscellaneous Methods: compareTo(), indexOf(), lastIndexOf()
Let’s look into some of the important StringBuilder methods one by one.
1. StringBuilder append()
- There are append() methods to add primitive data types to the string builder.
- We can append an object string representation to the string builder by calling append(Object) method.
- When an argument is an object, its toString() method is used to get the string representation.
- There are methods to append a character array to the string builder.
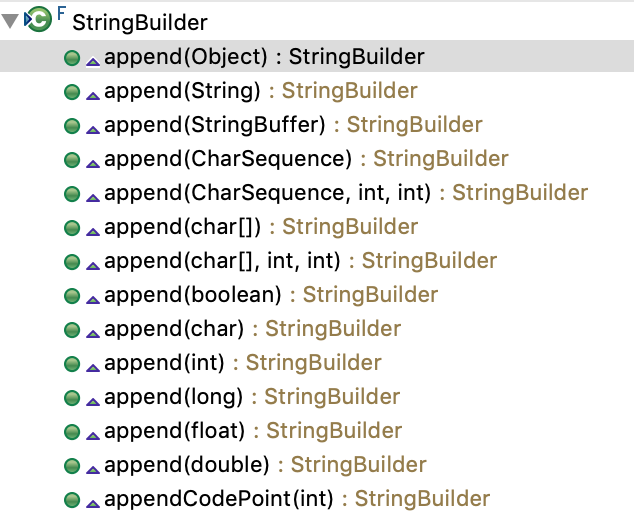
package net.javastring.strings;
public class JavaStringBuilder {
public static void main(String[] args) {
StringBuilder sb = new StringBuilder();
// append primitive data types
sb.append('a'); // append char
sb.append(true); // append boolean
sb.append(10); // append int
sb.append(12345L); // append long
sb.append(10.20); // append double
sb.append(10.20f); // append float
System.out.println(sb.toString());
// append char array
char[] charArray = { 'a', 'b', 'c', 'd' };
sb.append(charArray); // append complete char array
sb.append(charArray, 1, 2); // will append index 1 and 2
System.out.println(sb);
// append Objects
sb.append(new Object());
System.out.println(sb);
// append String, CharSequence
sb.append("Hello");
sb.append(new StringBuilder("Hi"));
sb.append(new StringBuilder("JavaString"), 4, 10);
System.out.println(sb);
}
}
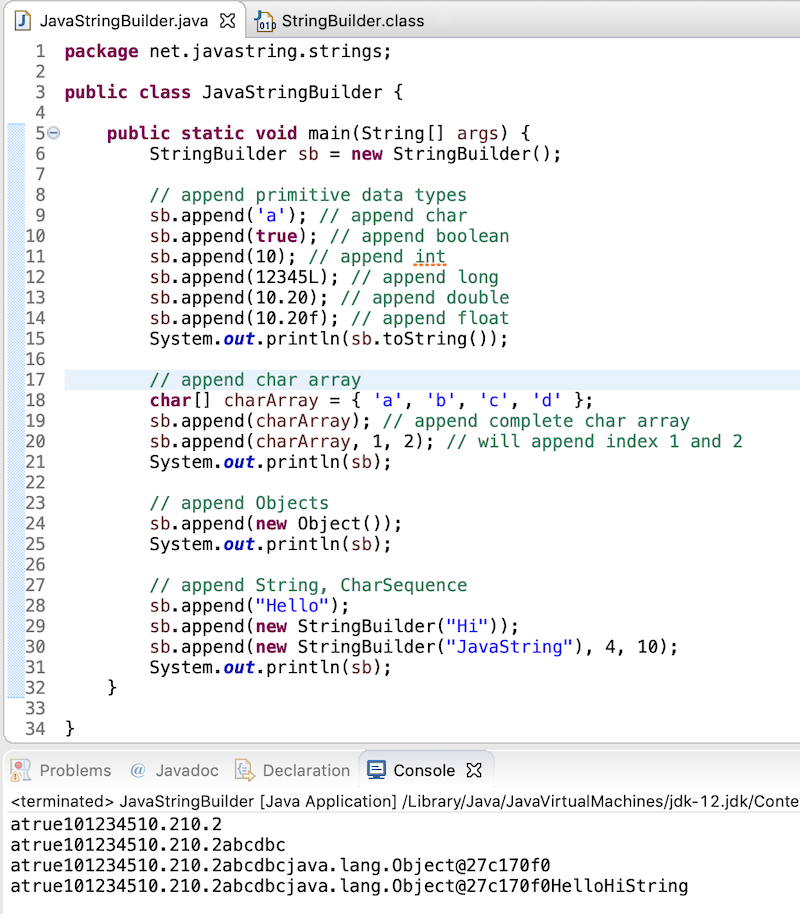
2. StringBuilder insert()
Just like append() methods, there are multiple overloaded insert() methods.
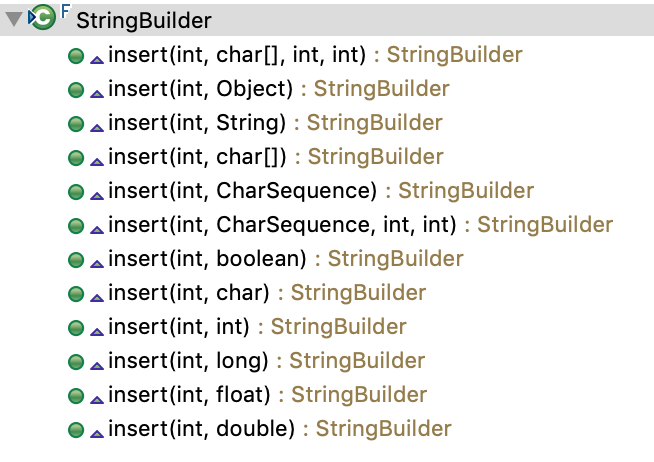
The first argument defines the index at which the second argument will be inserted. The index argument must be greater than or equal to 0, and less than or equal to the length of the string builder. If not, the StringIndexOutOfBoundsException
is thrown.
package net.javastring.strings;
public class JavaStringBuilder {
public static void main(String[] args) {
StringBuilder sb = new StringBuilder();
// insert primitive data types
sb.insert(0, 'a'); // insert char
sb.insert(1, 10); // insert int
sb.insert(1, 10.20f); // insert float
System.out.println(sb.toString());
// insert char array
char[] charArray = { '1', '2', '3'};
sb.insert(1, charArray); // insert complete char array
sb.insert(3, charArray, 1, 2); // will insert index 1 and 2
System.out.println(sb);
// insert String, CharSequence
sb.insert(9, "Java");
sb.insert(10, new StringBuilder("String"));
sb.insert(11, new StringBuilder("Developer"), 4, 6);
System.out.println(sb);
}
}
Output:
a10.210
a1223310.210
a1223310.JSlotringava210
3. StringBuilder delete()
There are two methods in StringBuilder to delete characters.
- delete(int start, int end): deletes the sequence of character from the index start to end.
- deleteCharAt(int index): deletes the character at the specified index.
Let’s use JShell this time to show the usage of StringBuilder delete() methods.
jshell> StringBuilder sb = new StringBuilder("Hello World");
sb ==> Hello World
jshell> sb.delete(6, 11)
$22 ==> Hello
jshell> sb.deleteCharAt(4)
$23 ==> Hell
4. StringBuilder replace()
The replace method syntax is: replace(
int
start,
int
end, String str)
The start and end parameters define the indexes in the string buffer that will be replaced with the str content. First, the characters between the indexes are removed. Then the specified string is inserted at the start index.
jshell> StringBuilder sb = new StringBuilder("Hello World 2019")
sb ==> Hello World 2019
jshell> sb.replace(6, 11, "JavaString");
$25 ==> Hello JavaString 2019
jshell> System.out.println(sb)
Hello JavaString 2019
5. StringBuilder reverse()
This method reverses the character sequence. It’s very useful in reversing a string.
jshell> StringBuilder sb = new StringBuilder("Hello");
sb ==> Hello
jshell> sb.reverse()
$28 ==> olleH
6. StringBuilder Miscellaneous Methods
- int compareTo(StringBuilder another): used to compare the two StringBuilder instances lexicographically.
- int indexOf(String str), indexOf(String str, int fromIndex): used to find the first occurrence of the string in the string builder.
- int lastIndexOf(String str): used to find the last index of the occurrence of the substring in the string builder.
- int lastIndexOf(String str, int fromIndex): returns the index within this string of the last occurrence of the specified substring, searching backward starting at the specified index.
package net.javastring.strings;
public class JavaStringBuilder {
public static void main(String[] args) {
StringBuilder sb = new StringBuilder("Hello Jello Trello");
StringBuilder sb1 = new StringBuilder("Hello Trello");
System.out.println(sb.compareTo(sb1));
System.out.println(sb.indexOf("ll"));
System.out.println(sb.indexOf("ll", 6));
System.out.println(sb.lastIndexOf("o"));
System.out.println(sb.lastIndexOf("o", 6));
}
}
Output:
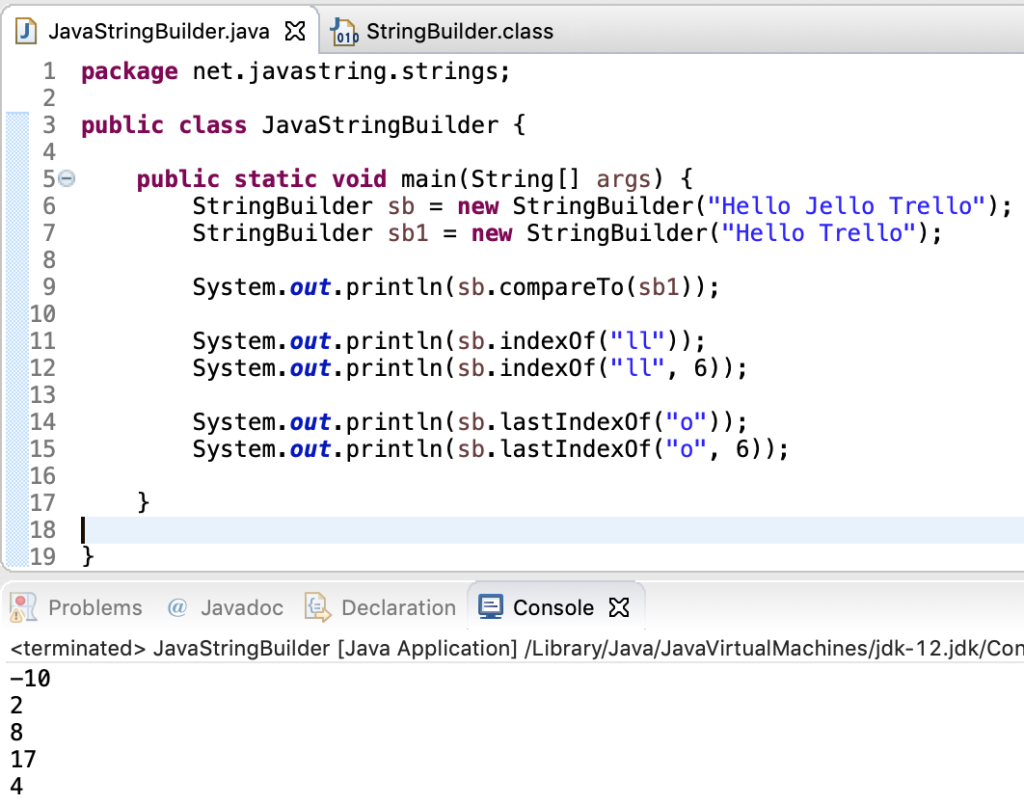
StringBuilder vs StringBuffer
- StringBuilder is not thread-safe whereas StringBuffer is thread-safe.
- StringBuilder methods are faster than StringBuffer methods because of no synchronization.
- StringBuilder doesn’t have the useless methods present in the StringBuilder class. For example, substring(), length() etc.
- StringBuilder was introduced in Java 1.5 whereas StringBuffer is present from Java 1.0 itself.
- StringBuilder is recommended for single threaded string manipulations whereas StringBuffer is recommended for the multi-threaded string manipulations.
Conclusion
Java StringBuilder is a utility class for string manipulation. You should use it for string manipulation in a single-threaded environment. For a multi-threaded environment, use its counterpart class, StringBuffer.