- Java String toUpperCase() method converts the string into the upper case.
- The characters in the string are converted to the upper case using the Locale rules.
- This method is locale-specific. So the output string can be different based on the locale settings.
- The toUpperCase() method uses Character class and standard Unicode rules to change the case of the characters.
- Since the case mapping is not always 1:1 char mappings, the output string length may be different from the source string.
- Since String is immutable, this method returns a new string and the original string remains unchanged.
Table of Contents
Java String toUpperCase() Methods
There are two variants of the toUpperCase() method.
- toUpperCase(): uses the system default locale to convert characters to upper case.
- toUpperCase(Locale locale): the characters are converted to upper case using the given locale rules.
Java String toUpperCase() Examples
Let’s look at some examples of using String toUpperCase() methods.
1. Simple String to Upper Case Conversion
jshell> Locale.getDefault()
$1 ==> en_IN
jshell> String str = "JavaString.net";
str ==> "JavaString.net"
jshell> String strUpperCase = str.toUpperCase();
strUpperCase ==> "JAVASTRING.NET"
My system default locale is set to “en_IN”, so the conversion is using English locale rules.
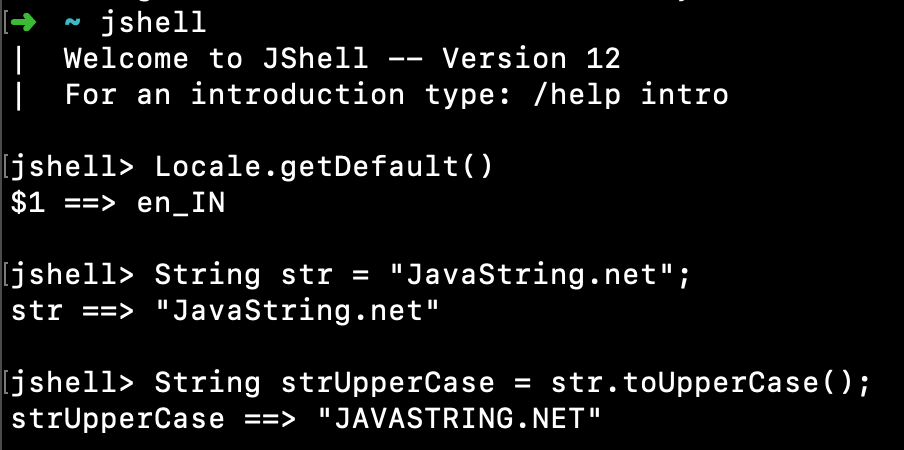
2. String to Upper Case Conversion with Locale
Let’s look at another example where we pass the Locale for the conversion rules.
jshell> String str = "title";
str ==> "title"
jshell> String strUpper = str.toUpperCase(new Locale("tr", "tur"));
strUpper ==> "TİTLE"
Notice that the second character is ‘\u005Cu0130’, which is the LATIN CAPITAL LETTER I WITH DOT ABOVE character.
We can also change the default Locale using Locale.setDefault() method. In this case, we don’t need to pass the locale object every time.
jshell> String str = "title";
str ==> "title"
jshell> Locale.setDefault(new Locale("tr", "tur"));
jshell> String strUpperCase = str.toUpperCase();
strUpperCase ==> "TİTLE"
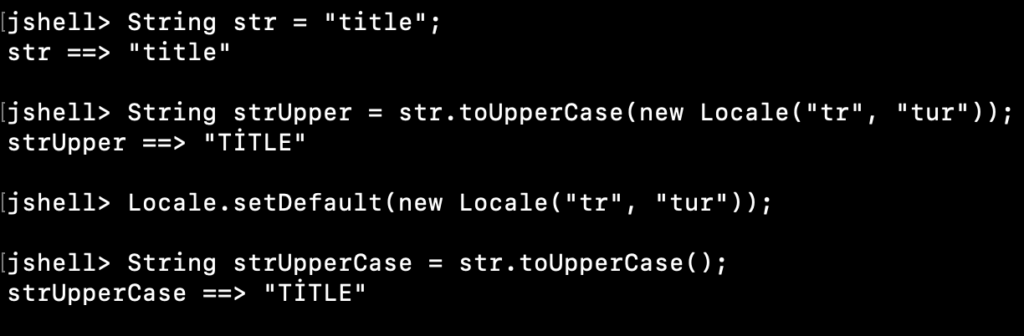
3. Locale Insensitive String to Upper Case using ROOT Locale
We can use Locale.ROOT to convert a string to upper case without locale-specific rules. The root locale language, country, and variant are empty strings.
jshell> String str = "title";
str ==> "title"
jshell> String strUpperCase = str.toUpperCase();
strUpperCase ==> "TİTLE"
jshell> String strUpperCase = str.toUpperCase(Locale.ROOT);
strUpperCase ==> "TITLE"
jshell> Locale.setDefault(Locale.ROOT);
jshell> String strUpperCase = str.toUpperCase();
strUpperCase ==> "TITLE"
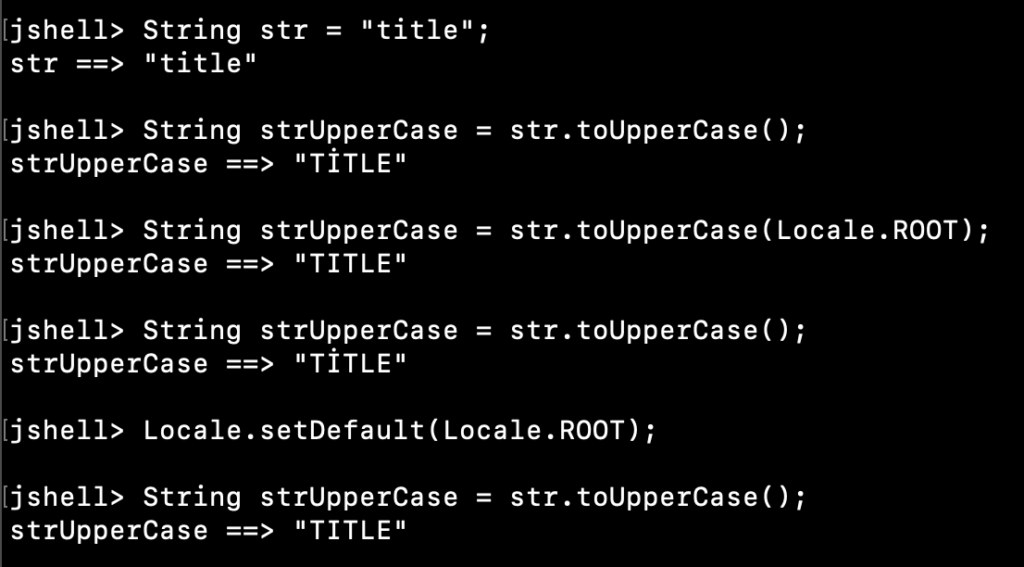
4. Change in String Length with toUpperCase()
Let’s look at an example where the string length is changed when it’s converted to upper case. There is a special character “eszett” (ß) whose upper case is “SS”.
jshell> String eszett = "ß";
eszett ==> "ß"
jshell> String eszettUpperCase = "ß".toUpperCase();
eszettUpperCase ==> "SS"
jshell> eszett.length()
$16 ==> 1
jshell> eszettUpperCase.length()
$17 ==> 2
Conclusion
Java String toUpperCase() method is used to convert the string characters to the upper case. It’s a locale-sensitive operation. It’s recommended to use ROOT locale for locale insensitive case conversion. It’s better to pass the Locale as an argument to get a consistent result and not relying on the system default locale.