Sometimes we have to remove characters from a String in Java. There is no remove()
method in String class to do this.
Table of Contents
Java Remove Character from String
Java String class has various replace()
methods. We can use this to remove characters from a string. The idea is to pass an empty string as a replacement.
Let’s look at the replace() methods present in the String class.
replace(
char
oldChar,
char
newChar)
: This method returns a new string where oldChar is replaced with newChar. This method replaces all the occurrences of the oldChar with the newChar character.replace(CharSequence target, CharSequence replacement)
: This method replaces the target substring with the replacement substring. This method replaces all the matches of target substring with the replacement substring.replaceFirst(String regex, String replacement)
: This method replaces the first match of the regex with the replacement string. This method is useful when we have to replace only the first occurrence of the substring.replaceAll(String regex, String replacement)
: It’s like the replaceFirst() method. The only difference is that all the occurrences of the matched regex are replaced with the replacement string.
NOTE: There is no empty character constant. So we can’t use the first replace(char c1, char c2)
method to remove a character from the string. We will have to use any of the other three methods by passing an empty string as a replacement.
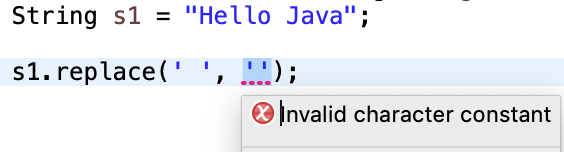
Java Remove Character from String Example
Let’s look at a simple example to remove all occurrences of a character from the string.
jshell> String s1 = "Hello";
s1 ==> "Hello"
jshell> s1.replace("l", "");
$26 ==> "Heo"
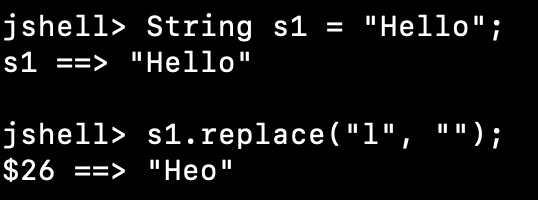
Java Remove Substring from String Example
Let’s look at an example to remove a substring from the string.
jshell> String s1 = "Java Python Spring Python";
s1 ==> "Java Python Spring Python"
jshell> String s2 = s1.replace("Python", "");
s2 ==> "Java Spring "
Java Remove Substring Regex Example
The replaceFirst()
and replaceAll()
methods accept regular expression as the first argument. We can use it to remove a pattern from the string. For example, remove all the lowercase characters from the string.
jshell> String s1 = "Hi Hello";
s1 ==> "Hi Hello"
jshell> s1.replaceAll("([a-z])", "");
$30 ==> "H H"
jshell> s1.replaceFirst("([a-z])", "");
$31 ==> "H Hello"
Remove Whitespaces from the String
Let’s look at an example to remove all the whitespaces from the string.
jshell> String s1 = "Hello World 2019";
s1 ==> "Hello World 2019"
jshell> String s2 = s1.replace(" ", "");
s2 ==> "HelloWorld2019"
What if the string has tab-character?
Let’s see how to remove tab-character and whitespaces from the string.
jshell> String s1 = "Hello World\t2019";
s1 ==> "Hello World\t2019"
jshell> String s2 = s1.replace(" ", "");
s2 ==> "HelloWorld\t2019"
jshell> String s3 = s2.replace("\t", "");
s3 ==> "HelloWorld2019"
We can also use regex for this.
jshell> String s1 = "Hello World\t2019";
s1 ==> "Hello World\t2019"
jshell> s1.replaceAll("\\s", "");
$37 ==> "HelloWorld2019"
How to Remove the Last Character from the String?
There is no method to remove the last character from the string. We can achieve this using substring()
method.
jshell> String str = "Hello World!";
str ==> "Hello World!"
jshell> str.substring(0, str.length()-1);
$39 ==> "Hello World"
Conclusion
We don’t need remove() method to remove characters from the string. The replace() methods are good enough for this task.