Java String chars() method returns an IntStream. The stream contains the integer code point values of the characters in the string object. This method was added to the String class in Java 9 release.
Table of Contents
Java String chars() Method Examples
Let’s look into some simple examples of chars() method.
1. Printing the String Character Code Points
jshell> String str = "Hello World";
str ==> "Hello World"
jshell> str.chars().forEach(System.out::println);
72
101
108
108
111
32
87
111
114
108
100
jshell>
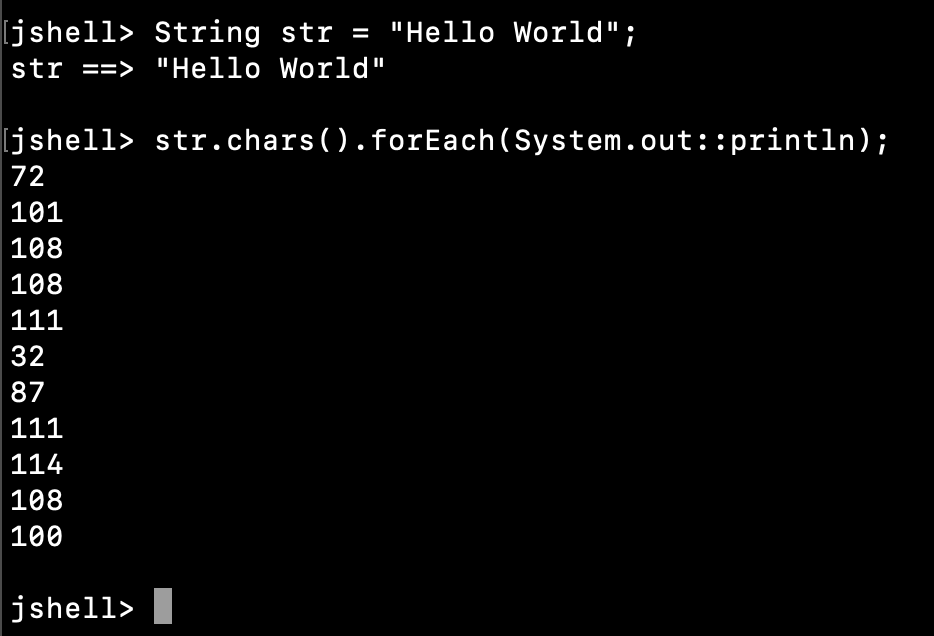
2. Processing String Characters in Parallel
If you have to process the string characters and you don’t mind unordered processing, you can create a parallel stream and process its characters.
jshell> str.chars().parallel().forEach(c -> System.out.println(Character.valueOf((char) c)));
W
o
r
l
d
l
o
H
e
l
jshell>
3. Converting String to List of Characters
We can use chars() method to get the character stream and process them one at a time. Here is an example to convert a string to list of characters while adding 1 to their code points.
jshell> String str = "Hello World";
str ==> "Hello World"
jshell> List<Character> charStr = new ArrayList<>();
charStr ==> []
jshell> str.chars().map(x -> x + 1).forEach(y -> charStr.add(Character.valueOf((char) y)));
jshell> System.out.println(charStr);
[I, f, m, m, p, !, X, p, s, m, e]
jshell>
Conclusion
Java String chars() method is useful when we have to process the characters of a large string. We can use a parallel stream for faster processing.