There are many ways to remove whitespaces from a string in Java. Note that the string is immutable, so the original string remains unchanged. We have to assign the result string to a variable to use it later on.
Table of Contents
Remove whitespace from String using replace() method
There are many characters that come under whitespace category. Some of the popular ones are space character, newline (\n), carriage return (\r), tab character (\t), etc. We can use replace() method if we know what all space characters are present in the string.
Case 1: String contains only space characters.
jshell> String str = "Hello Java";
str ==> "Hello Java"
jshell> str = str.replace(" ", "");
str ==> "HelloJava"
Case 2: String contains only space characters and tab characters.
jshell> String str = "Hello \t\t Java";
str ==> "Hello \t\t Java"
jshell> str = str.replace(" ", "").replace("\t", "");
str ==> "HelloJava"
There are many different types of whitespace characters. So using replace() method is not intuitive if you don’t know what all special whitespace characters are present in the string.
Remove whitespaces from String using replaceAll() method
Java String replaceAll() accepts a regular expression and replaces all the matches with the replacement string. The “\s” regex is used to match all the whitespace characters. So, we can use the replaceAll() method to easily replace all the whitespaces from the string.
jshell> String str1 = "Hello\t\n\r World";
str1 ==> "Hello\t\n\r World"
jshell> str1 = str1.replaceAll("\\s", "");
str1 ==> "HelloWorld"
Remove Whitespaces from String – Character.isWhitespace()
We can also write a simple utility method to remove all the whitespaces from the string. It’s just for coding practice and you should adhere to the standard methods to replace whitespaces from the string.
package net.javastring.strings;
public class JavaRemoveWhitespaceFromString {
public static void main(String[] args) {
String str2 = "Hello\n\r\t Java";
// using replaceAll()
System.out.println(str2.replaceAll("\\s", ""));
// using custom method
str2 = removeAllWhitespaces(str2);
System.out.println(str2);
}
private static String removeAllWhitespaces(String str2) {
char[] chars = str2.toCharArray();
StringBuilder sb = new StringBuilder();
for(int i = 0; i < chars.length; i++) {
if(!Character.isWhitespace(chars[i])) {
sb.append(chars[i]);
}
}
return sb.toString();
}
}
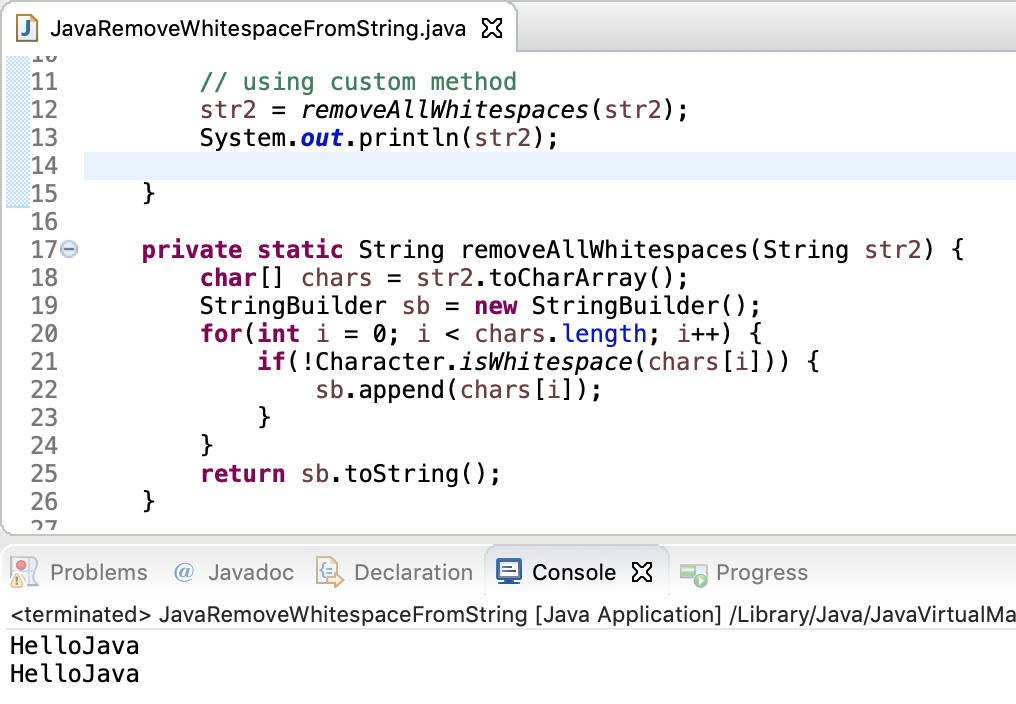
Conclusion
Removing whitespaces from a string is a very simple task. We can utilize replace() or replaceAll() methods for this.