- Java String replaceAll() method is used to replace each substring that matches the specified regular expression with the specified replacement string.
- The replaceAll() and replaceFirst() methods internally uses Matcher class.
- Both of these methods accepts two arguments – regex string and replacement string.
- If the regex is invalid, PatternSyntaxException is thrown.
- The methods were introduced in Java 1.4 release.
- The replacement of the matched substring is performed from the start of the string to the end.
Table of Contents
Java String replaceAll() and replaceFirst() Methods
The implementation of replaceAll() and replaceFirst() method is as follows:
public String replaceAll(String regex, String replacement) {
return Pattern.compile(regex).matcher(this).replaceAll(replacement);
}
public String replaceFirst(String regex, String replacement) {
return Pattern.compile(regex).matcher(this).replaceFirst(replacement);
}
String replaceAll() Regex Example
Let’s look at a simple example of replaceAll() method.
jshell> String str = "Welcome to 2019. This is year 2019";
str ==> "Welcome to 2019. This is year 2019"
jshell> String replaceAllStr = str.replaceAll("\\d{4}", "2020");
replaceAllStr ==> "Welcome to 2020. This is year 2020"
The regex “\d{4}” will match any substring having 4 digits such as 2019, 1111, etc. Then it’s replaced with “2020”.
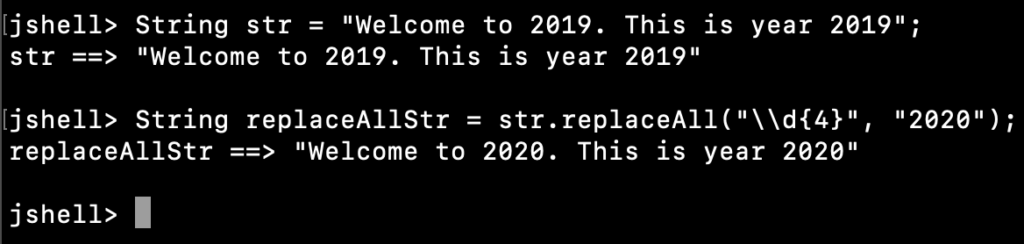
String replaceFirst() Regex Example
Let’s see what happens when the replaceFirst() method is used.
jshell> String str = "Welcome to 2019. This is year 2019";
str ==> "Welcome to 2019. This is year 2019"
jshell> String replaceFirstStr = str.replaceFirst("\\d{4}", "2020");
replaceFirstStr ==> "Welcome to 2020. This is year 2019"
Notice that only the first matched substring is replaced in this example.
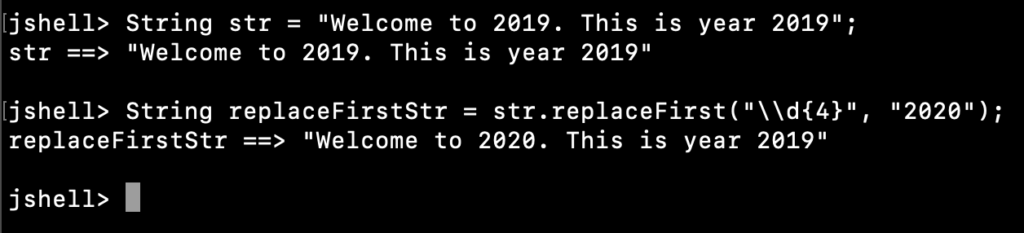
String replaceAll() without Regex
It’s not mandatory to provide a regex as the first argument. We can pass a simple string also but in that case, it will work exactly like replace() method.
jshell> String str = "Welcome to 2019. This is year 2019";
str ==> "Welcome to 2019. This is year 2019"
jshell> String replaceStr = str.replace("2019", "2020");
replaceStr ==> "Welcome to 2020. This is year 2020"
jshell> String replaceAllStr = str.replaceAll("2019", "2020");
replaceAllStr ==> "Welcome to 2020. This is year 2020"
You should use replaceAll() method only when you need a regular expression. It’s much complex and time-consuming than the normal replace() method.
String replaceAll() Case Insensitive?
The replaceAll() and replaceFirst() methods are case sensitive. We have two options for case-insensitive replacement.
- Use the Pattern class directly and initialize that with case-insensitive flag.
Pattern pattern = Pattern.compile("regex", Pattern.CASE_INSENSITIVE);
- Use “(?i)” with the regex string to perform case-insensitive matching.
jshell> String str = "Hello WORLD";
str ==> "Hello WORLD"
jshell> str.replaceAll("(?i)l", "l");
$29 ==> "Hello WORlD"
jshell> str.replaceAll("(?i)L", "l");
$30 ==> "Hello WORlD"
String replaceAll() with backslash (\) and Dollar Sign ($)
According to String replaceAll() documentation:
Note that backslashes (
https://docs.oracle.com/en/java/javase/12/docs/api/java.base/java/lang/String.html#replaceAll(java.lang.String,java.lang.String)\
) and dollar signs ($
) in the replacement string may cause the results to be different than if it were being treated as a literal replacement string.
Let’s see what happens when we try to replace backslash or dollar signs from a string using the replaceAll() method.
jshell> String str = "Hi\\Hello\\Yes$Yo$Yup";
str ==> "Hi\\Hello\\Yes$Yo$Yup"
jshell> String newStr = str.replaceAll("\\", "");
| Exception java.util.regex.PatternSyntaxException: Unexpected internal error near index 1
\
| at Pattern.error (Pattern.java:2015)
| at Pattern.compile (Pattern.java:1784)
| at Pattern.<init> (Pattern.java:1427)
| at Pattern.compile (Pattern.java:1068)
| at String.replaceAll (String.java:2135)
| at (#102:1)
jshell> String newStr = str.replaceAll("$", "");
newStr ==> "Hi\\Hello\\Yes$Yo$Yup"
- When we tried to replace the $ sign, there was no effect and the original string is returned.
- When we tried to replace backslash, the PatternSyntaxException is thrown.
The reason for this unusual behavior is that these characters have special meaning in regular expressions.
So, How to replace backslash and dollar sign characters?
It’s simple, just use plain replace() method because you want to treat them as a normal string.
jshell> String str = "Hi\\Hello\\Yes$Yo$Yup";
str ==> "Hi\\Hello\\Yes$Yo$Yup"
jshell> String newStr = str.replace("\\", "");
newStr ==> "HiHelloYes$Yo$Yup"
jshell> String newStr = str.replace("$", "");
newStr ==> "Hi\\Hello\\YesYoYup"
Conclusion
Java String replaceAll() method is very powerful. You should take extra precaution when using regular expressions to avoid replacing something that you didn’t intend to. This method is slow when compared to replace() method, so don’t use it to replace a substring with another substring.