Java String transform() method is used to apply a function to this string. The function should accept a single string argument and return an object. This utility method was added to String class in Java 12 release.
Table of Contents
String transform() Method Syntax
The transform method is defined as follows:
public <R> R transform(Function<? super String, ? extends R> f) {
return f.apply(this);
}
The Function
is a functional interface that accepts one argument and produces a result object.
Java String transform() Method Examples
Let’s look at some examples of using the transform() method.
1. Transforming CSV String to List of Strings
Here is a simple example of implementing Function and then using it to transform CSV string to a list of strings. We will use the String split() method in conjunction with the transform() method.
package net.javastring.strings;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import java.util.function.Function;
public class JavaStringTransform {
public static void main(String[] args) {
String str = "Java,Python,Android";
// using Function implementation
List<String> list1 = str.transform(new TransformFunction());
System.out.println(list1);
}
class TransformFunction implements Function<String, List<String>> {
@Override
public List<String> apply(String t) {
return Arrays.asList(t.split(","));
}
}
Output: [Java, Python, Android]
We can also use anonymous class implementation of Function interface.
// using anonymous class
List<String> list2 = str.transform(new Function<String, List<String>>() {
@Override
public List<String> apply(String t) {
return Arrays.asList(t.split(","));
}
});
System.out.println(list2);
Since Function is a functional interface, we can reduce the code size even further by using lambda expressions.
// using lambda expressions
List<String> list3 = str.transform(s1 -> Arrays.asList(s1.split(",")));
System.out.println(list3);
The output will remain the same in all the approaches. However, the code size will reduce a lot with lambda expressions.
2. Transforming String to a List of Objects
Let’s look at a slightly more complex example where we will transform the string to create a list of objects.
Let’s say the string is a comma-separated list of employee ids, we will use transform() method to create a list of employees from this string.
package net.javastring.strings;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import java.util.function.Function;
public class JavaStringTransform {
public static void main(String[] args) {
// converting string to other objects
String ids = "1,2,3";
List<Employee> list4 = ids.transform(csvStr -> {
String[] idArray = csvStr.split(",");
List<Employee> empList = new ArrayList<>();
for (String s1 : idArray) {
empList.add(new Employee(Integer.parseInt(s1)));
}
return empList;
});
System.out.println(list4);
}
}
class Employee {
private int id;
Employee(int i) {
this.id = i;
}
@Override
public String toString() {
return String.format("Emp[%d]", this.id);
}
}
Output:
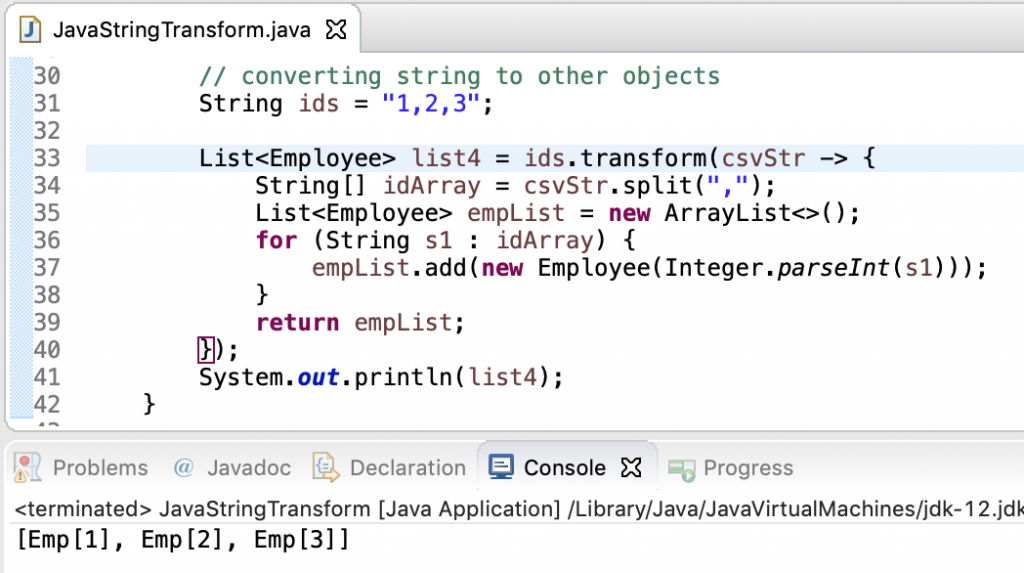
Conclusion
Java String transform() is a great utility method to use the string object to create other objects. If there is any exception thrown by the Function apply() method, it will be propagated to the caller program.