- Java String concatenation is the process of creating a new string by combining two or more strings.
- The String is the only class that supports “+” operator for string concatenation.
- We can also use String class concat() method to concatenate two strings.
- If we use + operator with String and primitive types, then the output is a string. The string representation of primitive type is used for the concatenation.
- When we use + operator with an object, its
toString()
method is called to get the string representation. Then both the strings are concatenated. - When we have to concatenate a large number of strings, use of StringBuilder and StringBuffer is recommended.
- StringBuilder is used to concatenate a large number of strings in the single-threaded environment.
- If you are working on a multithreaded environment, use StringBuffer for thread safety to concatenate strings.
Table of Contents
Using + for String Concatenation
Let’s look at some examples of concatenating strings using the + operator.
1. Concatenating Strings
jshell> String s1 = "Hello";
s1 ==> "Hello"
jshell> String s2 = "World";
s2 ==> "World"
jshell> String message = s1 + " " + s2;
message ==> "Hello World"
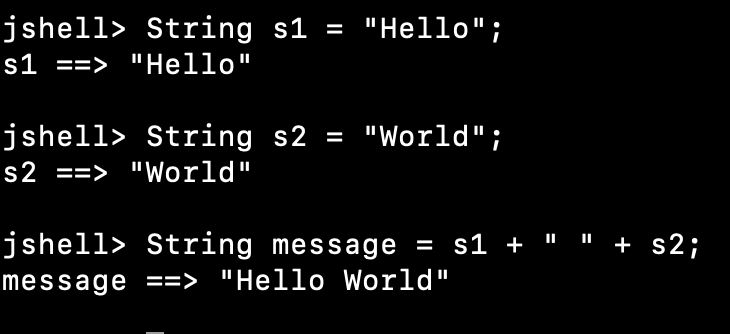
When we concatenate string constant expressions, it’s evaluated at the compile time and then treated as a string literal. So the string is created in the string pool area.
But, when we are concatenating string variables, the concatenation happens at runtime and the string object is created in the heap area. Let’s confirm this behavior with a simple example.
jshell> String s1 = "Ja";
s1 ==> "Ja"
jshell> String s2 = "va";
s2 ==> "va"
jshell> "Java" == "Ja" + "va" // compile time concatenation
$10 ==> true
jshell> "Java" == s1 + s2 // runtime concatenation
$11 ==> false
2. Concatenating String with a Primitive Type
jshell> String out = "Hello " + 2019
out ==> "Hello 2019"
jshell> String msg = "true " + true
msg ==> "true true"
3. Concatenating String with an Object
jshell> Object obj = new Object();
obj ==> java.lang.Object@3cd1f1c8
jshell> String str = "Hello " + obj
str ==> "Hello java.lang.Object@3cd1f1c8"
jshell> obj.toString()
$66 ==> "java.lang.Object@3cd1f1c8"
Using String.concat() Method
When we use the concat() method to concatenate two strings, it returns a new string. The original string remains unchanged since the string is immutable. We have to assign the output to a new string variable.
Since concat() method returns a new string, we can create a chain to concatenate multiple strings.
jshell> String hi = "Hello".concat(" ").concat("World")
hi ==> "Hello World"
StringBuilder and StringBuffer for String Concatenation
When we have to concatenate a large number of strings, StringBuilder and StringBuffer are preferred. Both of these classes behave in the same way. The only difference is that the StringBuffer is thread-safe whereas StringBuilder is not thread-safe.
Here is an example to concatenate a list of thousand strings using StringBuilder and StringBuffer.
package net.javastring.strings;
import java.util.ArrayList;
import java.util.List;
public class JavaStringConcatanation {
public static void main(String[] args) {
// concatenating large number of strings
List<String> numbers = new ArrayList<>();
for (int i = 0; i < 1000; i++) {
numbers.add(i, String.valueOf(i));
}
// using StringBuilder
StringBuilder sb = new StringBuilder();
for (String s : numbers)
sb.append(s);
System.out.println(sb.toString());
// using StringBuffer
StringBuffer sb1 = new StringBuffer();
for (String s : numbers)
sb1.append(s);
System.out.println(sb1.toString());
}
}
Java String Concatenation Performance
- If you are concatenating a few strings, using + operator or concat() method is fine because the performance gain with StringBuffer and StringBuilder will be negligible.
- For a single-threaded environment, use StringBuilder to concatenate a large number of strings.
- For a multi-threaded environment, use StringBuffer to concatenate a large number of strings.