Java String Pool is the special area in the heap memory to store string objects. There are two ways to create string objects. If we use double quotes to create a string object, it’s stored in the string pool. If we use the new operator to create a string, it’s created in the heap memory.
Table of Contents
How Does Java String Pool Works?
- When we create a string literal, it’s stored in the string pool.
- If there is already a string with the same value in the string pool, then new string object is not created. The reference to the existing string object is returned.
- Java String Pool is a cache of string objects. It’s possible because string is immutable.
- If we create a string object using new operator, it’s created in the heap area. If we want to move it to the string pool, we can use
intern()
method. - String Pool is a great example of Flyweight design pattern.
Understanding String Pool With a Program
Let’s understand the working of string pool with a simple program.
package net.javastring.strings;
public class JavaStringPool {
public static void main(String[] args) {
String s1 = "Hello";
String s2 = "Hello";
String s3 = new String("Hi");
String s4 = "Hi";
System.out.println("s1 == s2? " + (s1 == s2));
System.out.println("s3 == s4? " + (s3 == s4));
s3 = s3.intern();
System.out.println("s3 == s4? " + (s3 == s4));
}
}
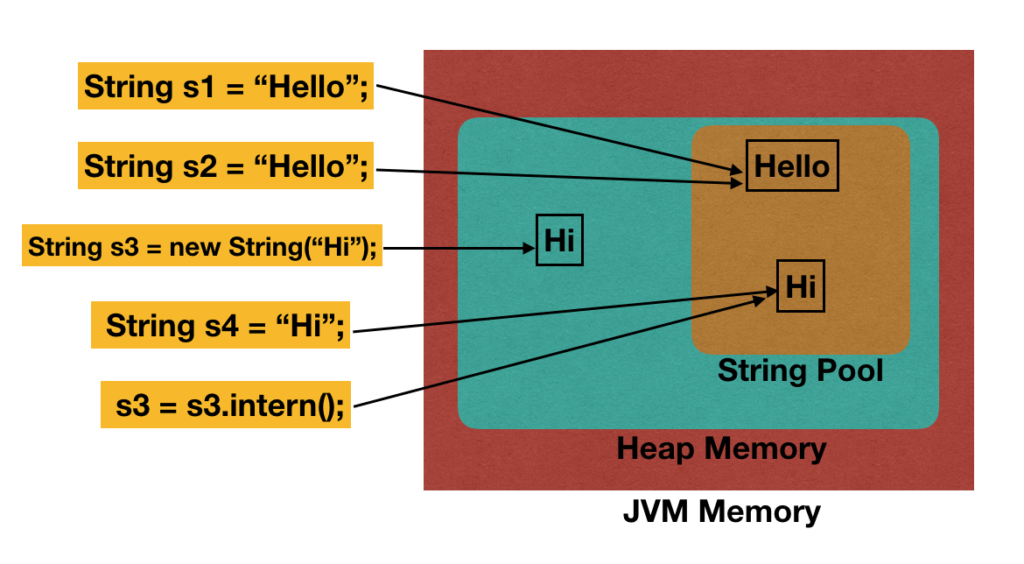
- When we create the first string s1, there is no string with the value “Hello” in the string pool. So a string “Hello” is created in the pool and its reference is assigned to s1.
- When we are creating second string s2, there is already a string with the value “Hello” in the string pool. The existing string reference is assigned to s2.
- When we create third string s3, it’s created in the heap area because we are using the new operator.
- When we create fourth string s4, a new string with the value “Hi” is created in the pool and its reference is assigned to s4.
- When we compare
s1 == s2
, it returnstrue
because both the variables are referring to the same string object. - When we compare
s3 == s4
, it returns false because they point to the different string objects. - When we call
intern()
method on s3, it checks if there is any string in the pool with the value “Hi”? Since we already have a string with this value in the pool, its reference is returned and assigned to s3. - Now
s3 == s4
returnstrue
because both the variables are referring to the same string objects.
What are the Benefits of Java String Pool?
Let’s look at some of the benefits of java string pool.
- Java String Pool allows caching of string objects. This saves a lot of memory for JVM that can be used by other objects.
- Java String Pool helps in better performance of the application because of reusability. It saves time to create a new string if there is already a string present in the pool with the same value.
Why we should never create String using new operator?
Let’s look at the code snippet to create a string using new operator.
String s3 = new String("Hi");
- A new string with value “Hi” is created in the string pool.
- Then the String constructor is called by passing the string created in the above step.
- A new string is created in the heap space and its reference is assigned to s3.
- The string created in the first step is orphan and available for garbage collection.
- We ended up creating two strings when we wanted to create a single string in the heap area.