Java String repeat() method returns a new string whose value is the concatenation of this string given number of times.
Table of Contents
Java String repeat(int count) Important Points
- If the count is 0, the empty string is returned.
- If the count is negative,
IllegalArgumentException
is thrown. - If the count is 1, the reference to this string is returned.
- If this string is empty, then an empty string is returned.
- This utility method was added to String class in Java 11 release.
- This method internally calls
Arrays.fill()
andSystem.arraycopy()
methods to create the new string.
String repeat() Method Examples
Let’s look at some examples of String repeat() method.
jshell> String str = "Hello";
str ==> "Hello"
jshell> String repeatedStr = str.repeat(3);
repeatedStr ==> "HelloHelloHello"
Let’s look at the exception when the count is negative.
jshell> "Hi".repeat(-2)
| Exception java.lang.IllegalArgumentException: count is negative: -2
| at String.repeat (String.java:3240)
| at (#13:1)

Let’s see what happens when the count is 0.
jshell> "Java".repeat(0)
$14 ==> ""
Let’s see what happens when the source string is empty.
jshell> "".repeat(10)
$15 ==> ""
jshell> "".repeat(0)
$16 ==> ""
jshell> "".repeat(-2)
| Exception java.lang.IllegalArgumentException: count is negative: -2
| at String.repeat (String.java:3240)
| at (#17:1)
jshell>
Let’s see what happens when the count is 1.
jshell> String s1 = "Java";
s1 ==> "Java"
jshell> String s2 = s1.repeat(1)
s2 ==> "Java"
jshell> s1 == s2
$20 ==> true
The output of == operator confirms that both the string variables are referring to the same string object.
OutOfMemoryError when repeat count is huge
If you look at the repeat() method implementation, there is a condition to check if the resulting string length will be too big? If yes, then throw OutOfMemoryError.
if (Integer.MAX_VALUE / count < len) {
throw new OutOfMemoryError("Repeating " + len + " bytes String " + count +
" times will produce a String exceeding maximum size.");
}
Let’s see if we get this error by providing a very large value for the repeat count.
jshell> "Hello".repeat(Integer.MAX_VALUE)
| Exception java.lang.OutOfMemoryError: Repeating 5 bytes String 2147483647 times will produce a String exceeding maximum size.
| at String.repeat (String.java:3255)
| at (#22:1)
jshell> "Hello".repeat(Integer.MAX_VALUE - 2)
| Exception java.lang.OutOfMemoryError: Repeating 5 bytes String 2147483645 times will produce a String exceeding maximum size.
| at String.repeat (String.java:3255)
| at (#23:1)
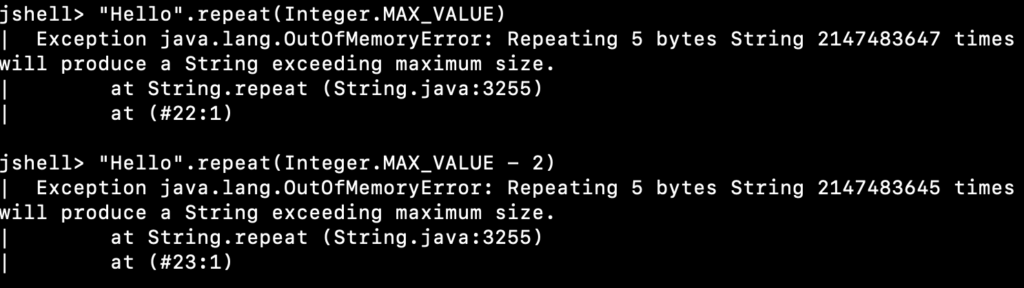
Conclusion
Java String repeat() is a simple utility method that can be used to concatenate a string specific number of times. It may produce OutOfMemoryError if the resulting string length is too large.