String comparison is a common operation in programming. We compare two strings to check if they are equal or not. Sometimes, we compare two strings to check which of them comes first lexicographically. This is useful when we have to sort a collection of strings. In this tutorial, we will look at the various ways for string comparison in Java.
Table of Contents
String Comparison in Java
We can compare two strings using following ways.
- equals() method
- == operator
- compareTo() method
- compareToIgnoreCase() method
Collator
compare() method
Let’s look into these string comparison methods one by one.
1. equals() method
We can compare two strings for equality using equals() method. Java string is case sensitive, so the comparison is also case sensitive. If you want to test equality without case consideration, use equalsIgnoreCase()
method.
jshell> String s1 = "Hello";
s1 ==> "Hello"
jshell> s1.equals("Hello");
$2 ==> true
jshell> s1.equals("HELLO");
$3 ==> false
jshell> s1.equalsIgnoreCase("HELLO");
$4 ==> true
jshell>
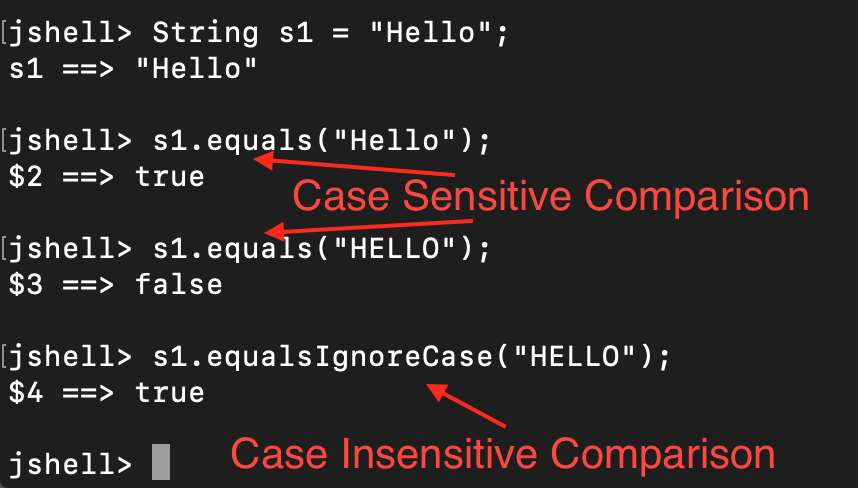
2. == operator
Java == operator returns true if both the variables are referring to the same object. Java String is immutable, so there is no point in using == for checking equality. You should always use the equals()
method for that.
Let’s look at some examples of using == operator for string comparison.
String s1 = "Hello";
String s2 = "Hello";
String s3 = new String("Hello");
System.out.println(s1 == s2); // true
System.out.println(s1 == s3); // false
In the above code snippet, all the string objects have the same value. Strings s1 and s2 are in the pool referring to the same object. String s3 is created in the heap space because of new
operator. That’s why s1==s2
is true
and s1==s3
is false
.
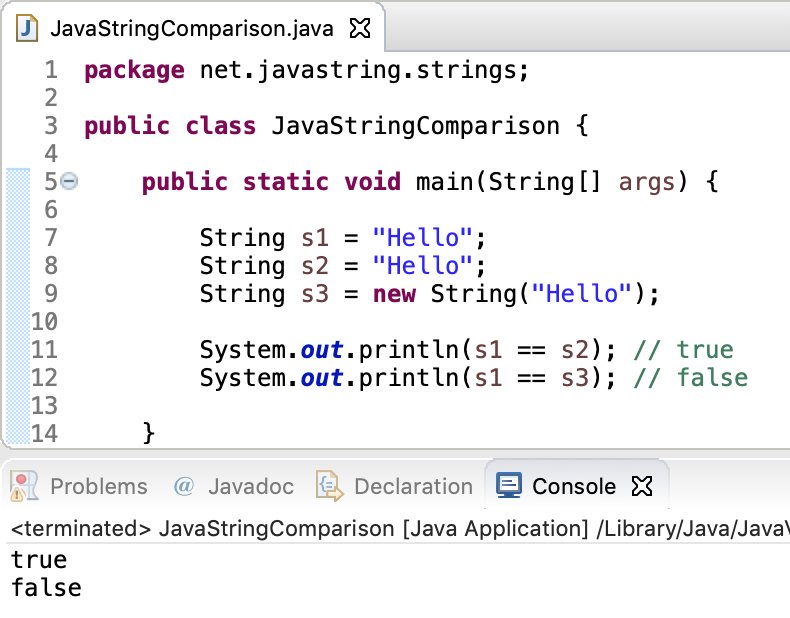
3. compareTo() Method
This method compares the two string lexicographically. The output is always an integer value. It depends on the following comparison rules. String class implements Comparable
interface. This is the method used when we try to sort an array or collection of strings.
- If the string comes before the argument string, the output is negative.
- The characters in both strings are compared one by one.
- If the characters at any index don’t match, then the difference between their code points is the output. So the output will be
this.charAt(n)-argumentString.charAt(n)
if the characters don’t match at index ‘n’. - If the characters match at all the indexes, then the shorter length string precedes the longer length string. So the output is negative. The value is the difference between the lengths of the strings.
- If both the strings have the same value, the output is 0. In this case,
equals()
between these two strings must betrue
.
Let’s look at some examples of compareTo() method and understand the output.
"Hello".compareTo("Hi")
: returns -4 because the characters are different at index 1. Code point of ‘e’ is 101 and the code point of ‘i’ is 105. The difference between them is -4."Hi".compareTo("Hi")
: returns 0 because both the strings are equal."Hi".compareTo("Hii")
: returns -1. The characters are the same in all the indexes of both the strings. The output is negative because “Hi” comes before “Hii”. The output value is 1 because their length difference is 1."Hii".compareTo("Hi")
: returns 1. The output is positive because “Hii” comes after “Hi”. The difference in their length is 1."He".compareTo("Hello")
: returns -3. You can figure out the output using the above logic.- “Hello”.compareTo(“HI”): returns 28. The code point of ‘e’ is 101 and ‘I’ is 73.
4. compareToIgnoreCase() Method
This method works in the same way as compareTo() method. The only difference is that the comparison is case insensitive. The strings are converted to upper case or lower case before the comparison. This is useful when you want to sort a collection of strings without considering their case.
Let’s look at some examples of compareToIgnoreCase() method.
package net.javastring.strings;
public class JavaStringComparison {
public static void main(String[] args) {
System.out.println("H".compareToIgnoreCase("h")); // 0
System.out.println("HI".compareToIgnoreCase("hi")); // 0
System.out.println("Hello".compareTo("HI")); // 28
System.out.println("HI".codePointAt(1)); // 73
System.out.println("Hello".compareToIgnoreCase("HI")); // -4
}
}
Output:
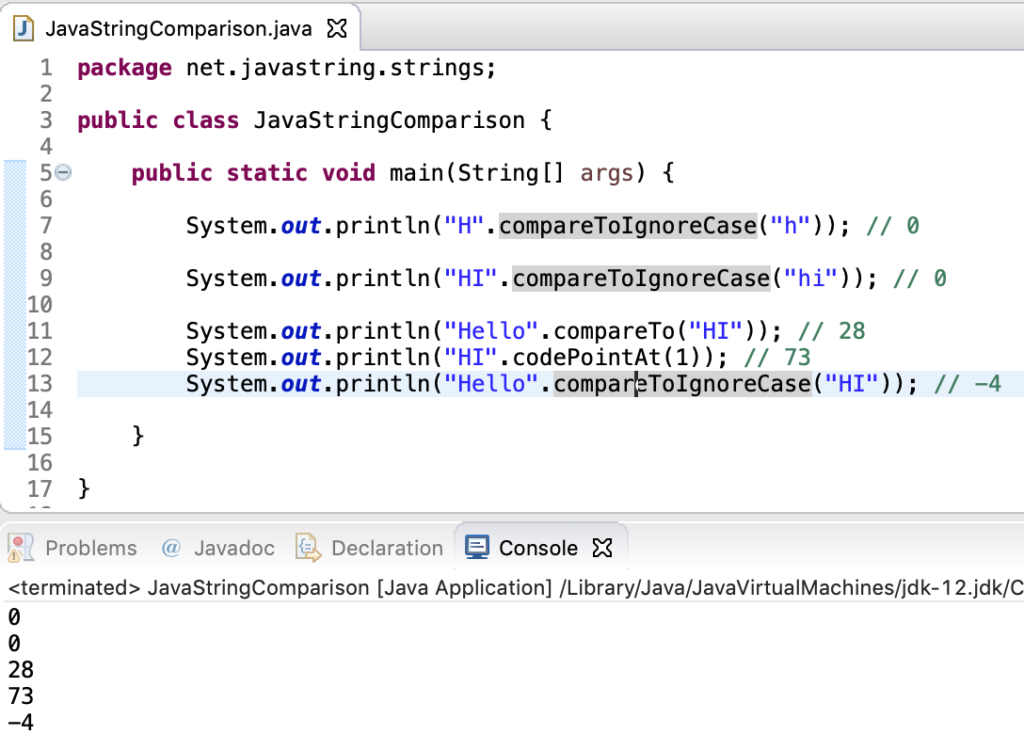
5. Collator class for locale-specific comparison
We can use Collator class for locale-specific string comparison. We can define our own comparison rules to compare the strings. Let’s look at a simple example of using Collator class for locale-specific comparison. We will also check how to define the custom comparison rules.
package net.javastring.strings;
import java.text.Collator;
import java.text.ParseException;
import java.text.RuleBasedCollator;
import java.util.Locale;
public class JavaStringComparisonCollator {
public static void main(String[] args) throws ParseException {
Collator collator = Collator.getInstance();
Collator collatorDE = Collator.getInstance(Locale.GERMANY);
System.out.println(collator.compare("ABC", "B")); // -1
System.out.println(collatorDE.compare("ABC", "B")); // -1
String rules = "< B < A";
RuleBasedCollator rbc = new RuleBasedCollator(rules);
System.out.println(rbc.compare("ABC", "B")); // 1
}
}
Conclusion
There are various ways to compare two strings.
- If you want to check for equality, then use equals() method.
- For lexicographical comparison, use
compareTo()
method. - For case insensitive lexicographically comparison, use
compareToIgnoreCase()
method. - If you want a locale-specific comparison, use
Collator
class. - For specifying your own comparison rules, use
RuleBasedCollator
class.