Java String valueOf() method is used to get the string representation of the argument. There are various overloaded valueOf() methods that accept primitive data types, char array, and object. The String valueOf() methods are static.
Table of Contents
Java String valueOf() Methods
If you look at the String class, there are 9 valueOf() methods.
- valueOf(boolean b): returns “true” or “false” based on the boolean argument value.
- valueOf(char c): returns the string representation of the character argument.
- valueOf(int i): returns the string representation of the integer argument. It calls
Integer.toString(i)
internally. - valueOf(long l): internally calls
Long.
toString
(l)
to get the string representation of the long value. - valueOf(float f): calls the
Float.toString(f)
to get the string representation of the float. - valueOf(double d): calls
Double.
toString
(d)
to get the string representation of the double value. - valueOf(Object obj): returns “null” if the object is null. Otherwise calls the object toString() method to get the string representation of the object.
- valueOf(char data[]): returns the string representation of the char array.
- valueOf(char data[], int offset, int count): creates a string from the subarray of the char array argument. This method throws StringIndexOutOfBoundsException if the
offset
is negative, orcount
is negative, oroffset+count
is larger thandata.length
.
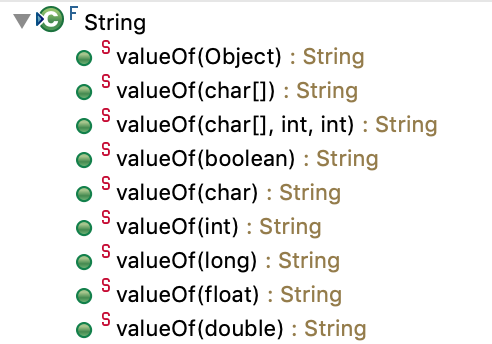
The valueOf() method uses the new operator to create the string. So, the string is created in the heap space. If you want to save memory, you can use the intern() method to move it to the string pool.
Java String valueOf() Examples
Let’s look at some examples of String valueOf() methods. We will use JShell to run the code snippets.
1. valueOf(boolean b)
➜ ~ jshell
| Welcome to JShell -- Version 12
| For an introduction type: /help intro
jshell> String str = String.valueOf(true);
str ==> "true"
jshell> String str = String.valueOf(false);
str ==> "false"
2. valueOf(char c)
jshell> String ch = String.valueOf('a')
ch ==> "a"
jshell> String ch = String.valueOf('\u0043')
ch ==> "C"
3. valueOf(int i)
jshell> String is = String.valueOf(55);
is ==> "55"
jshell> String is = String.valueOf(Integer.MAX_VALUE);
is ==> "2147483647"
jshell> String is = String.valueOf(Integer.MIN_VALUE);
is ==> "-2147483648"
jshell> String is = String.valueOf(5*5-2);
is ==> "23"
jshell> String is = String.valueOf(0xFF);
is ==> "255"
jshell> String is = String.valueOf(0b1101);
is ==> "13"
4. valueOf(long l)
jshell> String longStr = String.valueOf(Long.MIN_VALUE);
longStr ==> "-9223372036854775808"
jshell> String longStr = String.valueOf(Long.MAX_VALUE);
longStr ==> "9223372036854775807"
jshell> String longStr = String.valueOf(12345L);
longStr ==> "12345"
jshell> String longStr = String.valueOf(12345l);
longStr ==> "12345"
5. valueOf(float f)
jshell> String floatStr = String.valueOf(12.34);
floatStr ==> "12.34"
jshell> String floatStr = String.valueOf(12.34F);
floatStr ==> "12.34"
jshell> String floatStr = String.valueOf(12.34f);
floatStr ==> "12.34"
jshell> String floatStr = String.valueOf(Float.MAX_VALUE);
floatStr ==> "3.4028235E38"
jshell> String floatStr = String.valueOf(Float.MIN_VALUE);
floatStr ==> "1.4E-45"
jshell> String floatStr = String.valueOf(Float.NaN);
floatStr ==> "NaN"
jshell> String floatStr = String.valueOf(Float.POSITIVE_INFINITY);
floatStr ==> "Infinity"
jshell> String floatStr = String.valueOf(Float.NEGATIVE_INFINITY);
floatStr ==> "-Infinity"
jshell> String floatStr = String.valueOf(Float.MIN_NORMAL);
floatStr ==> "1.17549435E-38"
Float class contains many float constants and their string representation are not exactly floating-point numbers.
6. valueOf(double d)
jshell> String doubleStr = String.valueOf(12.34d);
doubleStr ==> "12.34"
jshell> String doubleStr = String.valueOf(12.34D);
doubleStr ==> "12.34"
jshell> String doubleStr = String.valueOf(Double.MAX_VALUE);
doubleStr ==> "1.7976931348623157E308"
jshell> String doubleStr = String.valueOf(Double.MIN_VALUE);
doubleStr ==> "4.9E-324"
jshell> String doubleStr = String.valueOf(Double.POSITIVE_INFINITY);
doubleStr ==> "Infinity"
jshell> String doubleStr = String.valueOf(Double.NEGATIVE_INFINITY);
doubleStr ==> "-Infinity"
7. valueOf(Object obj)
jshell> String objStr = String.valueOf(new Object());
objStr ==> "java.lang.Object@1698c449"
jshell> String objStr = String.valueOf(new Exception("Exception Message"));
objStr ==> "java.lang.Exception: Exception Message"
8. valueOf(char[] data)
jshell> char[] chars = {'a', 'b', 'c', 'd', 'e'};
chars ==> char[5] { 'a', 'b', 'c', 'd', 'e' }
jshell> String str = String.valueOf(chars);
str ==> "abcde"
jshell> char[] data = {}
data ==> char[0] { }
jshell> String str = String.valueOf(data);
str ==> ""
If the char array is empty, then empty string is returned.
9. valueOf(char[] data, int offset, int count)
jshell> char[] vowels = {'a', 'e', 'i', 'o', 'u'}
vowels ==> char[5] { 'a', 'e', 'i', 'o', 'u' }
jshell> String vs = String.valueOf(vowels, 1, 2);
vs ==> "ei"
Let’s look at some examples of StringIndexOutOfBoundsException when calling this method.
jshell> String vs = String.valueOf(vowels, -1, 2);
| Exception java.lang.StringIndexOutOfBoundsException: offset -1, count 2, length 5
| at String.checkBoundsOffCount (String.java:3395)
| at String.rangeCheck (String.java:289)
| at String.<init> (String.java:285)
| at String.valueOf (String.java:3080)
| at (#40:1)
jshell> String vs = String.valueOf(vowels, 1, 200);
| Exception java.lang.StringIndexOutOfBoundsException: offset 1, count 200, length 5
| at String.checkBoundsOffCount (String.java:3395)
...
10. Java String valueOf(null)
- When we pass null as argument, the valueOf(char[] data) method gets called and NullPointerException is thrown.
- If we pass an object that is null, the valueOf(Object obj) method gets called and “null” string is returned.
jshell> String nullStr = String.valueOf(null);
| Exception java.lang.NullPointerException
| at String.<init> (String.java:260)
| at String.valueOf (String.java:3056)
| at (#42:1)
jshell> Object obj = null;
obj ==> null
jshell> String nullStr = String.valueOf(obj);
nullStr ==> "null"
Java String copyValueOf() Methods
There are two copyValueOf() methods in String class that does exactly the same thing as valueOf(char[]) methods.
- copyValueOf(char data[]): equivalent to
valueOf(char[])
method. - copyValueOf(char data[], int offset, int count): same as
valueOf(char[] data, int offset, int count)
method.
valueOf() vs copyValueOf()
Both of these methods behave exactly the same. There are no performance benefits of using one over the another. However, removing copyValueOf() method doesn’t make sense because it will break backward compatibility. You can check out this StackOverflow question to read more on this topic.
Conclusion
Java String valueOf() methods provide simple utility to get the string representation of different data types. The string object is created in the heap space, so if it’s huge then you can use intern() method to move it to the string pool.