- Java String trim() method is used to remove all the leading and trailing white spaces from the string.
- The trim() method returns a new string and the original string remains unchanged.
- The white space is considered as any character whose Unicode code point is less than or equal to
'U+0020'
(the space character). - If the string is empty, or there are no leading and trailing white spaces then the reference to this string is returned.
- If the string contains only white spaces, then the empty string is returned.
- This method is not suitable to trim special types of white spaces because it just removes any character whose code point is less than or equal to the space character. But, there are many whitespace characters whose code point is more than that. You can check a list of all the whitespace characters in this Wikipedia article.
- Java 11 introduced strip() method to remove white spaces using the Character class API. It is recommended to use strip() method now to remove all the leading and trailing white spaces.
Table of Contents
Java String trim() Method Examples
Let’s look at some examples of using trim() method.
1. Removing Leading and Trailing Spaces from the String
jshell> String str = " Hello Java ";
str ==> " Hello Java "
jshell> String trimStr = str.trim();
trimStr ==> "Hello Java"
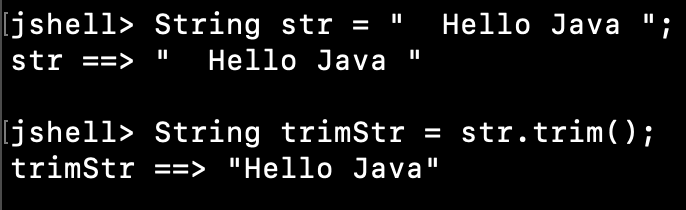
2. Trimming Tabs from the String
Let’s add some tabs to the string and trim them.
jshell> String str = "\t\t Hello Java \t";
str ==> "\t\t Hello Java \t"
jshell> String trimStr = str.trim();
trimStr ==> "Hello Java"
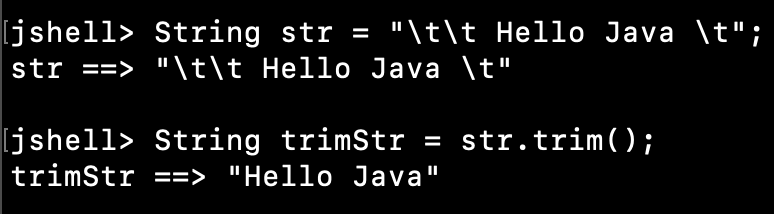
3. Removing New Line Characters from the String
jshell> String str = "\n\nHello Java \r\n";
str ==> "\n\nHello Java \r\n"
jshell> String trimStr = str.trim();
trimStr ==> "Hello Java"
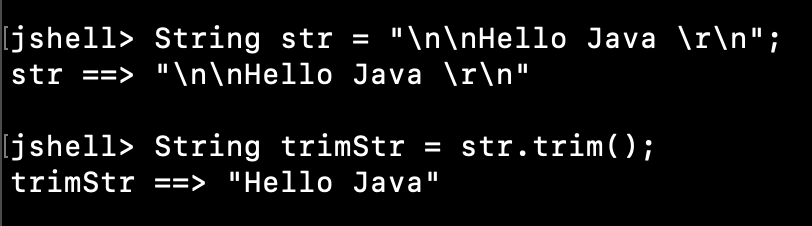
4. Empty and Strings with No Leading and Trailing White Spaces
Some miscellaneous examples with an empty string, string with white spaces only, and string with no leading and trailing white spaces.
jshell> String emptyStr = "";
emptyStr ==> ""
jshell> String emptyStrTrim = emptyStr.trim();
emptyStrTrim ==> ""
jshell> emptyStr == emptyStrTrim
$131 ==> true
jshell> String whitespacesStr = " \t\t\n\r ";
whitespacesStr ==> " \t\t\n\r "
jshell> String whitespacesStrTrim = whitespacesStr.trim();
whitespacesStrTrim ==> ""
jshell> String fruit = "Apple";
fruit ==> "Apple"
jshell> String fruitTrim = fruit.trim();
fruitTrim ==> "Apple"
jshell> fruit == fruitTrim;
$136 ==> true
Notice that when the trim() method doesn’t find any leading and trailing white spaces, the reference to this string is returned.
Recommended Read: Java String Pool.
trim() vs strip()
- The trim() method just checks the Unicode code point and remove them if it’s less than U+0020. The strip() method uses Character and Unicode API to check for white space characters.
- The trim() method is faster than the strip() method because of its simplicity.
- You can use trim() method if the string contains usual white space characters – space, tabs, newline, a carriage return.
- The recommended method is strip() to remove all the leading and trailing white space characters from the string. It uses standard APIs to check for white spaces and more reliable.
Let’s look at an example where the string has special white space characters whose Unicode code point is greater than U+0020.
jshell> String str = "\n\nHello Java \u2000\r\n"
str ==> "\n\nHello Java \r\n"
jshell> String trimStr = str.trim();
trimStr ==> "Hello Java "
jshell> String stripStr = str.strip();
stripStr ==> "Hello Java"
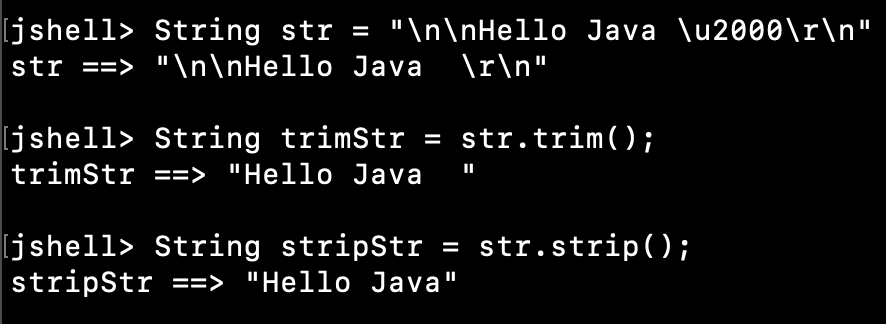
Notice that strip() method removed “\u2000” (en quad) white space character which was skipped by the trim() method.
Conclusion
Java String trim() method removes leading and trailing white spaces. However, it doesn’t use any standard APIs to check if a character is whitespace or not. It simply removes any character whose Unicode code point is less than or equal to U+0020. That’s why the strip() method was introduced in Java 11. If you are using Java 11 or higher versions, please use the strip() method.