Java String equalsIgnoreCase() method compares this string with the argument string without case consideration. This method returns true if both the strings have the same character sequence, ignoring their case.
Table of Contents
String equalsIgnoreCase() Method Implementation
The equalsIgnoreCase() method is implemented like this.
public boolean equalsIgnoreCase(String anotherString) {
return (this == anotherString) ? true
: (anotherString != null)
&& (anotherString.length() == length())
&& regionMatches(true, 0, anotherString, 0, length());
}
The Logic for Characters Comparison Ignoring Case
The two characters c1 and c2 are considered equal ignoring case when one of the following condition is true.
- c1 == c2
- Character.toLowerCase(Character.toUpperCase(c1)) == Character.toLowerCase(Character.toUpperCase(c2))
If the first condition is true, it means both the characters are the same.
If the second condition is true, it means their case was different.
Why we can’t have the second condition as Character.
toUpperCase
(c1) == Character.
toUpperCase
(c2)
?
It’s because there are situations where different Unicode characters have the same uppercase or lowercase value. So using both uppercase and lowercase methods make sure that both the characters are the same. You can read more about it at this StackOverflow Question.
Java String equalsIgnoreCase() Example
String s1 = "String";
String s2 = "string";
String s3 = "STRING";
String s4 = "Python";
System.out.println(s1.equalsIgnoreCase(s2)); // true
System.out.println(s1.equalsIgnoreCase(s3)); // true
System.out.println(s1.equalsIgnoreCase(s4)); // false
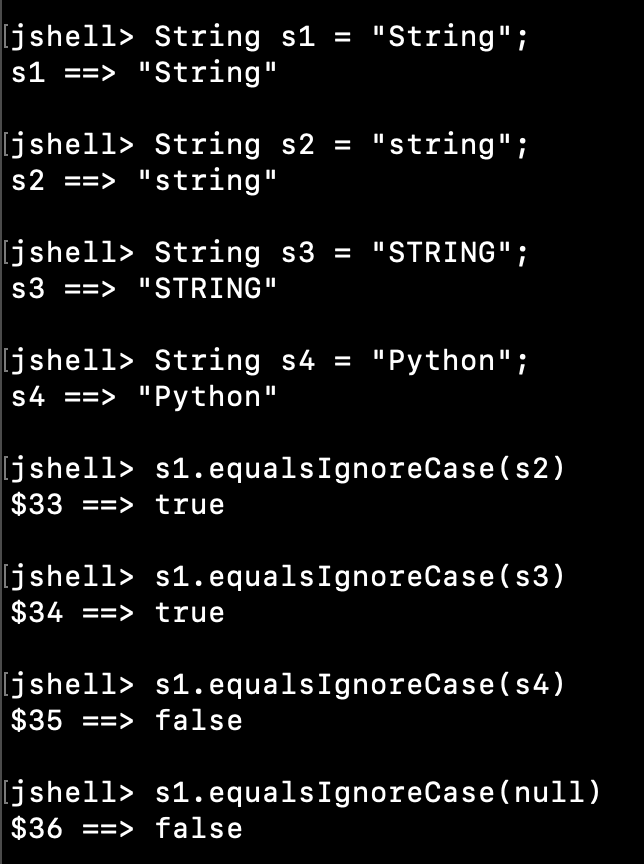
Best Way to use equalsIgnoreCase() Method
Let’s look at a method where we are using equalsIgnoreCase() method.
public void process(String language) {
if (language.equalsIgnoreCase("Java")) {
// processing for Java
} else if (language.equalsIgnoreCase("Python")) {
// processing for Python
} else {
// throw UnsupportedLanguageException
}
}
It looks to be properly implemented and very simple. But, there is one small problem. If someone passes language value as null
, this method will throw NullPointerException
.
We can fix this by adding a null check in the code, something like this:
public void process(String language) {
if (language == null){
// throw UnsupportedLanguageException
}
// further code
}
Or you can use the equalsIgnoreCase() cleverly to avoid NullPointerException completely.
public void process(String language) {
if ("Java".equalsIgnoreCase(language)) {
// processing for Java
} else if ("Python".equalsIgnoreCase(language)) {
// processing for Python
} else {
// default processing
}
}
Conclusion
Java String equalsIgnoreCase() method is useful for case insensitive string comparison. However, this method doesn’t take locale into account, so the result might be not proper for some locales.