- The String join() method is used to join multiple strings, arrays, or Iterable elements to form a new string.
- We can specify the delimiter string to be used when joining the elements.
- Java String join() methods are static.
- These methods got added in String class in Java 1.8 release.
- The join() method throws NullPointerException if the delimiter is null or the elements to join is null.
- The common use case of join() method is to create a CSV string from a collection of strings.
- This method internally uses StringJoiner class.
Table of Contents
String join() Methods
There are two variants of String join() method.
- join(CharSequence delimiter, CharSequence… elements): joins CharSequence elements with the given delimiter to form a new string. The acceptable arguments are String, StringBuffer, and StringBuilder. We can use this method to join string array elements.
- join(CharSequence delimiter, Iterable<? extends CharSequence> elements): This method is useful when we have to join iterable elements. Some of the common Iterables are – List, Set, Queue, and Stack.
Java String join() Method Examples
Let’s look at some examples of join() method usage.
1. Joining Multiple Strings
The join() method accepts variable arguments, so we can easily use it to join multiple strings.
jshell> String.join(",", "A", "B", "C");
$1 ==> "A,B,C"
2. Joining String Array Elements
jshell> String[] fruits = { "Apple", "Banana", "Orange" };
fruits ==> String[3] { "Apple", "Banana", "Orange" }
jshell> String.join(",", fruits);
$3 ==> "Apple,Banana,Orange"

3. Joining Multiple Array Elements
We can also join multiple array elements to form a new string using the join() method.
String[] capitalLetters = {"A","B","C"};
String[] smallLetters = {"a","b","c"};
String result = String.join("|",
String.join("|", capitalLetters),
String.join("|", smallLetters));
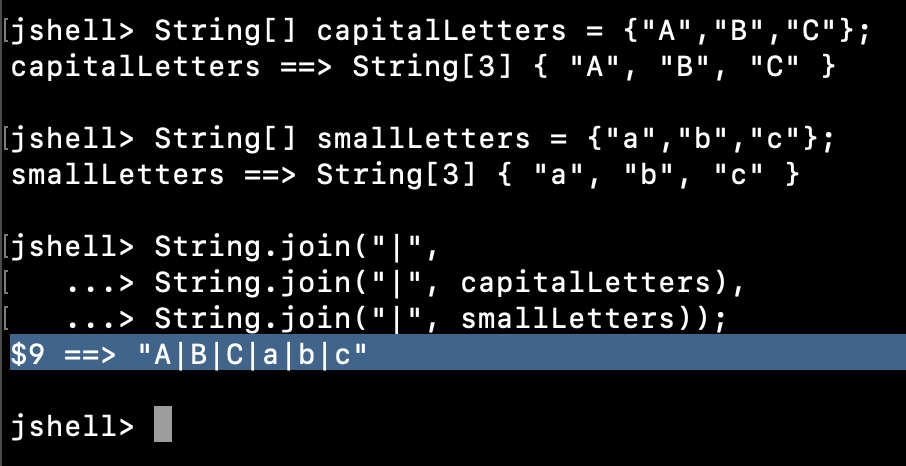
4. Joining StringBuffer, StringBuilder and Strings
The join() method accepts CharSequence objects. So we can pass StringBuilder, StringBuffer, and Strings as an argument for this method.
jshell> StringBuffer sb1 = new StringBuffer("ABC");
sb1 ==> ABC
jshell> StringBuffer sb2 = new StringBuffer("123");
sb2 ==> 123
jshell> StringBuilder sb3 = new StringBuilder("XYZ");
sb3 ==> XYZ
jshell> StringBuilder sb4 = new StringBuilder("987");
sb4 ==> 987
jshell> String result = String.join(" ", sb1, sb2, sb3, sb4);
result ==> "ABC 123 XYZ 987"
5. Joining List Elements
jshell> List<String> vowelsList = List.of("a", "e", "i", "o", "u");
vowelsList ==> [a, e, i, o, u]
jshell> String vowels = String.join(" ", vowelsList);
vowels ==> "a e i o u"
6. Joining Set Elements
package net.javastring.strings;
import java.util.HashSet;
import java.util.Set;
public class JavaStringJoin {
public static void main(String[] args) {
Set<CharSequence> setStrings = new HashSet<>();
setStrings.add("A");
setStrings.add(new StringBuilder("B"));
setStrings.add(new StringBuffer("C"));
String result = String.join("$$", setStrings);
System.out.println(result);
}
}
Output: A$$B$$C
7. Joining Queue Elements
package net.javastring.strings;
import java.util.PriorityQueue;
import java.util.Queue;
public class JavaStringJoin {
public static void main(String[] args) {
Queue<String> messagesQueue = new PriorityQueue<>();
messagesQueue.add("Hello");
messagesQueue.add("Hi");
messagesQueue.add("Bonjour");
String messagesCSV = String.join(",", messagesQueue);
System.out.println(messagesCSV);
}
}
Output: Bonjour,Hi,Hello
8. Joining Stack Elements
Stack<String> currencyStack = new Stack<>();
currencyStack.add("USD");
currencyStack.add("INR");
currencyStack.add("GBP");
String currenciesCSV = String.join(",", currencyStack);
System.out.println(currenciesCSV);
Output: USD,INR,GBP
Conclusion
Java String join() is a great utility method to add array elements or iterable elements to form a new string. This has removed the need to write our custom code for this functionality.