The String in java represents a stream of characters. There are many ways to convert string to character array.
Table of Contents
Java Convert String to char Array
- Manual code using for loop
- String toCharArray() method
- String getChars() method for subset
- String charAt() method
Let’s look into some examples to convert string to char array in Java.
1. Using for loop
We can write our own code to get the string characters one by one. Then populate the char array with the characters.
String s = "abc123";
char[] chrs = new char[s.length()];
for (int i = 0; i < s.length(); i++)
chrs[i] = s.charAt(i);
System.out.println(Arrays.toString(chrs));
Output:[a, b, c, 1, 2, 3]
This method is useful when you want to have some additional logic to populate the char array. For example, skipping duplicate characters or changing them to lowercase, etc.
2. toCharArray() method
This is the recommended method to convert string to char array. Let’s look at the example to convert string to char array using toCharArray() method. We will use JShell to run the code snippets.
jshell> String s = "abc123";
s ==> "abc123"
jshell> char[] chars = s.toCharArray();
chars ==> char[6] { 'a', 'b', 'c', '1', '2', '3' }
jshell> System.out.println(Arrays.toString(chars));
[a, b, c, 1, 2, 3]
jshell>
Output:
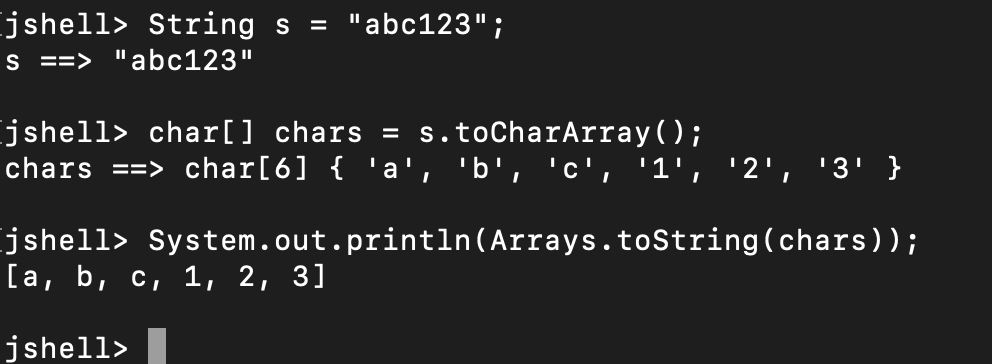
3. charAt() method
Sometimes we have to get the character at the specific index. We can use charAt() method for this.
jshell> String s = "Java";
s ==> "Java"
jshell> char c = s.charAt(3);
c ==> 'a'
4. getChars() method
This method is useful when we want to copy characters between specific indices. String getChars() method copies the characters from the string to the destination character array. The method syntax is:
public
void
getChars(
int
srcBegin,
int
srcEnd,
char
dst[],
int
dstBegin)
Let’s understand the significance of each of the method arguments.
- srcBegin: index from where the characters will be copied.
- srcEnd: index at which copying will stop. The last index to be copied will be srcEnd-1.
- dst: The destination character array. It should be initialized before calling this method.
- dstBegin: The start index in the destination array.
Let’s look at an example of getChars() method.
String s = "abc123";
char[] substringChars = new char[7];
s.getChars(1, 4, substringChars, 2);
System.out.println(Arrays.toString(substringChars));
Output: [, , b, c, 1, , ]
The string characters are copied from index 1 to 3. The characters are copied starting from 2nd index of the destination array.
If the destination array size is smaller than the required size, java.lang.StringIndexOutOfBoundsException
is thrown.
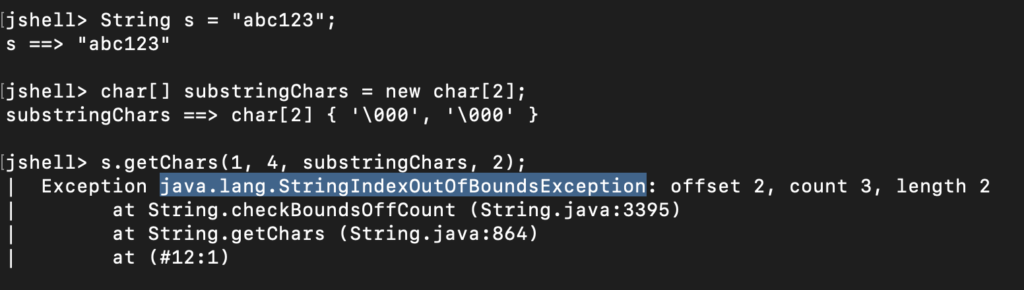
Conclusion
- String toCharArray() is the recommended method to convert string to char array.
- If you want to copy only a part of the string to a char array, use getChars() method.
- Use the charAt() method to get the char value at a specific index.
- You can write your own code to convert string to char array if there are additional rules to be applied while conversion.