Java String contentEquals() method returns true if this string has the same character sequence as the method argument.
Table of Contents
String contentEquals() Method Signatures
There are two overloaded version of contentEquals() method.
public boolean contentEquals(CharSequence cs)
: The string object content is compared with the CharSequence argument. If the character sequence is exactly the same, then this method returns true. Otherwise, this method returns false.public boolean contentEquals(StringBuffer sb)
: This method was introduced in Java 1.4 to compare the string content with the StringBuffer. The above method got introduced in Java 1.5. This method internally calls the above method. So it’s better to use the above method. I am wondering why this method is not deprecated until now.
Possible Arguments for contentEquals() Method
We can pass the following objects as an argument for the contentEquals() method.
- String
- StringBuffer
- StringBuilder
You can confirm this from the type hierarchy of the CharSequence interface.
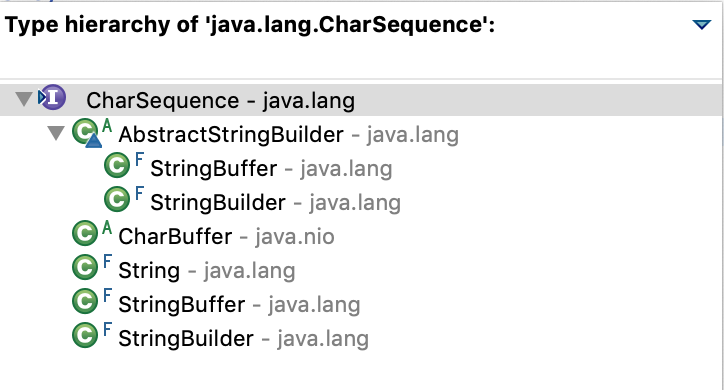
There are some abstract classes too. But the only concrete implementations provided by Java are the three classes above.
Java String contentEquals() Examples
Let’s look at some simple examples of using contentEquals() method.
1. contentEquals() with String Argument
String s1 = "Java";
System.out.println(s1.contentEquals("Java")); // true
System.out.println(s1.contentEquals("java")); // false
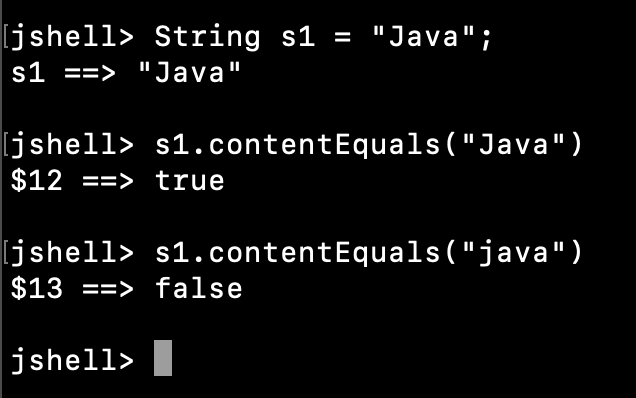
2. contentEquals() with StringBuffer Argument
StringBuffer sb = new StringBuffer();
sb.append("Java");
System.out.println(s1.contentEquals(sb)); // true
sb.append("String");
System.out.println(s1.contentEquals(sb)); // false
3. contentEquals() with StringBuilder Argument
StringBuilder sb1 = new StringBuilder("Java");
System.out.println(s1.contentEquals(sb1)); // true
sb1.append("String");
System.out.println(s1.contentEquals(sb1)); // false
Java String contentEquals() vs equals()
- Java String contentEquals() can be used with all the three implementations of CharSequence. String equals() method works with String only. The equals() method return false if the input argument is not String.
- If equals() method returns true, contentEquals() will also return true.
- If equals() returns false, contentEquals() may return true or false.
- If contentEquals() returns true, equals() may be false depending on the type of argument.
- If contentEquals() returns false, equals() will also return false.
Let’s look at an example to confirm above points.
// equals() vs contentEquals()
String a = "Java";
String b = "Java";
String c = "Python";
StringBuilder d = new StringBuilder("Java");
// equals() is true, then contentEquals() will also be true
System.out.println(a.equals(b)); // true
System.out.println(a.contentEquals(b)); // true
// equals() is false, then contentEquals() may be true or false
System.out.println(a.equals(c)); // false
System.out.println(a.contentEquals(c)); // false
System.out.println(a.equals(d)); // false
System.out.println(a.contentEquals(d)); // true
// contentEquals() is true, then equals() may be true or false
System.out.println(a.contentEquals(b)); // true
System.out.println(a.equals(b)); // true
System.out.println(a.contentEquals(d)); // true
System.out.println(a.equals(d)); // false
// contentEquals() is false, then equals() will also be false
d.append("String");
System.out.println(a.contentEquals(d)); // false
System.out.println(a.equals(d)); // false
System.out.println(a.contentEquals(c)); // false
System.out.println(a.equals(c)); // false
Conclusion
You can use contentEquals() method to compare the String with StringBuffer
or StringBuilder
. If you are comparing two strings contents, it’s better to use equals()
method.