Java String hashCode() method returns the integer hash code value of this string. String class overrides this method from Object class.
Table of Contents
How Does Java Calculates String Hash Code?
The string hash code calculation follows the below logic.
int hashcode = s[0]*31^(n-1) + s[1]*31^(n-2) + ... + s[n-1];
- Here s[i] is the character at ith index.
^
indicates exponentiation- n is the length of the string.
The hash code of an empty string is 0.
Java String hashCode() Examples
Let’s have a look at some examples of String hashCode() method.
package net.javastring.strings;
public class JavaStringHashCode {
public static void main(String[] args) {
String s1 = "Java";
String s2 = "Java";
String s3 = new String("Java");
System.out.println(s1.hashCode());
System.out.println(s2.hashCode());
System.out.println(s3.hashCode());
System.out.println("".hashCode());
}
}
Output:
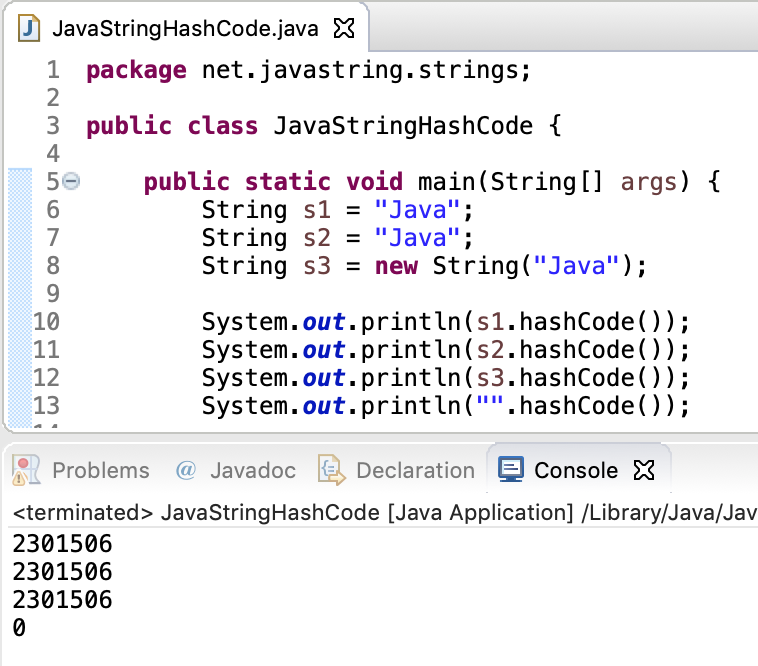
Java String hashCode() and equals() Contract
- If
equals()
istrue
for two strings, theirhashCode()
will be the same. - If two strings
hashCode()
is equal, it doesn’t mean they are equal.
The first statement will always be true because string characters are used to calculate the hash code. You can confirm this from the above java program too.
Let’s look at some code snippets to confirm the second statement.
String a = "Siblings";
String b = "Teheran";
System.out.println(a.hashCode());
System.out.println(b.hashCode());
System.out.println(a.hashCode() == b.hashCode());
System.out.println(a.equals(b));
Here is the output when the above code snippet is executed in JShell.
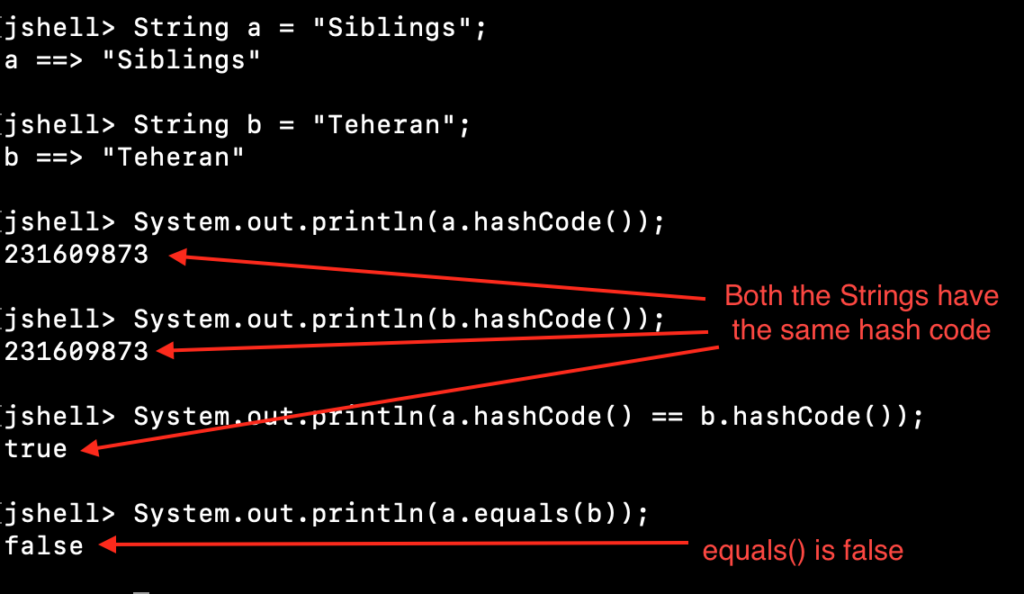
Java String hashcode Collision
When two strings have the same hashcode, it’s called a hashcode collision. There are many instances where the hash code collision will happen. For example, “Aa” and “BB” have the same hash code value 2112.
Conclusion
We shouldn’t rely on the hashCode() method to check if two strings are equal. String class overrides this function from Object class. It’s used by Java internally when String is used as Map key for get()
and put()
operations.