The simple approach to swap two strings in Java is by using a third variable. But, we can also swap two strings without using the third variable.
Swap Two Strings in Java using Third Variable
Here is the simple program to swap two strings using a third variable.
package net.javastring.strings;
public class JavaSwapStrings {
public static void main(String[] args) {
String s1 = "Apple";
String s2 = "Banana";
System.out.printf("Before Swapping s1 = %s and s2 = %s.\n", s1, s2);
String temp = s1;
s1 = s2;
s2 = temp;
System.out.printf("After Swapping s1 = %s and s2 = %s.\n", s1, s2);
}
}
Output:
Before Swapping s1 = Apple and s2 = Banana.
After Swapping s1 = Banana and s2 = Apple.
Swap Two Strings in Java without Third Variable
The idea is to use String concatenation and then substring() method to swap two strings without using any third variable.
package net.javastring.strings;
public class JavaSwapStrings {
public static void main(String[] args) {
String s1 = "Apple";
String s2 = "Banana";
System.out.println(String.format("Before Swapping s1 = %s and s2 = %s.\n", s1, s2));
s1 = s1 + s2; // s1 = "AppleBanana";
s2 = s1.substring(0, s1.length()-s2.length()); // s2 = "AppleBanana".substring(0, 11-6) = "Apple"
s1 = s1.substring(s2.length()); // s1 = "AppleBanana".substring(5) = "Banana"
System.out.println(String.format("After Swapping s1 = %s and s2 = %s.\n", s1, s2));
}
}
Output:
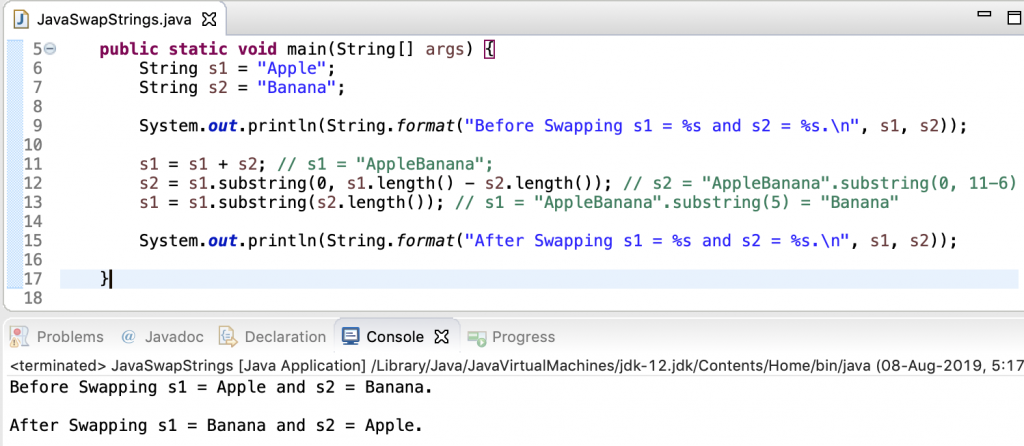
Notice the use of the String format() method. It works the same as System.out.printf()
method.