A string literal is is the sequence of characters enclosed in double-quotes. Java String literals are the preferred way to create string objects. They are string objects created in the string pool area.
Table of Contents
Creating String Literal in Java
jshell> String str = "Java";
str ==> "Java"
Where is String Literal stored in Java?
The string literals are always stored in the string pool. It’s also called a string literal pool. When a string literal is created, the pool is checked to see if there is already a string literal having the same value. If found, the reference to that string is returned. If not found, a new string is created in the pool and its reference is returned.
Which is better String Literal or String Object?
String literal is better most of the times. It saves memory and accessing them are faster than the string objects in the heap area. The string object is created using new operator when using other sources. For example, creating a string from the character array.
Java String Literal are Primitive Data Type?
No, string literals are the same as string objects. It’s a convenient and better way to create string objects for faster processing and saving memory space.
Java String Literal Features
- Java string literals are always stored in the string pool.
- String literals are an instance of String class.
- String literals are interned, read more at String intern() method.
- String literals with the same value always refer to the same string object in the string pool.
String Literals and Concatenation
We can break a long string into multiple shorter strings using the string concatenation operator (+).
String longString = "There are 7 days in a week." +
" There are 24 hours in a day." +
" There are 60 minutes in an hour.";
In this case, the string concatenation is computed at the compile-time and assigned to the string.
But, when the string concatenation happens at runtime, the strings are newly created in the heap area. Let’s confirm this behavior with a simple example.
jshell> String s1 = "A";
s1 ==> "A"
jshell> String s2 = "B";
s2 ==> "B"
jshell> "AB" == "A" + "B" // compile time concatenation
$34 ==> true
jshell> "AB" == s1 + s2 // runtime concatenation
$35 ==> false
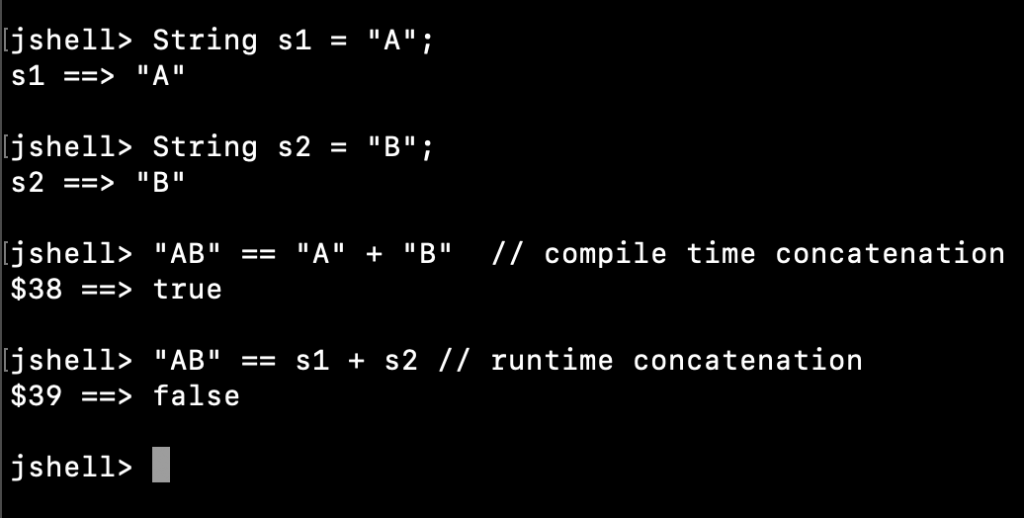
Conclusion
Java String literal are string objects created in the string pool. It’s the preferred way to create string objects. You can always call intern() method to move a string object from the heap area to the string pool and convert it to a string literal.