- Java StringBuffer class is used to manipulate string objects.
- StringBuffer is a thread-safe mutable sequence of characters.
- StringBuffer methods are synchronized, so it’s advised to use it for string manipulation in a multithreaded environment.
- Some of the common operations StringBuffer provides are – append, insert, reverse, and delete.
- StringBuilder class was introduced in Java 1.5, which is similar to StringBuffer except that it’s not thread-safe. You should use StringBuilder for string manipulation in a single threaded environment.
Table of Contents
StringBuffer Constructors
StringBuffer class has 4 constructors.
- StringBuffer(): creates an empty string buffer with a capacity of 16 characters.
- StringBuffer(int capacity): creates an empty string buffer with the specified character capacity. This is useful when you know the capacity required by the string buffer to save time in increasing the capacity.
- StringBuffer(String str): creates a string buffer having the same character as the string argument. The initial capacity of the string buffer is “length of str + 16”.
- StringBuffer(CharSequence seq): creates a string buffer with the same characters as in the CharSequence. The initial capacity of the string buffer is “length of seq + 16”.
Let’s look at some examples of creating StringBuffer object using above constructors.
package net.javastring.strings;
public class JavaStringBuffer {
public static void main(String[] args) {
StringBuffer sb = new StringBuffer();
System.out.println(sb.capacity());
System.out.println(sb.toString());
StringBuffer sb1 = new StringBuffer(1000);
System.out.println(sb1.capacity());
System.out.println(sb1.toString());
StringBuffer sb2 = new StringBuffer("Hello");
System.out.println(sb2.capacity());
System.out.println(sb2.toString());
CharSequence seq = new StringBuilder("Hello");
StringBuffer sb3 = new StringBuffer(seq);
System.out.println(sb3.capacity());
System.out.println(sb3.toString());
}
}
Output:
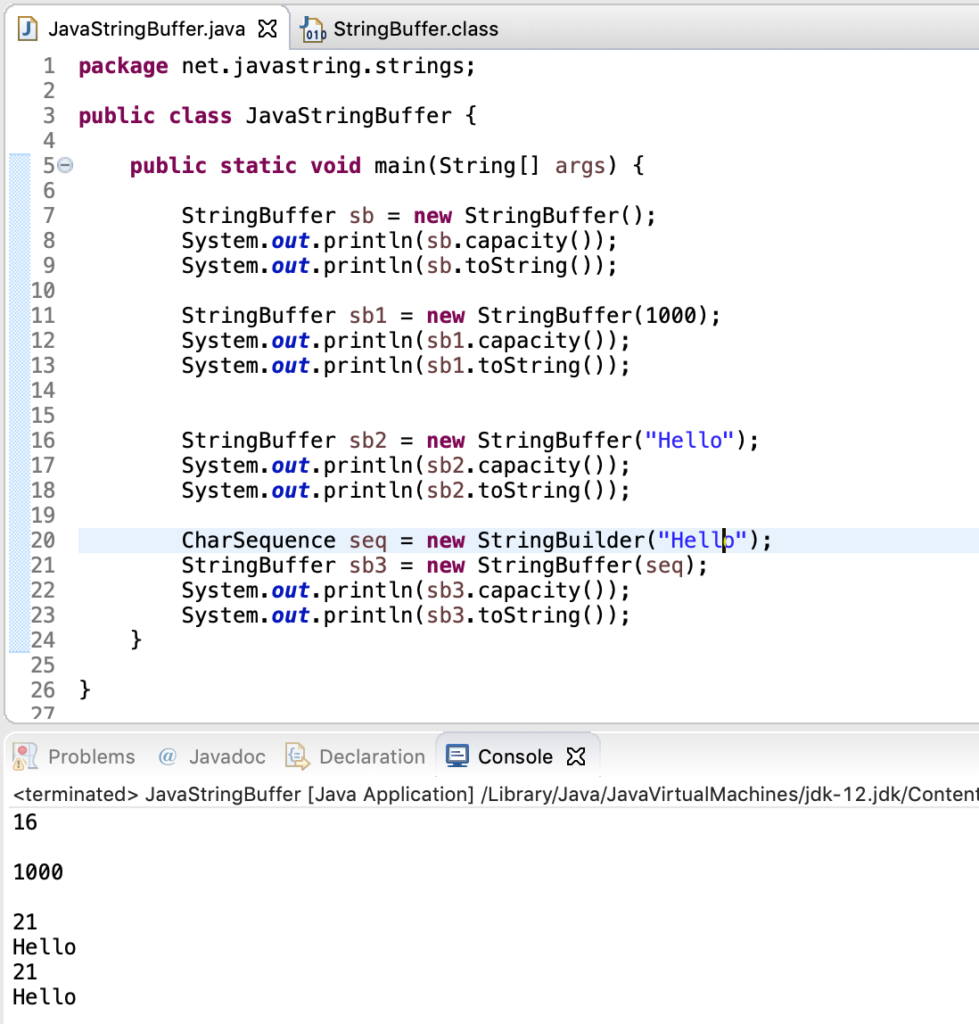
Java StringBuffer Capacity
- StringBuffer uses a byte array to store the characters.
- When we initialize the StringBuffer, the byte array is also initialized.
- The size of the byte array can be specified through the constructor.
- The default capacity of the byte array is 16.
- When we append more characters to the string buffer than the existing capacity, the byte array is reinitialized with higher capacity and the existing content.
- If you have some idea of the capacity required by the StringBuffer, use the constructor to specify the required initial capacity. It will make StringBuffer operations faster because the byte array reshuffle won’t be required.
- We can get the current capacity of StringBuffer by calling its
capacity()
method.
Java StringBuffer to String
We can call toString() method on the StringBuffer to get its string representation.
jshell> StringBuffer sb = new StringBuffer("Hello");
sb ==> Hello
jshell> String str = sb.toString();
str ==> "Hello"
Java StringBuffer Methods
StringBuffer methods can be divided into the following categories.
- append(): many overloaded methods to append primitive as well as objects to the StringBuffer. The data is always added at the end of the string buffer.
- insert(): used to insert characters at the given index. There are multiple overloaded methods for a specific type of argument.
- delete(): used to delete characters from the string buffer.
- replace(): replaces the character in the string buffer.
- reverse(): reverses the character sequence. It’s useful in reversing a string because String class doesn’t have a reverse() method.
- Miscellaneous Methods: length(), capacity(), compareTo(), trimToSize(), substring(), indexOf(), etc.
Let’s look into some of the important StringBuffer methods one by one.
1. StringBuffer append()
- There are append() methods to add primitive data types to the string buffer.
- We can append an object string representation to the string buffer by calling append(Object) method.
- When an argument is an object, its toString() method is used to get the string representation.
- There are methods to append a character array to the string buffer.
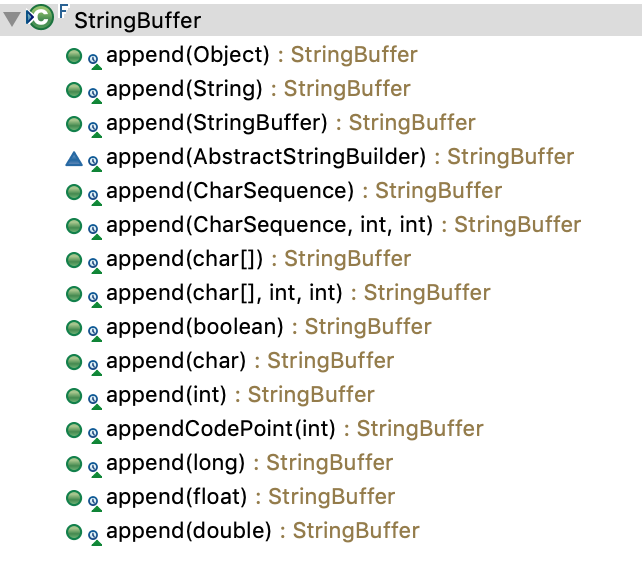
Let’s look at an example for different StringBuffer append() methods.
package net.javastring.strings;
public class JavaStringBuffer {
public static void main(String[] args) {
StringBuffer sb = new StringBuffer();
// append primitive data types
sb.append('a'); // append char
sb.append(true); // append boolean
sb.append(10); // append int
sb.append(12345L); // append long
sb.append(10.20); // append double
sb.append(10.20f); // append float
System.out.println(sb.toString());
// append char array
char[] charArray = { 'a', 'b', 'c', 'd' };
sb.append(charArray); // append complete char array
sb.append(charArray, 1, 2); // will append index 1 and 2
System.out.println(sb);
// append Objects
sb.append(new Object());
System.out.println(sb);
// append String, CharSequence
sb.append("Hello");
sb.append(new StringBuilder("Hi"));
sb.append(new StringBuilder("JavaString"), 4, 10);
System.out.println(sb);
}
}
Output:
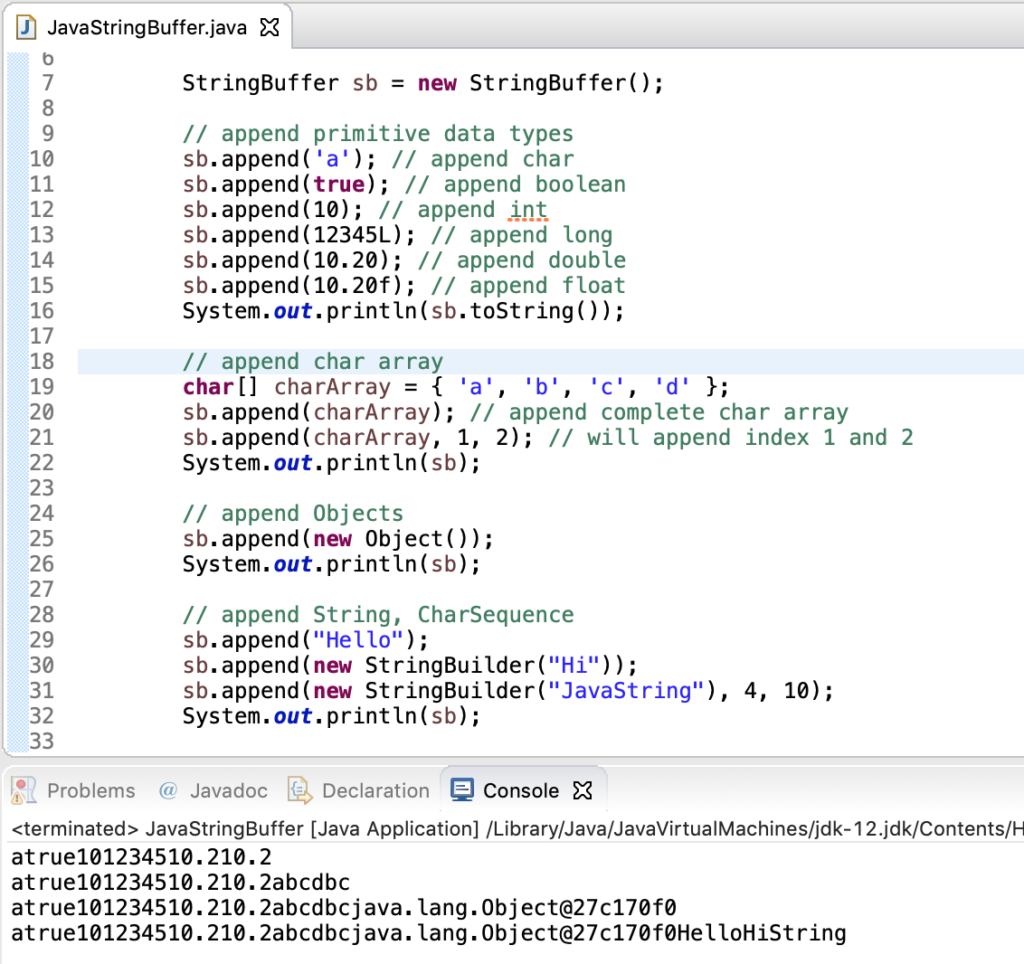
2. StringBuffer insert()
Just like append() methods, there are multiple overloaded insert() methods.
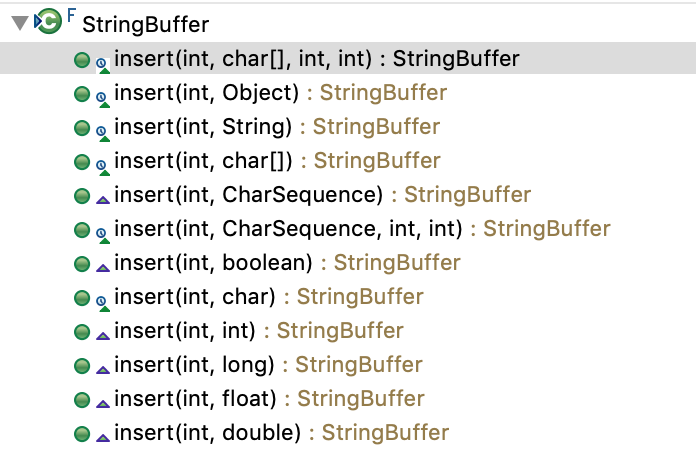
The first argument defines the index at which the second argument will be inserted. The index argument must be greater than or equal to 0, and less than or equal to the length of the string buffer. If not, the StringIndexOutOfBoundsException
will be thrown.
Let’s look at some examples of using insert() methods in a java program.
package net.javastring.strings;
public class JavaStringBuffer {
public static void main(String[] args) {
StringBuffer sb = new StringBuffer();
// insert primitive data types
sb.insert(0, 'a'); // insert char
sb.insert(1, true); // insert boolean
sb.insert(2, 10); // insert int
sb.insert(3, 12345L); // insert long
sb.insert(4, 10.20); // insert double
sb.insert(5, 10.20f); // insert float
System.out.println(sb.toString());
// insert char array
char[] charArray = { 'a', 'b', 'c', 'd' };
sb.insert(6, charArray); // insert complete char array
sb.insert(7, charArray, 1, 2); // will insert index 1 and 2
System.out.println(sb);
// insert Objects
sb.insert(8, new Object());
System.out.println(sb);
// insert String, CharSequence
sb.insert(9, "Hello");
sb.insert(10, new StringBuilder("Hi"));
sb.insert(11, new StringBuilder("JavaString"), 4, 10);
System.out.println(sb);
}
}
Output:
at11110.20.223450rue
at1111abcbcd0.20.223450rue
at1111abjava.lang.Object@27c170f0cbcd0.20.223450rue
at1111abjHHStringielloava.lang.Object@27c170f0cbcd0.20.223450rue
3. StringBuffer delete()
There are two methods in StringBuffer to delete characters.
- delete(int start, int end): deletes the sequence of character from the index start to end.
- deleteCharAt(int index): deletes the character at the specified index.
Let’s use JShell this time to show the usage of these methods.
jshell> StringBuffer sb = new StringBuffer("Hello World 2019");
sb ==> Hello World 2019
jshell> sb.delete(6, 12);
$5 ==> Hello 2019
jshell> System.out.println(sb);
Hello 2019
jshell> sb.deleteCharAt(5);
$7 ==> Hello2019
jshell> System.out.println(sb);
Hello2019
4. StringBuffer replace()
The replace method syntax is: replace(
int
start,
int
end, String str)
The start and end parameters defines the indexes in the string buffer that will be replaced with the str content. First the characters between the indexes is removed. Then the specified string is inserted at the start index.
jshell> StringBuffer sb = new StringBuffer("Hello World 2019");
sb ==> Hello World 2019
jshell> sb.replace(6, 11, "JavaString");
$10 ==> Hello JavaString 2019
jshell> System.out.println(sb);
Hello JavaString 2019
5. StringBuffer reverse()
This method reverses the character sequence. It’s very useful in reversing a string.
jshell> StringBuffer sb = new StringBuffer("Hello Java");
sb ==> Hello Java
jshell> sb.reverse();
$13 ==> avaJ olleH
6. StringBuffer Miscellaneous Methods
Let’s look at some of the miscellaneous methods in StringBuffer class.
- length(): returns the length of the string buffer.
- capacity(): returns the current capacity of the string buffer.
- ensureCapacity(int minimumCapacity): ensures that the string buffer capacity is at least equal to the specified minimum capacity.
- trimToSize(): attempts to reduce the storage usage.
- setLength(int newLength): sets the length of the string buffer.
- charAt(int index): returns the character at the given index. It’s very similar to String charAt() method.
- getChars(int srcBegin, int srcEnd, char[] dest, int dstBegin): the characters from the string buffer are copies to the character array argument.
- setCharAt(int index, char ch): sets the specified character at the given index.
- substring(int start), substring(int start, int end): returns a substring from the string buffer.
- indexOf(String str), indexOf(String str, int fromIndex), lastIndexOf(String str), lastIndexOf(String str, int fromIndex): returns the index for the first and last occurrence of the substring.
Here is an example to showcase these methods usage.
package net.javastring.strings;
import java.util.Arrays;
public class JavaStringBuffer {
public static void main(String[] args) {
StringBuffer sb = new StringBuffer("Hello Java");
System.out.println("length() = " + sb.length());
System.out.println("capacity() = " + sb.capacity());
sb.ensureCapacity(1000);
System.out.println("New capacity = " + sb.capacity());
sb.trimToSize();
System.out.println("New capacity = " + sb.capacity());
System.out.println("Current length = " + sb.length());
sb.setLength(50);
System.out.println("New capacity = " + sb.capacity());
System.out.println("New length = " + sb.length());
System.out.println("char at index 4 = " + sb.charAt(4));
char[] dest = new char[5];
sb.getChars(2, 7, dest, 0);
System.out.println("char array from string buffer = " + Arrays.toString(dest));
sb.setCharAt(5, '-');
System.out.println(sb);
System.out.println("Substring = " + sb.substring(7));
System.out.println("Substring = " + sb.substring(6, 8));
System.out.println("indexOf() = " + sb.indexOf("ll"));
System.out.println("indexOf() = " + sb.indexOf("1234"));
}
}
Output:
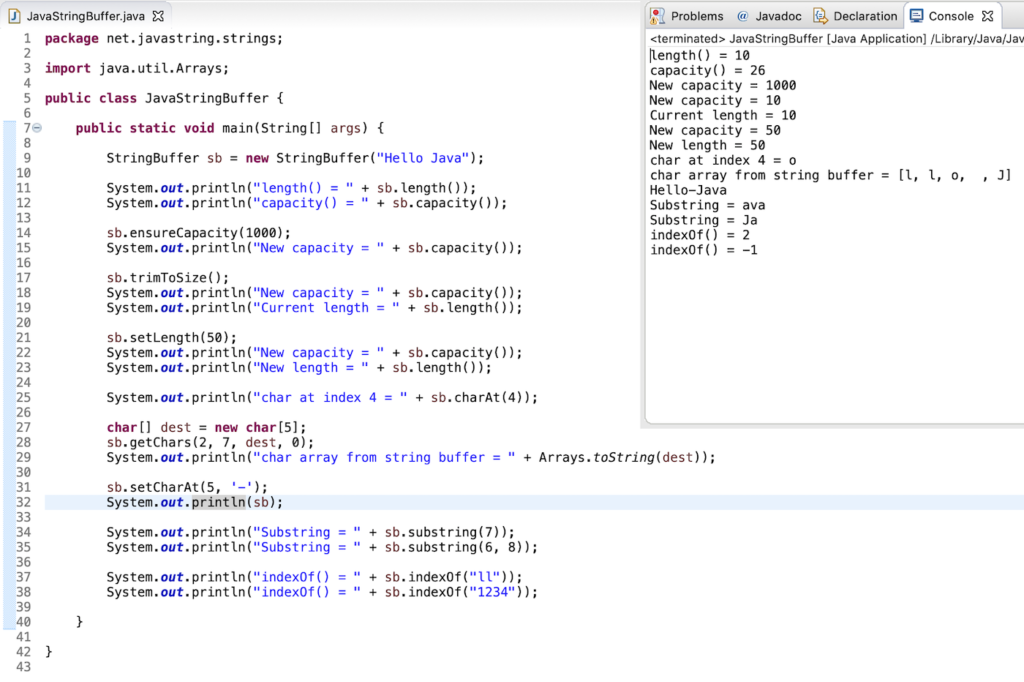
StringBuffer vs StringBuilder
- StringBuffer is thread-safe whereas StringBuilder is not thread-safe.
- StringBuffer methods are little bit slow when compared to StringBuilder methods because of synchronization.
- StringBuffer has some additional methods such as substring(), length() etc. These are not required because String class already has these methods.
- StringBuffer class is present from Java 1.0 onwards. StringBuilder is a new class introduced in Java 1.5
- StringBuffer is recommended for multi-threaded environment whereas StringBuilder is recommended for single threaded environment.
Conclusion
Java StringBuffer is a utility class for string manipulation. You should use it for string manipulation in a multi-threaded environment. For single threaded environment, use its counterpart class StringBuilder.