String is one of the most popular classes in the Java Programming language. You will find Strings everywhere in Java programs. In fact, the java main function argument itself is an array of Strings.
Table of Contents
Quick Overview of Java String Class
- Java String Class is present in
java.util
package. - A string is a sequence of characters. But, it’s not a primitive data type but an object.
- String object is immutable in Java. So once created, we can’t change it.
- String has a shortcut way to create its objects using double quotes. These string objects are also called string literals.
- String is the only java class that supports operator overloading. We can use the ‘+’ operator to concatenate two strings.
- Java store string objects in a specific pre-defined area. String pool is the part of java heap space to store string literals.
- String is serializable in nature and implements
Serializable
interface. - Other interfaces implemented by String class are Comparable, CharSequence, Constable, and ConstantDesc. The Constable and ConstantDesc interfaces are part of Java 12 Constants API.
Different Ways to Create String in Java
There are two ways to create string objects in java program.
- Using Double Quotes: A shortcut and special way to create strings. This is the easiest and preferred way to create a String object. The string object is created in the String Pool. For example,
String s1 = "Hello";
- Using new operator: We can also use
new
operator too for creating a string object. The string object is stored in the heap space and doesn’t take advantage of the string pool. For example,String s2 = new String("Hello");
Below image illustrates the process of creating strings using both the above methods.
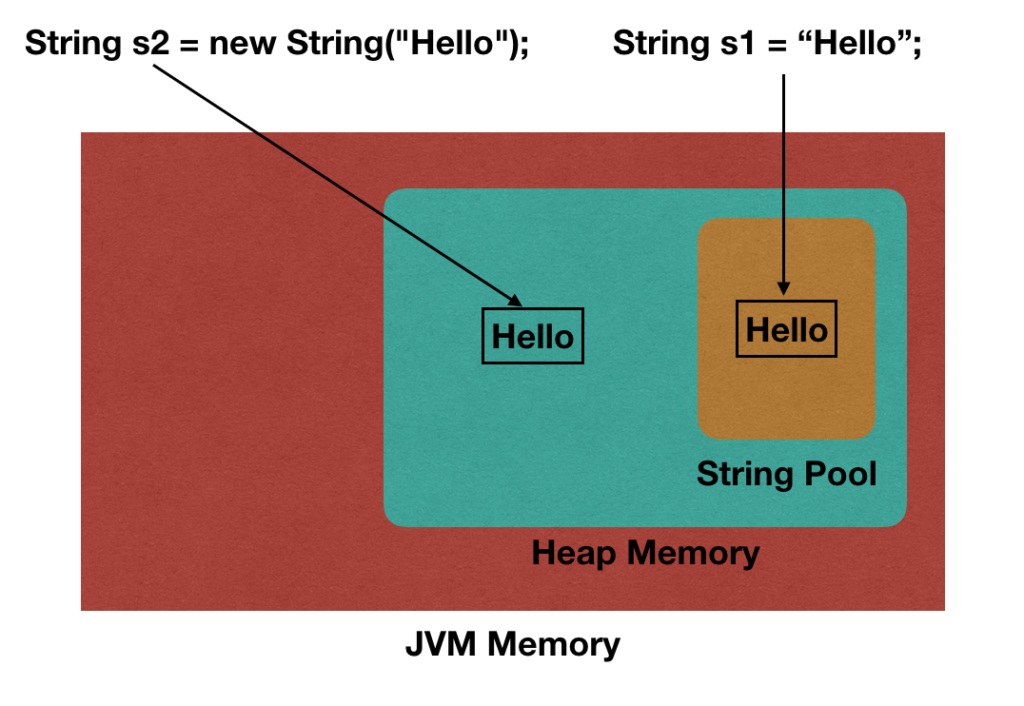
Important Methods of String Class
Let’s look at some of the important methods of String class. Some of these methods are overloaded to handle different scenarios with the arguments.
length()
: Returns the length of the string object.charAt(int index)
: Returns the character value at the given index.toCharArray()
: this method creates a character array from this string.getBytes(String charsetName)
: used to create a byte array from this string.equals(Object anObject)
: used to compare this string with another object.equalsIgnoreCase(String anotherString)
: used to compare this string with another string case-insensitively.compareTo(String anotherString), compareToIgnoreCase(String str)
: compares this string to another string lexicographically. The first one being case-sensitive and the second one performs case insensitive comparison.startsWith(String prefix)
: returns true if this string starts with the given string.endsWith(String suffix)
: returns true if this string ends with the given string.substring(int beginIndex, int endIndex)
: returns a substring of this string.concat(String str)
: Concatenates the given string to the end of this string and return it.replace(char oldChar, char newChar)
: returns a new string after replacing oldChar with the newChar.matches(String regex)
: checks whether this string matches the given regular expression.split(String regex)
: splits this string into a string array using the regular expression argument.join(CharSequence delimiter, CharSequence... elements)
: A utility method to join many strings into a new string with the specified delimiter. We can use this method to create a CSV record from the array of strings.toLowerCase(), toUpperCase()
: used to get the lowercase and uppercase version of this string.trim()
: used to remove leading and trailing whitespaces from this string.strip(), stripLeading(), stripTrailing()
: returns new string after stripping white spaces from this string. If you are confused what’s the difference betweenstrip()
andtrim()
– there are none. Both of them perform the same task butstrip()
method is more readable. It goes in line with similar methods in other programming languages.isBlank()
: returns true if the string is empty or contains only white spaces.lines()
: introduced in Java 11, returns a stream of lines from this string.indent(int n)
: introduced in Java 12, returns an indented string based on the argument value.transform(Function<? super String, ? extends R> f)
: introduced in Java 12 to apply a function to this string. The function should accept a single string argument and return R.format(String format, Object... args)
: returns a formatted string using the specified format and arguments.valueOf(Object obj)
: returns the string representation of the given object. There are overloaded versions to work with primitive data types, arrays, and objects.intern()
: returns the string from the string pool.repeat(int count)
: returns a new string after concatenating this string specified times.describeConstable(), resolveConstantDesc(MethodHandles.Lookup lookup)
: implemented for Java 12 Constants API.
Benefits of String Immutability
- String Pool is possible because String is immutable. Hence saving memory and fast performance.
- More secure since we can’t change the value of string object.
- Thread safety while working with strings in a multi-threaded environment.
- Class loading is more secure since the string value passed as an argument to load a class can’t be changed.
Quick Word on StringBuffer and StringBuilder
String immutability provides a lot of benefits. But, when we have to manipulate strings, it causes a lot of memory and inefficient operations.
That’s why there are two classes for string manipulation – StringBuffer and StringBuilder. Both of them provide similar operations. But, StringBuffer is synchronized and StringBuilder is not.