- Java String array is used to store a fixed number of string objects.
- String arrays are used a lot in Java programs. Even the Java main method parameter is a string array.
- The common use cases are – read CSV data into a string array, read text file lines into a string array.
Table of Contents
How to Declare String Array in Java?
There are two ways to declare string array in Java.
- Declaration without size.
- Declaring with array size
String[] strArray;
String[] strArray1 = new String[5];
Few points to note about the above ways of string array declaration:
- When string array is declared without size, its value is null.
- When we declare a string array with size, the array is also initialized with null values.
- We can also declare a string array as
String strArray3[]
but the above approach is recommended because it’s following convention and follows the best practice.
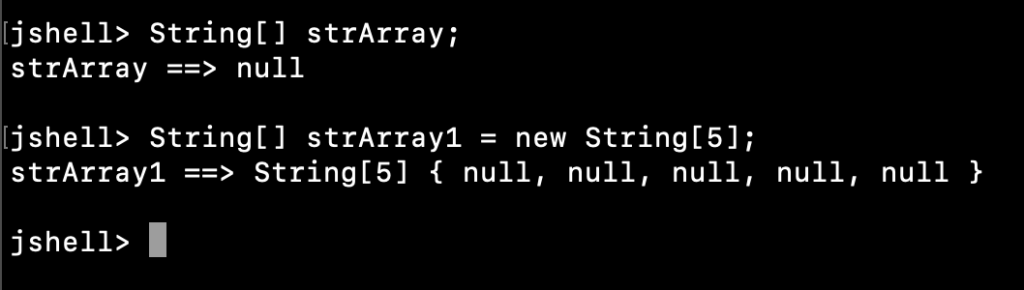
How to Initialize String Array in Java?
There are two ways to initialize a string array.
- Declaration and Initialization at the same time. It’s also called inline initialization.
- Declaring the string array and then populate the values one by one.
// inline declaration and initialization
String[] fruits = new String[] { "Apple", "Banana", "Guava" };
// shortcut method to declare and initialize at the same time
String[] cities = { "Cupertino", "Bangalore" };
// first declare and then populate values one by one
String companies[] = new String[3];
companies[0] = "Apple";
companies[1] = "Google";
companies[2] = "Microsoft";
How to check if two String Arrays are Equal?
We use equals() method to check if two objects are equal or not. Let’s see what happens with the equals() method when we have two string arrays with the same content.
jshell> String[] a1 = {"1", "2"}
a1 ==> String[2] { "1", "2" }
jshell> String[] a2 = {"1", "2"}
a2 ==> String[2] { "1", "2" }
jshell> a1.equals(a2)
$5 ==> false
jshell>
The reason for the output “false” is that string array is an object. And Object class implements equals() method like this:
public boolean equals(Object obj) {
return (this == obj);
}
Since both the arrays are referring to different objects, the output is false.
So, how to compare two string arrays for equality?
- We can use Arrays.toString() method to convert string array to string. Then use the equals() method to check equality. This method will make sure that both the arrays have same strings at the same positions.
jshell> Arrays.toString(a1).equals(Arrays.toString(a2))
$6 ==> true
Iterating over Java String Array
We can iterate over the elements of string array using two ways.
- Using for loop
- Using Java 8 for each loop
String[] fruits = new String[] { "Apple", "Banana", "Guava" };
// recommended for Java 8 or higher version
for (String fruit : fruits) {
System.out.println(fruit);
}
// old approach, for below java 8 versions
for (int i=0; i< fruits.length; i++) {
System.out.println(fruits[i]);
}
How to search a String in the String Array?
We can use for loop to search for a string in the string array. Here is a simple program to find the indexes of the string in a string array.
package net.javastring.strings;
import java.util.ArrayList;
import java.util.List;
public class JavaStringArray {
public static void main(String[] args) {
String[] vowels = new String[] { "A", "E", "I", "O", "U", "O", "E" };
System.out.println(findFirstIndex("E", vowels));
System.out.println(findAllIndexes("E", vowels));
}
public static int findFirstIndex(String str, String[] array) {
for (int i = 0; i < array.length; i++) {
if (str.equals(array[i]))
return i;
}
return -1;
}
public static List<Integer> findAllIndexes(String str, String[] array) {
List<Integer> indexesList = new ArrayList<>();
for (int i = 0; i < array.length; i++) {
if (str.equals(array[i]))
indexesList.add(i);
}
return indexesList;
}
}
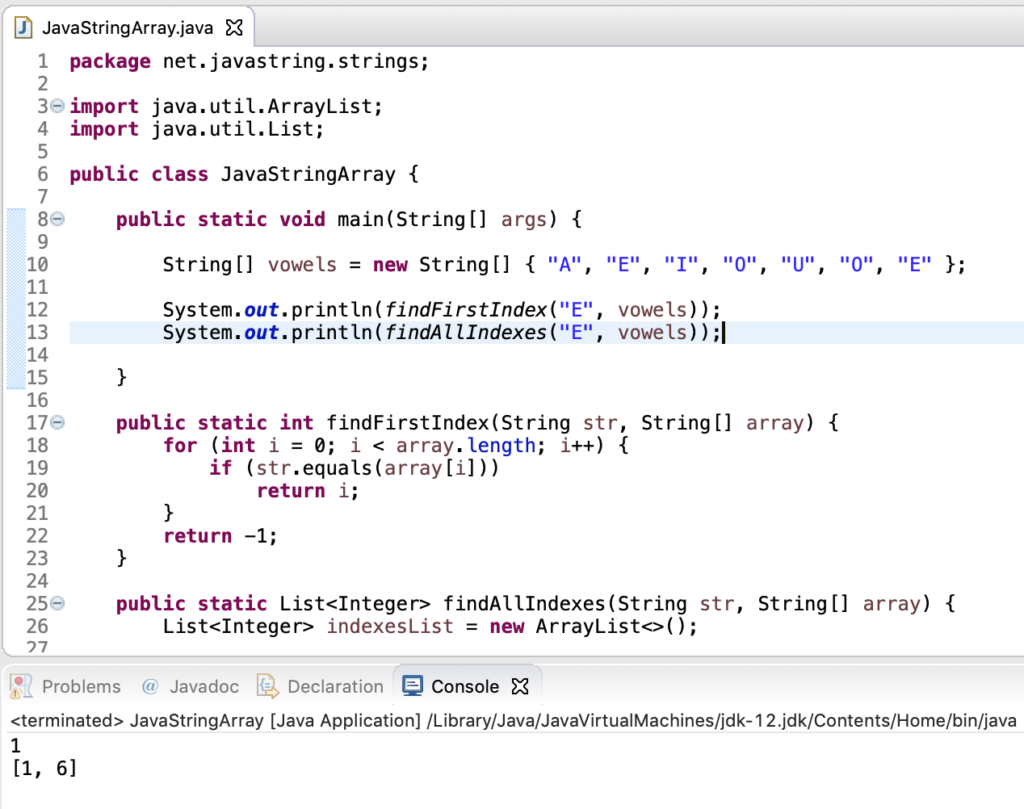
Java String Array Length
We can use length attribute of the array to find the length of the string array.
jshell> String[] vowels = new String[] { "A", "E", "I", "O", "U"}
vowels ==> String[5] { "A", "E", "I", "O", "U" }
jshell> vowels.length
$8 ==> 5
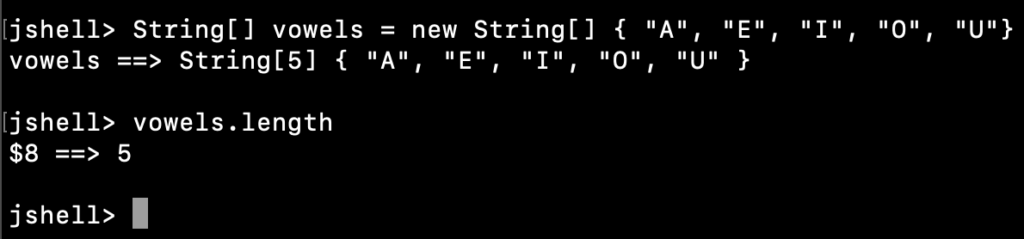
Java String Array to String
We can convert string array to string using Arrays.toString() method.
jshell> String[] vowels = new String[] { "A", "E", "I", "O", "U"}
vowels ==> String[5] { "A", "E", "I", "O", "U" }
jshell> Arrays.toString(vowels);
$10 ==> "[A, E, I, O, U]"
jshell> System.out.println(Arrays.toString(vowels));
[A, E, I, O, U]
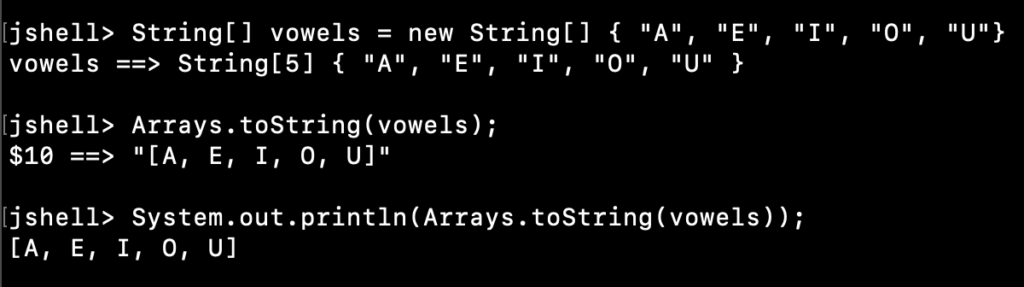
Did you thought what happens when we try to print the string array directly?
Let’s see with a simple example.
jshell> String[] chars = {"A","B"}
chars ==> String[2] { "A", "B" }
jshell> System.out.println(chars)
[Ljava.lang.String;@28c97a5
The reason for the output is that the Object class toString() method is used to get the string representation of the array. The implementation is like this:
public String toString() {
return getClass().getName() + "@" + Integer.toHexString(hashCode());
}
Now it’s clear why the output is of no use to us. Always use Arrays.toString() method to convert an array to its string representation.
Java String Array to List
We can use Arrays.asList() method to convert string array to the list of string.
jshell> String[] countries = new String[] { "USA", "UK", "INDIA"};
countries ==> String[3] { "USA", "UK", "INDIA" }
jshell> List<String> countriesList = Arrays.asList(countries);
countriesList ==> [USA, UK, INDIA]

Sorting a String Array
We can use Arrays.sort() or Arrays.parallelSort() method to sort a string array.
jshell> String[] countries = new String[] { "USA", "UK", "INDIA" };
countries ==> String[3] { "USA", "UK", "INDIA" }
jshell> Arrays.parallelSort(countries);
jshell> System.out.println(Arrays.toString(countries));
[INDIA, UK, USA]
The strings are sorted in their natural order i.e. lexicographically. We can pass a Comparator to define our own sorting logic. Here is a simple example to sort the string array in reverse order.
String[] vowels = new String[] { "A", "E", "I", "O", "U" };
Arrays.parallelSort(vowels, (String s1, String s2) -> -s1.compareTo(s2));
System.out.println(Arrays.toString(vowels));
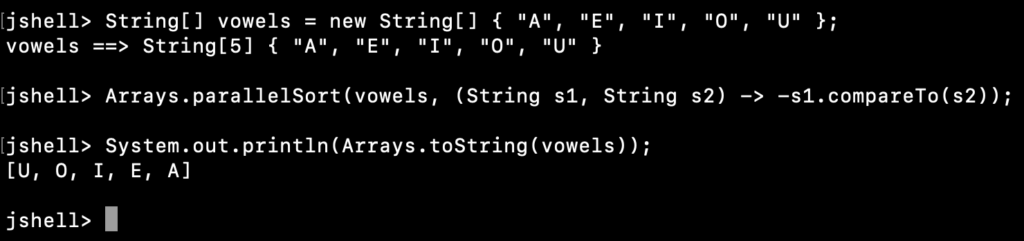
Converting String to String Array
We can use the String class split() method to convert a string into a string array. One of the popular use cases is to convert a CSV string to the string array.
jshell> String csvData = "1,2,3,4,5";
csvData ==> "1,2,3,4,5"
jshell> String[] datas = csvData.split(",");
datas ==> String[5] { "1", "2", "3", "4", "5" }
jshell> System.out.println(Arrays.deepToString(datas));
[1, 2, 3, 4, 5]