Java String equals() method compares this string to another object.
Table of Contents
Java String equals() Signature
String equals() method signature is:
public boolean equals(Object anObject)
The result is true
if the argument is String having the same value as this string. For all other cases, it will return false
. If we pass null
argument, then the method will return false
.
Why equals() Method Parameter is Not String?
It’s an interesting question. It makes prefect sense to have the method parameter as String because we are checking equality between two string objects.
So why this method argument is Object?
It’s because String equals() method is overriding Object class equals() method. You can confirm this by looking at the equals() method java documentation too.
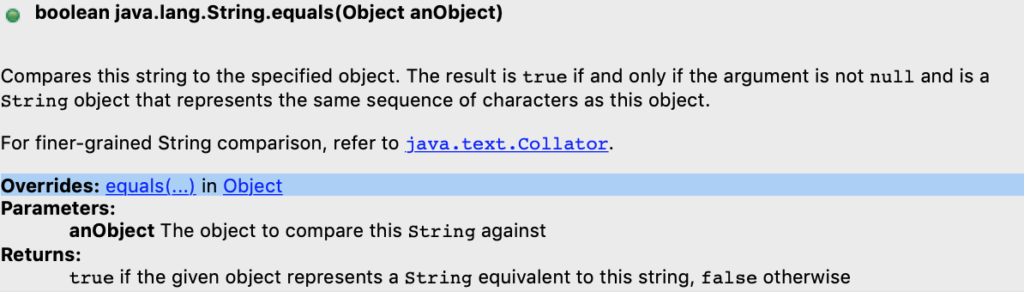
Important Points about String equals() Method
- Java String
equals()
method overrides the Object class equals() method. - If you want to check two strings for equality, you should always use equals() method.
- String equals() method doesn’t throw any exception. It always returns a boolean result.
- If you are looking to check equality ignoring case, then use
equalsIgnoreCase()
method.
Java String equals() Method Example
Let’s look at a simple example of String equals() method.
package net.javastring.strings;
public class JavaStringEquals {
public static void main(String[] args) {
String s1 = "abc";
String s2 = "abc";
System.out.println("s1 is equal to s2? " + (s1.equals(s2)));
}
}
Java String equals() and equalsIgnoreCase() Example
Let’s look at another example where we will accept two strings from user input. Then we will check their equality using equals() and equalsIgnoreCase() methods.
package net.javastring.strings;
import java.util.Scanner;
public class JavaStringEquals {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("Enter The First String:");
String str1 = sc.nextLine();
System.out.println("Enter The Second String:");
String str2 = sc.nextLine();
sc.close();
System.out.println("Both the input Strings are Equal? = " + str1.equals(str2));
System.out.println("Both the input Strings are Case Insensitive Equal? = " + str1.equalsIgnoreCase(str2));
}
}
Output:
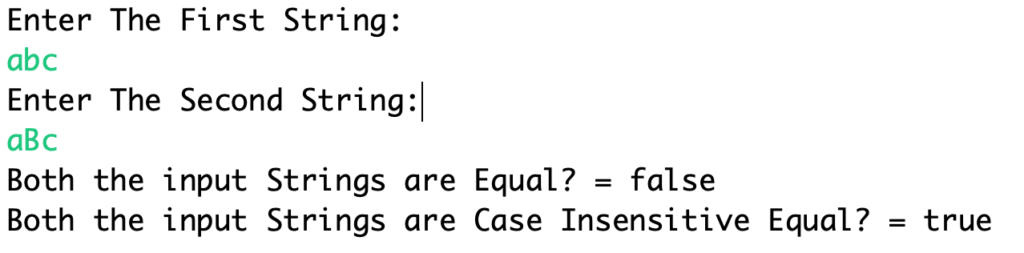