Java String split() method returns a String array. It accepts string argument and treats it as regular expression. Then the string is split around the matches found.
Table of Contents
String split() Method Syntax
There are two overloaded split() methods.
public String[] split(String regex, int limit)
: The limit argument defines the result string array size. If the limit is 0 or negative then all possible splits are part of the result string array.public String[] split(String regex)
: This is a shortcut method to split the string into maximum possible elements. This method callssplit(regex, 0)
method.
Important Points About String split() Method
- The result string array contains the strings in the order of their appearance in this string.
- If there are no matches, then the result string array contains a single string. The string element has the same value as this string.
- We can use split() method to convert CSV data into a string array.
- Trailing empty strings are not included in the result. For example,
"A1B2C3D4E5".split("[0-9]")
will result in the string array[A, B, C, D, E]
.
Java String split() Examples
Let’s look at some simple examples of split() method.
package net.javastring.strings;
import java.util.Arrays;
public class JavaStringSplit {
public static void main(String[] args) {
String s = "Hello World 2019";
String[] words = s.split(" ");
System.out.println(Arrays.toString(words));
String s1 = "A1B2C3D4E5";
String[] characters = s1.split("[0-9]");
System.out.println(Arrays.toString(characters));
String[] twoWordsArray = s.split(" ", 2);
System.out.println(Arrays.toString(twoWordsArray));
System.out.println(Arrays.toString(s.split("X", 2)));
String s2 = "A1B2C3D ";
System.out.println(Arrays.toString(s2.split("[0-9]")));
}
}
Output:
[Hello, World, 2019]
[A, B, C, D, E]
[Hello, World 2019]
[Hello World 2019]
[A, B, C, D ]
String split() Examples using JShell
We can run some examples using jshell too.
jshell> "Hello World 2019".split(" ");
$34 ==> String[3] { "Hello", "World", "2019" }
jshell> "A1B2C3D4E5".split("[0-9]");
$35 ==> String[5] { "A", "B", "C", "D", "E" }
jshell> "Hello World 2019".split(" ", 2);
$36 ==> String[2] { "Hello", "World 2019" }
jshell> " A1B2C3D ".split("[0-9]");
$37 ==> String[4] { " A", "B", "C", "D " }
jshell>
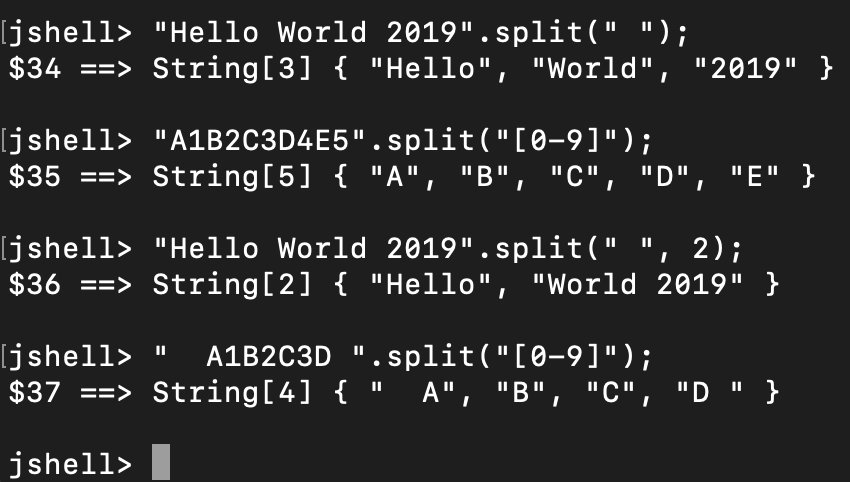