Java String format() method returns a formatted string using the given format and the arguments. It’s inspired by the C language sprintf() method.
Table of Contents
String format() Methods
There are two variants of the String format() method. Both of these methods are static.
- format(String format, Object… args): This method uses the system default locale for the formatting.
- format(Locale l, String format, Object… args): This method uses the given locale for the formatting.
These methods throw IllegalFormatException
if the format has invalid specifiers, insufficient, or wrong type of arguments.
String format() Method and Formatter Class
The java.util.Formatter class provides support for creating a formatted string. If you look at the String format() method implementation, they internally use Formatter class.
public static String format(String format, Object... args) {
return new Formatter().format(format, args).toString();
}
public static String format(Locale l, String format, Object... args) {
return new Formatter(l).format(format, args).toString();
}
We can use String.format() as well as Formatter.format() method. The result will be the same.
Why String format() method is not popular?
Let’s have a look at a simple use case of String format() method.
jshell> String.format("My name is %s","Pankaj")
$68 ==> "My name is Pankaj"
We can achieve the same result using String Concatenation.
jshell> "My name is " + "Pankaj"
$69 ==> "My name is Pankaj"
Using string concatenation is easy because we don’t need to worry about the conversion specifiers. And most of the times, the formatting requirements are simple. That’s why the String format() method is not very popular.
When to use String format() Method
We can use string concatenation to create a formatted string. But, in some cases, the format() method is preferred.
- When we have to format a large number of strings. String concatenation is costly when compared to format() method.
- If the formatted string is long, it can cause readability issues. We can use format() method in this case.
- String format() method provides special conversion logic that is not possible in string concatenation. Some of them are – changing the base of the number, date and time conversion, specific width, and precision, etc.
- When the string format has to be read from a property file, format() method benefits are clearly visible.
- String format() method provides locale support, which is missing in string concatenation.
How to print formatted String to Console?
Sometimes we have to print the formatted string to the console. There are two methods in System class for this:
- System.out.printf()
- System.err.printf()
Both of these methods internally use Formatter class to create the formatted string. So System.
out
.print(String.
format
(format, args))
is the same as System.
out
.printf(format, args)
.
Java String format() Method Examples
Let’s look at some common conversion specifiers of format() method.
1. Floating Point Number to Hexadecimal Format (%a, %A)
jshell> String.format("Hex is %a",10.55);
$70 ==> "Hex is 0x1.519999999999ap3"
jshell> String.format("Hex is %A",10.55);
$71 ==> "Hex is 0X1.519999999999AP3"
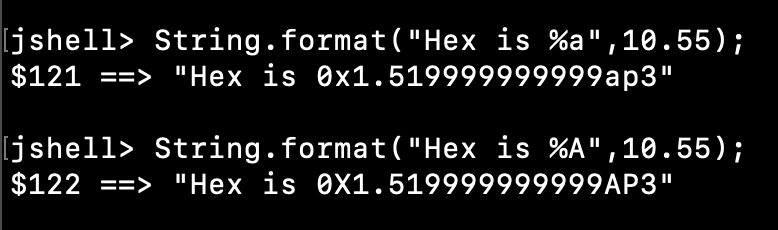
2. Formatting to Boolean Value (%b, %B)
If the argument is “false” or “null” then the result is “false”, otherwise the result is “true”.
jshell> String.format("value is %b",true);
$72 ==> "value is true"
jshell> String.format("value is %b",false);
$73 ==> "value is false"
jshell> String.format("value is %b",123);
$74 ==> "value is true"
jshell> String.format("value is %b","abc");
$76 ==> "value is true"
jshell> String.format("value is %B",true);
$77 ==> "value is TRUE"
jshell> String.format("value is %B",false);
$78 ==> "value is FALSE"
3. Character Formatting (%c, %C)
jshell> String.format("Unicode is %C",'T');
$79 ==> "Unicode is T"
jshell> String.format("Unicode is %c",'T');
$80 ==> "Unicode is T"
jshell> String.format("Unicode is %c",'\u0065');
$81 ==> "Unicode is e"
jshell> String.format("Unicode is %C",'\u0065');
$82 ==> "Unicode is E"
4. Decimal Integer Formatting (%d)
jshell> String.format("Number is %d", 10)
$83 ==> "Number is 10"
jshell> String.format("Number is %d", 0xFF)
$84 ==> "Number is 255"
jshell> String.format("Number is %d", 0b111)
$85 ==> "Number is 7"
5. Floating Point in Scientific Notation (%e, %E)
jshell> String.format("Number is %e", 10.55)
$86 ==> "Number is 1.055000e+01"
jshell> String.format("Number is %E", 10.55)
$87 ==> "Number is 1.055000E+01"
6. Floating Point in Decimal (%f)
jshell> String.format("Number is %f", 10.55)
$89 ==> "Number is 10.550000"
7. Object Hash Code Formatting (%h, %H)
The result is the hashCode() value of the object in the hexadecimal format.
jshell> String.format("Hash Code is %h", 10)
$90 ==> "Hash Code is a"
jshell> String.format("Hash Code is %h", "Java")
$92 ==> "Hash Code is 231e42"
jshell> String.format("Hash Code is %H", "Java")
$95 ==> "Hash Code is 231E42"
jshell> "Java".hashCode()
$94 ==> 2301506
“2301506” in the hexadecimal format is “231e42”.
8. Inserting New Line in the Formatting (%n)
jshell> String.format("Hello %s.%nHow are you?","Pankaj")
$96 ==> "Hello Pankaj.\nHow are you?"
9. Number in Octal and Hexadecimal Format (%o, %x, %X)
jshell> String.format("Number is %o", 10)
$97 ==> "Number is 12"
jshell> String.format("Number is %x", 15)
$99 ==> "Number is f"
jshell> String.format("Number is %X", 15)
$100 ==> "Number is F"
10. Object toString() Formatting (%s)
jshell> String.format("My name is %s","Pankaj")
$101 ==> "My name is Pankaj"
jshell> String.format("Exception is %s",new Exception("Error Message"))
$102 ==> "Exception is java.lang.Exception: Error Message"
jshell> String.format("Exception is %s",new Object())
$103 ==> "Exception is java.lang.Object@64c64813"
11. Date and Time Formatting (%t)
Date and Time formatting has some additional conversion specifiers. Some of them are:
- T: time
- A: Full name of the day of the week
- a: Short name of the day of the week
- B: Full name of the month
- b: Short name of the month
- C: Last two digits of the year, starting from 00 to 99
- c: Date and time formatted as “%ta %tb %td %tT %tZ %tY”
- D: Date formatted as “%tm/%td/%ty”, like “07/15/19”
You can get complete details of the conversion specifiers from the Formatter Class API Documentation.
jshell> String.format("%tT",Calendar.getInstance())
$105 ==> "14:55:43"
jshell> String.format("%tA",Calendar.getInstance())
$107 ==> "Monday"
jshell> String.format("%tc",Calendar.getInstance())
$108 ==> "Mon Jul 15 15:04:16 IST 2019"
12. Specifying Formatting Width
We can prefix an integer with the conversion specifier to specify the width of the result.
jshell> String.format("{%10d}", 2019);
$110 ==> "{ 2019}"
jshell> String.format("{%20s}", "Hello");
$111 ==> "{ Hello}"
13. Specifying Precision for the Formatting
We can specify the maximum number of the characters to be returned in the result by specifying the precision. It works only with numbers and strings.
jshell> String.format("%.5s", "Hello Format")
$113 ==> "Hello"
jshell> String.format("%.2f", 123.4567)
$114 ==> "123.46"
14. Specifying Argument Index
We can specify the argument index to be used for the formatting. The index is written between % and $ sign. The index starts at 1.
jshell> String.format("{%3$s %2$s %1$s}", "a","b","c")
$115 ==> "{c b a}"
15. Left and Right Aligned Formatting
jshell> String.format ("{%4s}", "a")
$116 ==> "{ a}"
jshell> String.format ("{%-4s}", "a")
$117 ==> "{a }"
16. Padding with 0s
jshell> String.format ("{%05d}", 99);
$118 ==> "{00099}"
17. Locale-Specific Formatting Examples
jshell> String.format(Locale.US, "%,d", 11111111)
$119 ==> "11,111,111"
jshell> String.format(Locale.FRENCH, "%,d", 11111111)
$120 ==> "11 111 111"
Conclusion
Java String format() method is very powerful. It provides a lot of conversion specifiers to help us in converting data from one type to another. It saves a lot of time if we use it properly and not rely on doing the conversion yourself.