Java integer to string conversion is a very simple task. There are various ways to convert an integer to string in Java.
- Convert using Integer.toString(int i)
- Convert using String.valueOf(int i)
- Convert using Integer constructor, deprecated in Java 9.
- Convert using String.format() method
- Convert using String concatenation
- Convert using StringBuffer and StringBuilder classes
- Convert using DecimalFormat class
- Converting Integer to different radix strings using Integer class
Table of Contents
Java Integer to String Conversion Examples
Let’s look into all the above methods examples to convert Java integer to a String object.
1. Integer.toString(int i)
This method converts the integer to the signed decimal string represenation. The int argument may be in any format, it’s first converted to a decimal value and then returned. The sign of the integer argument is maintained in the result string.
jshell> String str = Integer.toString(10);
str ==> "10"
jshell> String str = Integer.toString(-10);
str ==> "-10"
jshell> String str = Integer.toString(0xFF);
str ==> "255"
jshell> String str = Integer.toString(0b111);
str ==> "7"
jshell> String str = Integer.toString(077);
str ==> "63"
2. String.valueOf(int i)
String valueOf(int i) method returns the string representation of the integer. This method internally calls the Integer.toString(int i) method.
jshell> String str = String.valueOf(12);
str ==> "12"
jshell> String str = String.valueOf(-12);
str ==> "-12"
jshell> String str = String.valueOf(0b1111);
str ==> "15"
3. Using Integer Constructor and toString() Method
We can create the Integer object from the int value and then call its toString() method to convert int to string. This method has been deprecated in Java 9. So it’s good to know this method but you shouldn’t use it in your program.
jshell> String str = new Integer(10).toString();
str ==> "10"
4. String.format() Method
We can use the String format() method to convert an integer to string object.
jshell> String s1 = String.format("%d", 100);
s1 ==> "100"
jshell> String s1 = String.format("%d", 0xFF);
s1 ==> "255"
We can use this method for padding extra 0s in the left part of the output string if you want the string to have a specific length.
jshell> String.format("%05d", 100);
$66 ==> "00100"
jshell> String.format("%05d", 44);
$67 ==> "00044"
jshell> String.format("%05d", -1);
$68 ==> "-0001"
5. Using String Concatenation to convert Java Integer to String
We can concatenate an empty string with the int value to convert it to a string object.
jshell> int i = 10;
i ==> 10
jshell> Integer io = Integer.valueOf(100);
io ==> 100
jshell> String str = "" + i;
str ==> "10"
jshell> String str = "" + io;
str ==> "100"
6. Using StringBuffer and StringBuilder classes
We can append the integer to empty StringBuilder/StringBuffer object. Then call the toString() method to get the string representation.
jshell> String s1 = new StringBuffer().append(20).toString();
s1 ==> "20"
jshell> String s1 = new StringBuilder().append(20).toString();
s1 ==> "20"
7. Convert Java integer to String using DecimalFormat class
DecimalFormat class is used to format decimal numbers. We can use its format() method to convert an integer to string object.
jshell> import java.text.DecimalFormat;
jshell> DecimalFormat decimalFormat = new DecimalFormat("#");
decimalFormat ==> java.text.DecimalFormat@674dc
jshell> String s1 = decimalFormat.format(1234);
s1 ==> "1234"
Converting Integer to String with Radix
Integer class provides some utility methods to convert an integer to string representation in different radix values. The prefixes used to denote integer base is not part of the output string.
- toBinaryString(int i)
- toOctalString(int i)
- toHexString(int i)
- toString(int i, int radix)
Let’s look into some examples of using these methods to convert an integer to string in a different base.
package net.javastring.strings;
public class JavaIntegerToString {
public static void main(String[] args) {
String strBinary = Integer.toBinaryString(10);
System.out.println(strBinary);
String strOctal = Integer.toOctalString(10);
System.out.println(strOctal);
String strHex = Integer.toHexString(10);
System.out.println(strHex);
String str7Radix = Integer.toString(10, 7);
System.out.println(str7Radix);
}
}
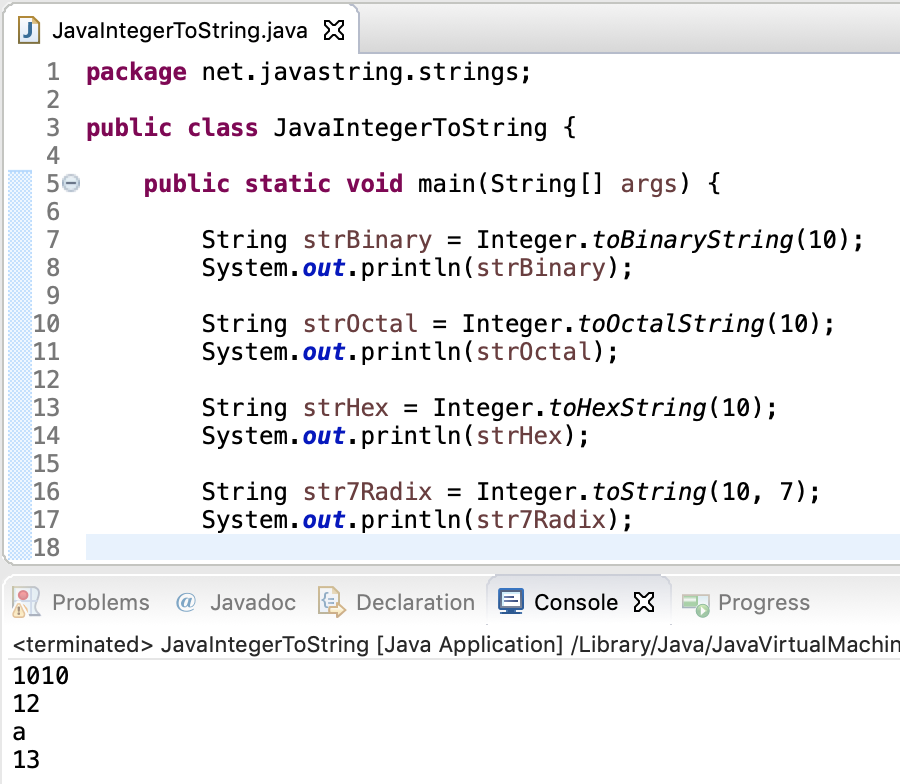
Notice that the output string doesn’t have the prefixes that define the integer radix.
Best way to Convert Integer to String
There are many ways to convert an integer to string object. However, the recommended way is to use Integer.toString(int i) method. This method gets called by String.valueOf(int i) method too.