- Java String replace() method is used to replace part of the string and create a new string object.
- Since the string is immutable, the original string remains the same.
- The replace() method returns a new string and we have to assign it to a variable to use it.
- There are two overloaded replace() methods in the String class.
Table of Contents
Java String replace() Methods
There are two variants of replace() method in the String class.
- replace(char oldChar, char newChar): replaces all the occurrences of the oldChar with the newChar and returns a new string. If the string doesn’t contain oldChar character, then the reference of this string is returned.
- replace(CharSequence target, CharSequence replacement): replaces all the occurrences of the target with the replacement and return a new string. Since the method argument is CharSequence, we can pass String, StringBuilder, and StringBuffer objects as an argument. The replacement is performed from the start of the string to the end. This utility method was added in Java 1.5 release.
String replace() Method Examples
Let’s look at some examples of replace() method.
1. Replacing a Character in the String
Let’s look at a simple example to replace all the occurrences of a character with another character.
jshell> String str = "Hello World";
str ==> "Hello World"
jshell> String newString = str.replace('o', 'O');
newString ==> "HellO WOrld"
We can also pass the Unicode value of the character. The Unicode code of ‘o’ is ‘\u006F’ and the Unicode code of ‘O’ is ‘\u004F’.
jshell> String newString = str.replace('\u006F', '\u004F');
newString ==> "HellO WOrld"
If the replace() method doesn’t replace anything in the source string, the reference of the source string is returned. Let’s test this with the equality operator.
jshell> String str = "Hello World";
str ==> "Hello World"
jshell> String newString = str.replace('x', 'y')
newString ==> "Hello World"
jshell> str == newString
$73 ==> true
2. Replacing a Substring with another Substring
We can use the replace() method to replace a substring with another substring. Let’s look at a simple example.
jshell> String str = "Hello World";
str ==> "Hello World"
jshell> String newString = str.replace("World", "Java");
newString ==> "Hello Java"
The replacement is always performed from the start to the end. The substring is replaced one by one and the final result string is returned. Let’s understand why this is an important point.
jshell> String str = "aaaaa";
str ==> "aaaaa"
jshell> String newString = str.replace("aa", "bb");
newString ==> "bbbba"
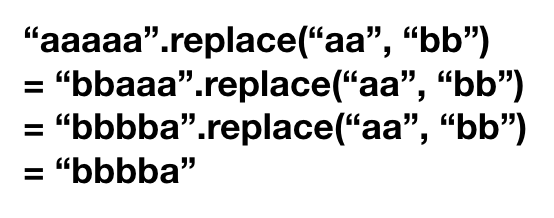
3. Using StringBuffer and StringBuilder in replace() Method
The popular implementations of the CharSequence
interface are:
- String
- StringBuffer
- StringBuilder
We have already seen the example of using String in the replace() method. Let’s look at an example where we are using StringBuffer and StringBuilder objects in the replace() method.
jshell> String str = "Hello World";
str ==> "Hello World"
jshell> StringBuffer target = new StringBuffer("World");
target ==> World
jshell> StringBuilder replacement = new StringBuilder("Java");
replacement ==> Java
jshell> String newString1 = str.replace(target, replacement);
newString1 ==> "Hello Java"
If you look at the replace() method implementation, the toString() method is called on the arguments to convert them to string.
4. When replace() target is an empty string
Let’s see what happens when the target substring is an empty string.
jshell> String str = "123"
str ==> "123"
jshell> str.replace("","A")
$86 ==> "A1A2A3A"
So the replacement string is added before and after each character in the string. Also, the target string is added at the start and the end of the string.
5. Using replace() Method to Remove Character or Substring
We can also use replace() method to remove a character or a substring. The trick is to pass replacement as an empty string. Note that we can’t use the first replace() method that accepts characters because there is no such thing as an empty character.
jshell> String str = "Hello World 2019";
str ==> "Hello World 2019"
jshell> str.replace(" ", "");
$89 ==> "HelloWorld2019"
jshell> str.replace(" World", "");
$90 ==> "Hello 2019"
jshell> str.replace("o", "");
$91 ==> "Hell Wrld 2019"
6. Using replace() Method to Change CSV Delimiter
Let’s say we have CSV data where the delimiter is a comma. For some reason, we want to change the delimiter to the pipe character. We can easily do this using the replace() method.
jshell> String csvData = "Apple,Banana,Orange";
csvData ==> "Apple,Banana,Orange"
jshell> String csvWithPipeDelimiter = csvData.replace(",", "|");
csvWithPipeDelimiter ==> "Apple|Banana|Orange"
Conclusion
Java String replace() method is useful in replacing part of a string and create a new string. It’s a utility method and you can use it to remove a character or a substring from the string.