The byte is a primitive type in Java. In this tutorial, we will learn how to convert String to byte array. We will also learn how to convert byte array to string.
Table of Contents
Java String to Byte Array
We can convert String to byte array using getBytes() method. This method encodes the string into a sequence of bytes using the platform’s default charset. Let’s look at a simple example of getBytes() method.
jshell> String s1 = "Java";
s1 ==> "Java"
jshell> byte[] byteArray = s1.getBytes();
byteArray ==> byte[4] { 74, 97, 118, 97 }
Overloaded getBytes() Methods
String getBytes() method is overloaded. There are two more variations of this method.
public byte[] getBytes(Charset charset)
: This method encodes the string to the byte array using the given Charset.public byte[] getBytes(String charsetName)
: It’s similar to the above method. Here the Charset name is provided as a string argument. If the charset is not supported,UnsupportedEncodingException
is thrown.
Let’s look at some examples of using these two getBytes() methods.
jshell> import java.nio.charset.StandardCharsets;
jshell> String s1 = "Java";
s1 ==> "Java"
jshell> s1.getBytes(StandardCharsets.UTF_16);
$56 ==> byte[10] { -2, -1, 0, 74, 0, 97, 0, 118, 0, 97 }
jshell> s1.getBytes(StandardCharsets.UTF_8);
$57 ==> byte[4] { 74, 97, 118, 97 }
jshell> s1.getBytes("UTF-8");
$58 ==> byte[4] { 74, 97, 118, 97 }
jshell> s1.getBytes("UTF-16");
$59 ==> byte[10] { -2, -1, 0, 74, 0, 97, 0, 118, 0, 97 }
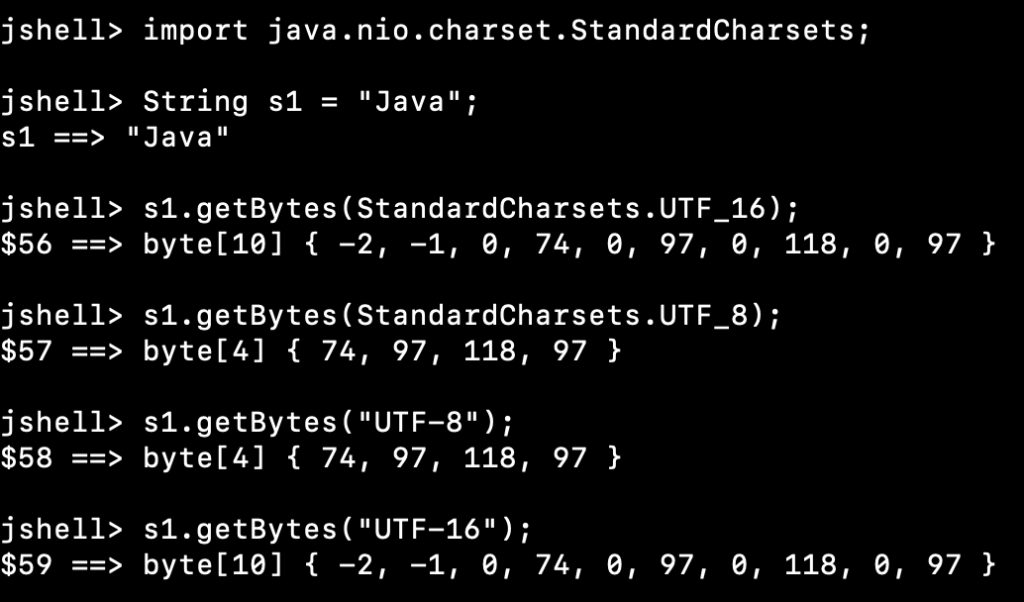
Java Byte Array to String
String class has a constructor that takes byte array argument. Let’s see how to create a string from the byte array.
jshell> byte[] ba = {74, 97, 118, 97};
ba ==> byte[4] { 74, 97, 118, 97 }
jshell> String s2 = new String(ba);
s2 ==> "Java"
Java byte to String
We can use Byte class toString() method to convert byte to string representation.
jshell> byte b = 67;
b ==> 67
jshell> Byte.toString(b);
$61 ==> "67"
What if we want to treat the byte value as code point and then convert it to the string?
We can do this using Character.toString() method.
jshell> byte b = 67;
b ==> 67
jshell> String.valueOf(Character.toString(b));
$63 ==> "C"
What if the String has special characters?
We know that byte value ranges from -128 to 127. So what happens when our string contains special characters.
Let’s see if string to byte array and then byte array to string works fine or not.
package net.javastring.strings;
import java.nio.charset.StandardCharsets;
import java.util.Arrays;
public class JavaStringFromByteArray {
public static void main(String[] args) {
String str = "©";
byte[] bytes = str.getBytes();
System.out.println(Arrays.toString(bytes));
String str1 = new String(bytes);
System.out.println(str1);
byte[] bytes1 = str.getBytes(StandardCharsets.ISO_8859_1);
System.out.println(Arrays.toString(bytes1));
String str2 = new String(bytes1);
System.out.println(str2);
}
}
Output:
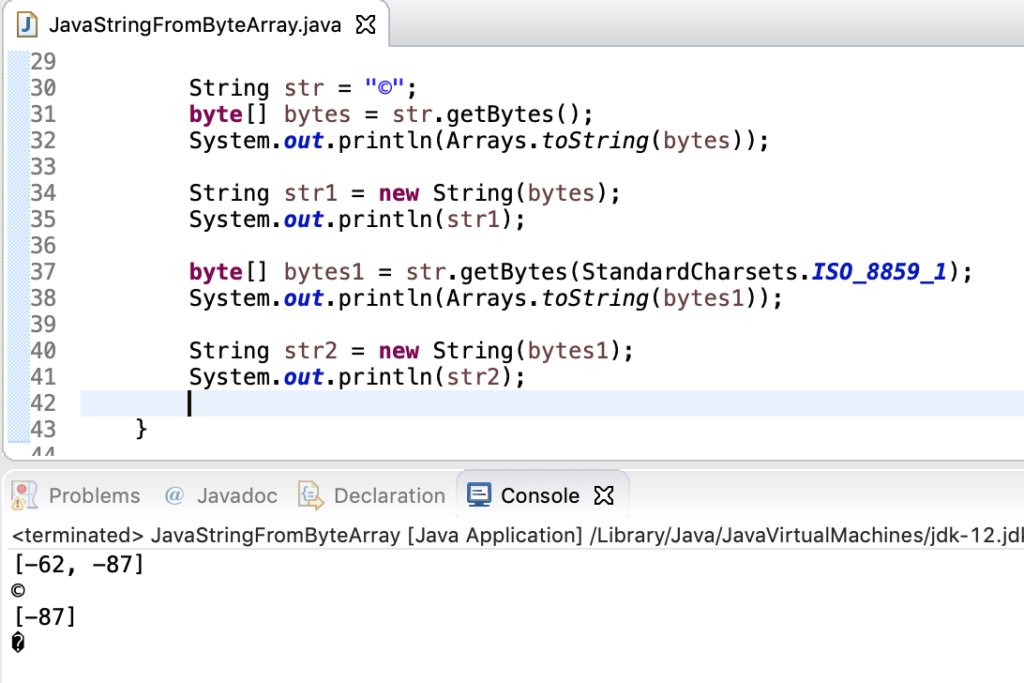
It’s clear from the output that the string to byte array conversion works fine with the default Charset. But, with the ISO_8859_1 Charset the output is not correct. So the reverse conversion also fails.
It’s important to use the proper Charset for conversion of string to byte array. Otherwise, you may get improper results.
How to Find the System Default Charset?
According to the Charset documentation:
The default charset is determined during virtual-machine startup and typically depends upon the locale and charset being used by the underlying operating system.
https://docs.oracle.com/javase/7/docs/api/java/nio/charset/Charset.html
We can find the system default Charset using its defaultCharset() method.
jshell> import java.nio.charset.Charset;
jshell> Charset.defaultCharset();
$65 ==> UTF-8
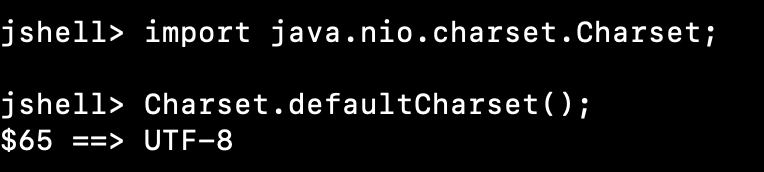
Conclusion
- We can use String getBytes() method to convert string to byte array.
- It’s important to know the string contents, so that we can specify correct Charset for encoding.
- We can convert byte to string using Byte.toString() method.
- We can use Character.toString() method to treat byte value as code point and convert to corresponding character.
- The JVM default Charset depends on the operating system and locale. We can get this information using Charset.defaultCharset() method.