- Java String lines() method returns the stream of lines from the string.
- The lines are separated by line terminators – \n, \r, and \r\n.
- A line is considered as a sequence of characters followed by a line terminator or end of the string.
- The line doesn’t include line terminators.
- The stream contains the lines in which they occur in the string from start to end.
- If the string is empty, zero lines are returned.
- This method was added to String API in Java 11 release.
- Java String lines() method is better than the split() method because the elements are supplied lazily and the searching of line terminators is faster.
Table of Contents
Java String lines() Examples
Let’s look at some examples of using String lines() method.
1. String lines to List
We can use lines() method to get the stream of lines. Then use the forEach() method to add all these lines to a list.
package net.javastring.strings;
import java.util.ArrayList;
import java.util.List;
public class JavaStringLines {
public static void main(String[] args) {
String str = "\nHi\nHello\nYes\r\nNo\n";
List<String> lines = new ArrayList<>();
str.lines().forEach(s -> lines.add(s));
System.out.println(lines);
}
}
Output: [, Hi, Hello, Yes, No]
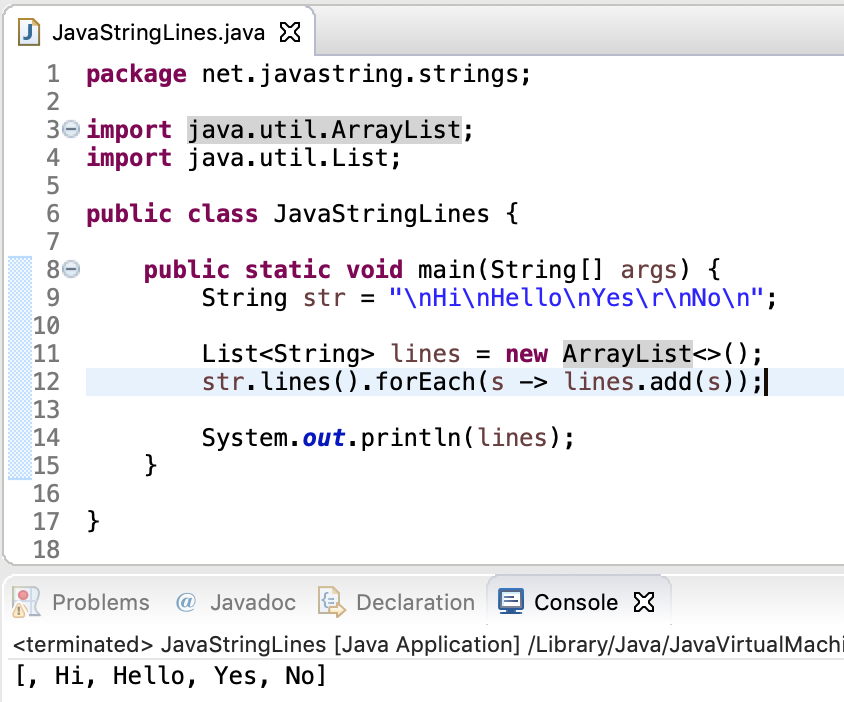
The output is more clear if we write the string object like this:
String str = "\n"
+ "Hi\n"
+ "Hello\n"
+ "Yes\r\n"
+ "No\n";
2. Count the Lines in a String
We can count the number of lines in a string using the lines() method followed by the Stream count() method.
jshell> String str = "\n" + "Hi\n" + "Hello\n" + "Yes\r\n" + "No\n";
str ==> "\nHi\nHello\nYes\r\nNo\n"
jshell> long count = str.lines().count();
count ==> 5

3. Print all the Lines in a String
We can print the lines in a string using the lines() method. It’s better than splitting and then printing the lines because it saves time and memory.
jshell> String str = "\nHi\nHello\nYes\r\nNo\n";
str ==> "\nHi\nHello\nYes\r\nNo\n"
jshell> str.lines().forEach(System.out::println);
Hi
Hello
Yes
No
jshell>
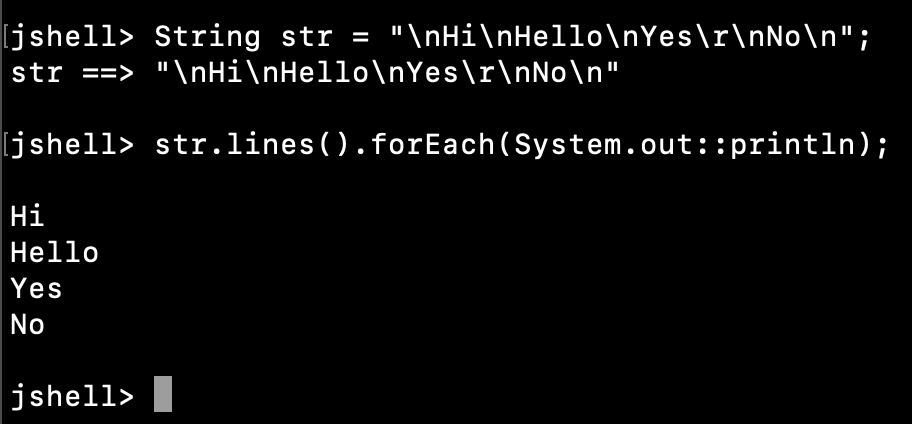
4. String Lines to Array
We can use toArray() method of Stream API to convert string lines into an array. Note that the output will be an Object array and not String array.
package net.javastring.strings;
import java.util.Arrays;
public class JavaStringLines {
public static void main(String[] args) {
String str = "Java\nPython\rC\r\nAndroid";
Object[] lines = str.lines().toArray();
System.out.println(Arrays.toString(lines));
}
}
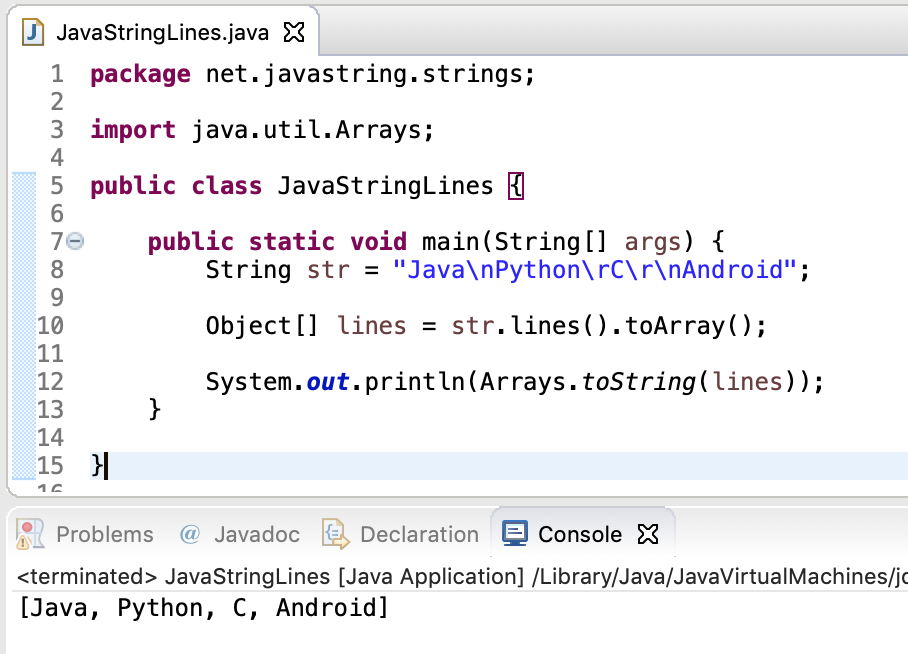
Conclusion
Java String lines() provides a stream of lines. We can use it to convert the string lines to list and array. It’s much better in performance than the split() method to get the string lines because of Stream API lazy initializations.